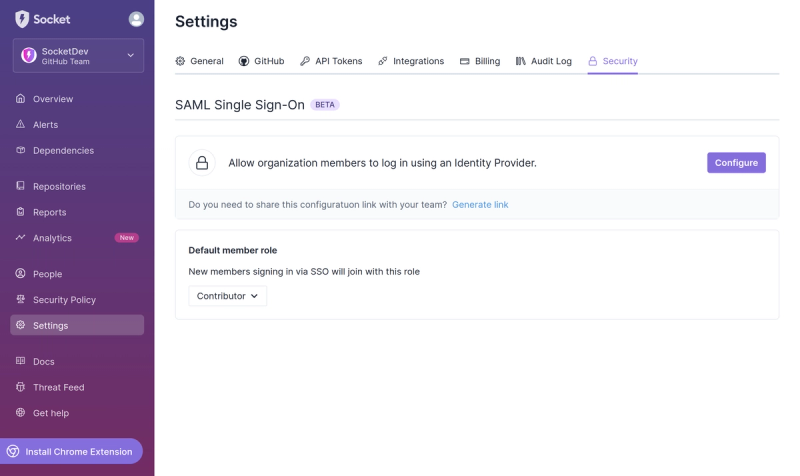
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
roachjs
Advanced tools
Readme
This client is a port from the original Golang client. Internally it's is more or less the same, but this driver provides a friendlier javascript interface.
$ npm install roachjs
var Roach = require('roachjs')
var client = new Roach({
uri: 'https://localhost:8080'
})
module.exports = client
client.get("sample_key", function(err, value, res) {
if(err) throw err
client.put("other_key", value, function(err, res) {
if(err) {
// Failed
}
else {
// Sucess
}
})
})
// You should prepare your queries and send them in a single batch
// For optimal performance
var c = client.prepare()
// This callback will be the first to be executed
c.get("sample_key", function(err, value, res) {
if(err) throw err
// Do something...
})
c.get("sample_key2", function(err, value, res) {
if(err) throw err
// Do something...
})
c.put("some_key", "some_value", function(err) {
if(err) throw err
// Do something
})
// The flush callback is the last one to be called
c.flush(function(err, res) {
if(err) throw err
console.log('Sucessfuly flushed %d queries.', res.responses.length)
})
var opts = {
name: "transaction example",
}
var errNoApples = new Error('Insufficient apples!')
var transaction = function(txn, commit, abort) {
txn.get("applesInStock", function(err, value, res) {
if(err || applesInStock.err) {
return abort(err)
}
var dispatch = 5
var inStock = parseInt(applesInStock.value)
if(inStock < dispatch) {
return abort(errNoApples)
}
// Upgrade for a prepared client
txn = txn.prepare()
txn.increment("applesInStock", -dispatch)
txn.increment("applesInRoute", +dispatch)
// Commit automatically flushes
commit()
})
}
client.runTransaction(opts, transaction, function(err, res) {
if(err === errNoApples) {
// Alert user there are no more apples...
}
else if(err) {
// Transaction failed...
}
else {
// Transaction commited...
}
})
Returns a new roachjs client with options.
name | type | description |
---|---|---|
opts | object | see |
opt | description | default |
---|---|---|
uri | uri to the cockroach http endpoint | https://localhost:8080/ |
host | host or ip to the cockroach http endpoint | localhost |
port | port to the cockroach http endpoint | 8080 |
ssl | connect throught https | true |
user | user to run the requests with | root |
retry | retry requests when cockroach responds with a busy signal | true |
http | http module to use | require('https') |
agent | http agent to use on the requests (read more) | new http.Agent() |
clock | clock module to use (read more) | internal clock module |
method |
---|
get |
put |
conditionalPut |
contains |
increment |
scan |
delete |
deleteRange |
prepare |
runTransaction |
Gets a single entry from the datastore, specified by key
.
name | type | description |
---|---|---|
key | string | |
callback | callback | function(err, value, res) {} |
name | type | description |
---|---|---|
err | Error() | |
value | Buffer | |
res | object | see |
client.get("key", function(err, value, res) {})
Puts a value in the datastore in the specified key
. Ideally you
should send in buffers, but you can pass a string, preferably an utf-8 encoded string.
name | type | description |
---|---|---|
key | string | |
value | Buffer, string | |
callback | callback | function(err, res) {} |
name | type | description |
---|---|---|
err | Error() | |
res | object | see |
client.put("key", "value", function(err, res) {})
ConditionalPut sets the value
for a key
if the existing value matches the ifValue
.
Specifying an empty or null ifValue
means the entry must not yet exist.
name | type | description |
---|---|---|
key | string | |
value | Buffer, string | |
ifValue | Buffer, string, null | use null to put if entry doens't exists |
callback | callback | function(err, res) {} |
name | type | description |
---|---|---|
err | Error() | |
actualValue | Buffer | If conditional put fails this value is set |
res | object | see |
client.conditionalPut("status", "running", "stopped", function(err, actualValue, res) {})
client.conditionalPut("status", "new", null, function(err, actualValue, res) {})
Contains determines if a key
exists in the datastore.
name | type | description |
---|---|---|
key | string | |
callback | callback | function(err, exists, res) {} |
name | type | description |
---|---|---|
err | Error() | |
exists | boolean | |
res | object | see |
client.contains("john", function(err, exists, res) {
if(exists === true) {
// john exists in the datastore
}
})
Increment increments the value at the specified key
by some increment
value.
Once called for a key
, Put & Get will return errors; only Increment will continue to be a valid command.
The value must be deleted before it can be reset using Put.
name | type | description |
---|---|---|
key | string | |
increment | integer | |
callback | callback | function(err, newValue, res) {} |
name | type | description |
---|---|---|
err | Error() | |
newValue | integer | the new value for this counter, after the increment operation |
res | object | see |
client.increment("counter", 5, function(err, newValue, res) {
console.log('counter current value is', newValue)
})
Scan the datastore for keys in the range of the start_key
and end_key
, limiting the result by limit
.
name | type | description |
---|---|---|
key | string | |
start_key | string | |
end_key | string | |
limit | integer | |
callback | callback | function(err, rows, res) {} |
name | type | description |
---|---|---|
err | Error() | |
rows | array | |
res | object | see |
client.scan("a", "Z", 100, function(err, rows, res) {
for(row as rows) {
console.log(row)
}
})
Delete an entry from the datastore specified by key
.
name | type | description |
---|---|---|
key | string | |
callback | callback | function(err, res) {} |
name | type | description |
---|---|---|
err | Error() | |
res | object | see |
client.delete("key", function(err, res) {})
Delete all keys found in a range, from start_key
to end_key
, limited by limit
.
name | type | description |
---|---|---|
key | string | |
start_key | string | |
end_key | string | |
limit | integer | |
callback | callback | function(err, deleted, res) {} |
name | type | description |
---|---|---|
err | Error() | |
deleted | integer | number of entries deleted |
res | object | see |
client.deleteRange("a", "Z", 100, function(err, deleted, res) {
console.log('deleted %d entries', deleted)
})
Return you a new prepared client. It has all the methods from the original client. Read Advanced client usage II to understand how to use this client. You should always use this client when sending in multiple queries, this will batch them together in a single request.
method | description |
---|---|
flush | Flush the prepared queries |
var c = client.prepare()
c.get("key", function(err, value, res) {
// Do something...
})
c.get("key2", function(err, value, res) {
// Do something...
})
c.put("key3", "value", function(err, res) {
// Do something...
})
c.flush()
Flush the prepared queries buffer, and send it as a batch request.
name | type | description |
---|---|---|
callback | callback | optional |
name | type | description |
---|---|---|
err | Error() | batch request failed |
res | object | see |
client.flush(function(err, res) {
if(err) {
// Flush failed..
}
else {
console.log('flushed %d queries.', res.responses.length)
}
})
Returns an response object.
property | type | description |
---|---|---|
err | Error() | is null if no error was returned |
value | string, number, boolean | general response value |
res | object | see |
RunTransaction executes a retryable transaction
function in
the context of a distributed transaction. The transaction is
automatically aborted if retryable function returns any error aside from
recoverable internal errors, and is automatically committed otherwise.
retryable should have no side effects which could cause problems in the event
it must be run more than once. The opts
contains transaction settings.
name | type | description |
---|---|---|
opts | object | options |
transacation | retryable function | function(txn, commit, abort) {} |
callback | callback | function(err, res) {} |
opt | description | default |
---|---|---|
name | transaction name for debugging | "" |
isolation | 0 |
name | type | description |
---|---|---|
err | Error() | if transaction fails |
res | object | see |
The res
argument contains the full database response, each database command can
contain a different set of properties. This document will try to state some of the possible properties.
property | type | description |
---|---|---|
timestamp | integer | timestamp of the returned entry |
wall_time | integer | timestamp of when the read or write operation was performed |
The transaction function is an retryable function, it may be
executed more than once. This function should never forget to
call commit
or abort
. Throwing an error inside this
function also aborts the transaction.
name | type | description |
---|---|---|
txn | Prepared client | this client is the same as client.prepare() , you can flush yourself if you don't wan't to commit yet. |
commit | callback | to try to commit transaction |
abort | callback | to abort transaction |
abort()
accepts an optional Error
. This error will be passed to the
.runTransaction callback.var transaction = function(txn, commit, abort) {
txn = txn.prepare()
for(var i = 0; i < 100; i++) {
var key = i.toString()
txn.put(key, "hello")
}
// Commit automatically flushes
// the prepared transaction.
commit()
}
Cockroachdb's protocol buffer files are mantained at a repository called cockroachdb/cockroach-proto, this is
maintained as a subtree
in this library, in case you need to manually update or change them, follow this steps.
You will need to update the folder cockroach-proto with the latest content of the cockroachdb/cockroach-proto repository, you could do this with:
$ git subtree pull -P cockroach-proto git@github.com:cockroachdb/cockroach-proto.git master --squash
Run the following npm script to compile the .proto files to javascript, it will automatically place the files in the lib folder.
$ npm run build-proto
You may wan't to use a custom clock module in some cases, you can pass it through the clock
option, when instantiating a new Client
.
Your clock module should have a now
method, and this method should return the current timestamp in nanoseconds, here is an example:
var clockModule = module.exports = {
now: function TimestampNanoseconds() {
// Should return the current timestamp in nanoseconds
return ...;
}
}
var client = new Client({
clock: clockModule
})
FAQs
Node.js driver for cockroachdb.
The npm package roachjs receives a total of 45 weekly downloads. As such, roachjs popularity was classified as not popular.
We found that roachjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.