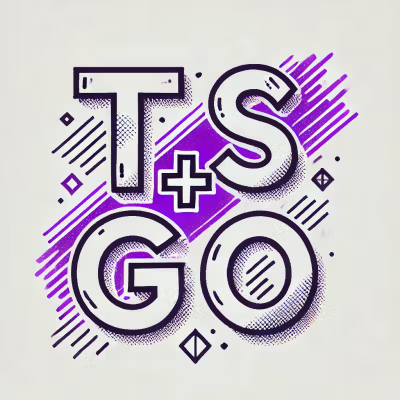
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
rockets-client
Advanced tools
> A small client for [Rockets](../README.md) using [JSON RPC](https://www.jsonrpc.org) as communication contract over a [WebSocket](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket).
A small client for Rockets using JSON RPC as communication contract over a WebSocket.
You can install this package from NPM:
npm add rxjs rockets-client
Or with Yarn:
yarn add rxjs rockets-client
For CDN, you can use unpkg:
https://unpkg.com/rockets-client/dist/bundles/rockets-client.umd.min.js
The global namespace for rockets is rocketsClient
:
const {Client} = rocketsClient;
const rockets = new Client({
url: 'myhost'
});
Create a client and connect:
import {Client} from 'rockets-client';
const rockets = new Client({
url: 'myhost',
onConnected() {
console.info('I just connected');
},
onClosed(evt) {
console.log(`Socket connection closed with code ${evt.code}`);
}
});
Close the connection with the socket cleanly:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
rockets.subscribe({
next(notification) {
console.log(notification);
},
complete() {
console.log('Socket connection closed');
}
});
rockets.disconnect();
Listen to server notifications:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
rockets.subscribe(notification => {
console.log(notification);
});
Send notifications:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
rockets.notify('mymethod', {
ping: true
});
Send a notification using the Notification
object:
import {Client, Notification} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const notification = new Notification('mymethod', {
ping: true
});
rockets.notify(notification);
Make a request:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const response = await rockets.request('mymethod', {
ping: true
});
console.log(response);
NOTE: There is no need to wait for a connection to be established with the socket as requests will be buffered and sent once the connection is alive. In case of a socket error, the request promise will reject. The same is true for batch requests and notifications.
Or make a request using the Request
object:
import {Client, Request} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const request = new Request('mymethod', {
ping: true
});
const response = await rockets.request(request);
console.log(response);
Handle a request error:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
try {
await rockets.request('mymethod');
} catch (err) {
console.log(err.code);
console.log(err.message);
console.log(err.data);
}
NOTE: Any error that may occur will be a JsonRpcError
.
Cancel a request:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const task = rockets.request('mymethod');
task.cancel();
Get progress updates for a request:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const task = rockets.request('mymethod');
task.on('progress')
.subscribe(progress => {
console.log(progress);
});
Make a batch request:
import {Client, Notification, Request} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const request = new Request('mymethod');
const notification = new Notification('mymethod');
const [response] = await rockets.batch(...[
request,
notification
]);
const result = await response.json();
console.log(result);
NOTE: Notifications will not return any responses and if no requests are sent, the batch request will resolve without any arguments.
Handle a batch request error:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
try {
const request = new Request('mymethod');
await rockets.batch(request);
} catch (err) {
console.log(err.code);
console.log(err.message);
console.log(err.data);
}
NOTE: The batch promise will not reject for response errors, but you can catch the response error when using the response .json()
method:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
try {
const request = new Request('mymethod');
const [response] = await rockets.batch(request);
await response.json();
} catch (err) {
console.log(err.code);
console.log(err.message);
console.log(err.data);
}
Cancel a batch request:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const request = new Request('mymethod');
const task = rockets.batch(request);
task.cancel();
NOTE: Notifications cannot be canceled.
Get progress updates for a batch request:
import {Client} from 'rockets-client';
const rockets = new Client({url: 'myhost'});
const request = new Request('mymethod');
const task = rockets.batch(request);
task.on('progress')
.subscribe(progress => {
console.log(progress);
});
If you're a contributor and wish to make a release of this package use:
# Cut a minor release
# A release can be: patch, minor, major;
yarn release --release-as minor
See release as a target type imperatively like npm-version for more release options.
After the changes land in master, the new version will be automatically published by the CI.
IMPORTANT: Follow the semver for versioning.
FAQs
> A small client for [Rockets](../README.md) using [JSON RPC](https://www.jsonrpc.org) as communication contract over a [WebSocket](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket).
The npm package rockets-client receives a total of 9 weekly downloads. As such, rockets-client popularity was classified as not popular.
We found that rockets-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.