Comparing version 0.8.3 to 0.8.4
{ | ||
"name": "rusha", | ||
"version": "0.8.3", | ||
"version": "0.8.4", | ||
"description": "A high-performance pure-javascript SHA1 implementation suitable for large binary data.", | ||
@@ -33,3 +33,4 @@ "main": "rusha.js", | ||
"grunt-sweet.js": "~0.1.5", | ||
"microtime": "^1.0.1", | ||
"microtime": "^2.1.1", | ||
"mocha": "^3.0.2", | ||
"sweet.js": "~0.7.1" | ||
@@ -36,0 +37,0 @@ }, |
@@ -61,6 +61,6 @@ # Rusha [](https://travis-ci.org/srijs/rusha) | ||
If you want to check the performance for yourself in your own browser, I compiled a [JSPerf Page](http://jsperf.com/rusha/2). | ||
If you want to check the performance for yourself in your own browser, I compiled a [JSPerf Page](http://jsperf.com/rusha/13). | ||
A normalized estimation based on the best results for each implementation, smaller is better: | ||
 | ||
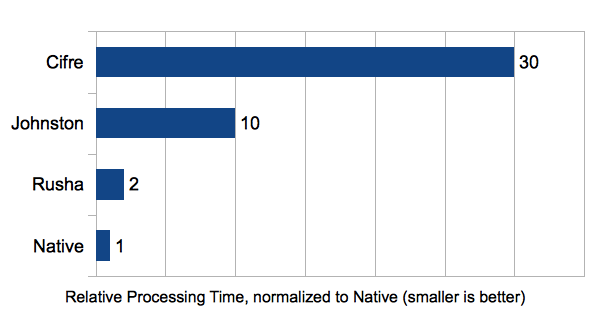 | ||
@@ -67,0 +67,0 @@ Results per Implementation and Platform: |
172
rusha.js
@@ -1,2 +0,3 @@ | ||
/* | ||
(function () { | ||
var /* | ||
* Rusha, a JavaScript implementation of the Secure Hash Algorithm, SHA-1, | ||
@@ -29,36 +30,32 @@ * as defined in FIPS PUB 180-1, tuned for high performance with large inputs. | ||
*/ | ||
(function () { | ||
var util = { | ||
getDataType: function (data) { | ||
if (typeof data === 'string') { | ||
return 'string'; | ||
} | ||
if (data instanceof Array) { | ||
return 'array'; | ||
} | ||
if (typeof global !== 'undefined' && global.Buffer && global.Buffer.isBuffer(data)) { | ||
return 'buffer'; | ||
} | ||
if (data instanceof ArrayBuffer) { | ||
return 'arraybuffer'; | ||
} | ||
if (data.buffer instanceof ArrayBuffer) { | ||
return 'view'; | ||
} | ||
if (data instanceof Blob) { | ||
return 'blob'; | ||
} | ||
throw new Error('Unsupported data type.'); | ||
util = { | ||
getDataType: function (data) { | ||
if (typeof data === 'string') { | ||
return 'string'; | ||
} | ||
}; | ||
// The Rusha object is a wrapper around the low-level RushaCore. | ||
// It provides means of converting different inputs to the | ||
// format accepted by RushaCore as well as other utility methods. | ||
if (data instanceof Array) { | ||
return 'array'; | ||
} | ||
if (typeof global !== 'undefined' && global.Buffer && global.Buffer.isBuffer(data)) { | ||
return 'buffer'; | ||
} | ||
if (data instanceof ArrayBuffer) { | ||
return 'arraybuffer'; | ||
} | ||
if (data.buffer instanceof ArrayBuffer) { | ||
return 'view'; | ||
} | ||
if (data instanceof Blob) { | ||
return 'blob'; | ||
} | ||
throw new Error('Unsupported data type.'); | ||
} | ||
}; | ||
function Rusha(chunkSize) { | ||
'use strict'; | ||
// Private object structure. | ||
var self$2 = { fill: 0 }; | ||
// Calculate the length of buffer that the sha1 routine uses | ||
var // Private object structure. | ||
self$2 = { fill: 0 }; | ||
var // Calculate the length of buffer that the sha1 routine uses | ||
// including the padding. | ||
var padlen = function (len) { | ||
padlen = function (len) { | ||
for (len += 9; len % 64 > 0; len += 1); | ||
@@ -68,14 +65,16 @@ return len; | ||
var padZeroes = function (bin, len) { | ||
for (var i = len >> 2; i < bin.length; i++) | ||
bin[i] = 0; | ||
for (var i$2 = len >> 2; i$2 < bin.length; i$2++) | ||
bin[i$2] = 0; | ||
}; | ||
var padData = function (bin, chunkLen, msgLen) { | ||
bin[chunkLen >> 2] |= 128 << 24 - (chunkLen % 4 << 3); | ||
bin[((chunkLen >> 2) + 2 & ~15) + 14] = msgLen >> 29; | ||
// To support msgLen >= 2 GiB, use a float division when computing the | ||
// high 32-bits of the big-endian message length in bits. | ||
bin[((chunkLen >> 2) + 2 & ~15) + 14] = msgLen / (1 << 29) | 0; | ||
bin[((chunkLen >> 2) + 2 & ~15) + 15] = msgLen << 3; | ||
}; | ||
// Convert a binary string and write it to the heap. | ||
var // Convert a binary string and write it to the heap. | ||
// A binary string is expected to only contain char codes < 256. | ||
var convStr = function (H8, H32, start, len, off) { | ||
var str = this, i, om = off % 4, lm = len % 4, j = len - lm; | ||
convStr = function (H8, H32, start, len, off) { | ||
var str = this, i$2, om = off % 4, lm = len % 4, j = len - lm; | ||
if (j > 0) { | ||
@@ -93,4 +92,4 @@ switch (om) { | ||
} | ||
for (i = om; i < j; i = i + 4 | 0) { | ||
H32[off + i >> 2] = str.charCodeAt(start + i) << 24 | str.charCodeAt(start + i + 1) << 16 | str.charCodeAt(start + i + 2) << 8 | str.charCodeAt(start + i + 3); | ||
for (i$2 = om; i$2 < j; i$2 = i$2 + 4 | 0) { | ||
H32[off + i$2 >> 2] = str.charCodeAt(start + i$2) << 24 | str.charCodeAt(start + i$2 + 1) << 16 | str.charCodeAt(start + i$2 + 2) << 8 | str.charCodeAt(start + i$2 + 3); | ||
} | ||
@@ -106,6 +105,6 @@ switch (lm) { | ||
}; | ||
// Convert a buffer or array and write it to the heap. | ||
var // Convert a buffer or array and write it to the heap. | ||
// The buffer or array is expected to only contain elements < 256. | ||
var convBuf = function (H8, H32, start, len, off) { | ||
var buf = this, i, om = off % 4, lm = len % 4, j = len - lm; | ||
convBuf = function (H8, H32, start, len, off) { | ||
var buf = this, i$2, om = off % 4, lm = len % 4, j = len - lm; | ||
if (j > 0) { | ||
@@ -123,4 +122,4 @@ switch (om) { | ||
} | ||
for (i = 4 - om; i < j; i = i += 4 | 0) { | ||
H32[off + i >> 2] = buf[start + i] << 24 | buf[start + i + 1] << 16 | buf[start + i + 2] << 8 | buf[start + i + 3]; | ||
for (i$2 = 4 - om; i$2 < j; i$2 = i$2 += 4 | 0) { | ||
H32[off + i$2 >> 2] = buf[start + i$2] << 24 | buf[start + i$2 + 1] << 16 | buf[start + i$2 + 2] << 8 | buf[start + i$2 + 3]; | ||
} | ||
@@ -137,3 +136,3 @@ switch (lm) { | ||
var convBlob = function (H8, H32, start, len, off) { | ||
var blob = this, i, om = off % 4, lm = len % 4, j = len - lm; | ||
var blob = this, i$2, om = off % 4, lm = len % 4, j = len - lm; | ||
var buf = new Uint8Array(reader.readAsArrayBuffer(blob.slice(start, start + len))); | ||
@@ -152,4 +151,4 @@ if (j > 0) { | ||
} | ||
for (i = 4 - om; i < j; i = i += 4 | 0) { | ||
H32[off + i >> 2] = buf[i] << 24 | buf[i + 1] << 16 | buf[i + 2] << 8 | buf[i + 3]; | ||
for (i$2 = 4 - om; i$2 < j; i$2 = i$2 += 4 | 0) { | ||
H32[off + i$2 >> 2] = buf[i$2] << 24 | buf[i$2 + 1] << 16 | buf[i$2 + 2] << 8 | buf[i$2 + 3]; | ||
} | ||
@@ -195,8 +194,13 @@ switch (lm) { | ||
}; | ||
// Convert an ArrayBuffer into its hexadecimal string representation. | ||
var hex = function (arrayBuffer) { | ||
var i, x, hex_tab = '0123456789abcdef', res = [], binarray = new Uint8Array(arrayBuffer); | ||
for (i = 0; i < binarray.length; i++) { | ||
x = binarray[i]; | ||
res[i] = hex_tab.charAt(x >> 4 & 15) + hex_tab.charAt(x >> 0 & 15); | ||
var // Precompute 00 - ff strings | ||
precomputedHex = new Array(256); | ||
for (var i = 0; i < 256; i++) { | ||
precomputedHex[i] = (i < 16 ? '0' : '') + i.toString(16); | ||
} | ||
var // Convert an ArrayBuffer into its hexadecimal string representation. | ||
hex = function (arrayBuffer) { | ||
var binarray = new Uint8Array(arrayBuffer); | ||
var res = new Array(arrayBuffer.byteLength); | ||
for (var i$2 = 0; i$2 < res.length; i$2++) { | ||
res[i$2] = precomputedHex[binarray[i$2]]; | ||
} | ||
@@ -211,9 +215,9 @@ return res.join(''); | ||
var p; | ||
// If v is smaller than 2^16, the smallest possible solution | ||
// is 2^16. | ||
if (v <= 65536) | ||
if (// If v is smaller than 2^16, the smallest possible solution | ||
// is 2^16. | ||
v <= 65536) | ||
return 65536; | ||
// If v < 2^24, we round up to 2^n, | ||
// otherwise we round up to 2^24 * n. | ||
if (v < 16777216) { | ||
if (// If v < 2^24, we round up to 2^n, | ||
// otherwise we round up to 2^24 * n. | ||
v < 16777216) { | ||
for (p = 1; p < v; p = p << 1); | ||
@@ -225,4 +229,4 @@ } else { | ||
}; | ||
// Initialize the internal data structures to a new capacity. | ||
var init = function (size) { | ||
var // Initialize the internal data structures to a new capacity. | ||
init = function (size) { | ||
if (size % 64 > 0) { | ||
@@ -264,9 +268,9 @@ throw new Error('Chunk size must be a multiple of 128 bit'); | ||
}; | ||
// Write data to the heap. | ||
var write = function (data, chunkOffset, chunkLen) { | ||
var // Write data to the heap. | ||
write = function (data, chunkOffset, chunkLen) { | ||
convFn(data)(self$2.h8, self$2.h32, chunkOffset, chunkLen, 0); | ||
}; | ||
// Initialize and call the RushaCore, | ||
var // Initialize and call the RushaCore, | ||
// assuming an input buffer of length len * 4. | ||
var coreCall = function (data, chunkOffset, chunkLen, msgLen, finalize) { | ||
coreCall = function (data, chunkOffset, chunkLen, msgLen, finalize) { | ||
var padChunkLen = chunkLen; | ||
@@ -290,13 +294,13 @@ if (finalize) { | ||
}; | ||
// Calculate the hash digest as an array of 5 32bit integers. | ||
var rawDigest = this.rawDigest = function (str) { | ||
var msgLen = str.byteLength || str.length || str.size || 0; | ||
initState(self$2.heap, self$2.padMaxChunkLen); | ||
var chunkOffset = 0, chunkLen = self$2.maxChunkLen, last; | ||
for (chunkOffset = 0; msgLen > chunkOffset + chunkLen; chunkOffset += chunkLen) { | ||
coreCall(str, chunkOffset, chunkLen, msgLen, false); | ||
} | ||
coreCall(str, chunkOffset, msgLen - chunkOffset, msgLen, true); | ||
return getRawDigest(self$2.heap, self$2.padMaxChunkLen); | ||
}; | ||
var // Calculate the hash digest as an array of 5 32bit integers. | ||
rawDigest = this.rawDigest = function (str) { | ||
var msgLen = str.byteLength || str.length || str.size || 0; | ||
initState(self$2.heap, self$2.padMaxChunkLen); | ||
var chunkOffset = 0, chunkLen = self$2.maxChunkLen, last; | ||
for (chunkOffset = 0; msgLen > chunkOffset + chunkLen; chunkOffset += chunkLen) { | ||
coreCall(str, chunkOffset, chunkLen, msgLen, false); | ||
} | ||
coreCall(str, chunkOffset, msgLen - chunkOffset, msgLen, true); | ||
return getRawDigest(self$2.heap, self$2.padMaxChunkLen); | ||
}; | ||
// The digest and digestFrom* interface returns the hash digest | ||
@@ -398,12 +402,14 @@ // as a hex string. | ||
}; | ||
// If we'e running in Node.JS, export a module. | ||
if (typeof module !== 'undefined') { | ||
if (// If we'e running in Node.JS, export a module. | ||
typeof module !== 'undefined') { | ||
module.exports = Rusha; | ||
} else if (typeof window !== 'undefined') { | ||
} else if (// If we're running in a DOM context, export | ||
// the Rusha object to toplevel. | ||
typeof window !== 'undefined') { | ||
window.Rusha = Rusha; | ||
} | ||
// If we're running in a webworker, accept | ||
// messages containing a jobid and a buffer | ||
// or blob object, and return the hash result. | ||
if (typeof FileReaderSync !== 'undefined') { | ||
if (// If we're running in a webworker, accept | ||
// messages containing a jobid and a buffer | ||
// or blob object, and return the hash result. | ||
typeof FileReaderSync !== 'undefined') { | ||
var reader = new FileReaderSync(), hasher = new Rusha(4 * 1024 * 1024); | ||
@@ -410,0 +416,0 @@ self.onmessage = function onMessage(event) { |
@@ -1,2 +0,2 @@ | ||
/*! rusha 2015-06-22 */ | ||
(function(){var a={getDataType:function(a){if(typeof a==="string"){return"string"}if(a instanceof Array){return"array"}if(typeof global!=="undefined"&&global.Buffer&&global.Buffer.isBuffer(a)){return"buffer"}if(a instanceof ArrayBuffer){return"arraybuffer"}if(a.buffer instanceof ArrayBuffer){return"view"}if(a instanceof Blob){return"blob"}throw new Error("Unsupported data type.")}};function b(d){"use strict";var e={fill:0};var f=function(a){for(a+=9;a%64>0;a+=1);return a};var g=function(a,b){for(var c=b>>2;c<a.length;c++)a[c]=0};var h=function(a,b,c){a[b>>2]|=128<<24-(b%4<<3);a[((b>>2)+2&~15)+14]=c>>29;a[((b>>2)+2&~15)+15]=c<<3};var i=function(a,b,c,d,e){var f=this,g,h=e%4,i=d%4,j=d-i;if(j>0){switch(h){case 0:a[e+3|0]=f.charCodeAt(c);case 1:a[e+2|0]=f.charCodeAt(c+1);case 2:a[e+1|0]=f.charCodeAt(c+2);case 3:a[e|0]=f.charCodeAt(c+3)}}for(g=h;g<j;g=g+4|0){b[e+g>>2]=f.charCodeAt(c+g)<<24|f.charCodeAt(c+g+1)<<16|f.charCodeAt(c+g+2)<<8|f.charCodeAt(c+g+3)}switch(i){case 3:a[e+j+1|0]=f.charCodeAt(c+j+2);case 2:a[e+j+2|0]=f.charCodeAt(c+j+1);case 1:a[e+j+3|0]=f.charCodeAt(c+j)}};var j=function(a,b,c,d,e){var f=this,g,h=e%4,i=d%4,j=d-i;if(j>0){switch(h){case 0:a[e+3|0]=f[c];case 1:a[e+2|0]=f[c+1];case 2:a[e+1|0]=f[c+2];case 3:a[e|0]=f[c+3]}}for(g=4-h;g<j;g=g+=4|0){b[e+g>>2]=f[c+g]<<24|f[c+g+1]<<16|f[c+g+2]<<8|f[c+g+3]}switch(i){case 3:a[e+j+1|0]=f[c+j+2];case 2:a[e+j+2|0]=f[c+j+1];case 1:a[e+j+3|0]=f[c+j]}};var k=function(a,b,d,e,f){var g=this,h,i=f%4,j=e%4,k=e-j;var l=new Uint8Array(c.readAsArrayBuffer(g.slice(d,d+e)));if(k>0){switch(i){case 0:a[f+3|0]=l[0];case 1:a[f+2|0]=l[1];case 2:a[f+1|0]=l[2];case 3:a[f|0]=l[3]}}for(h=4-i;h<k;h=h+=4|0){b[f+h>>2]=l[h]<<24|l[h+1]<<16|l[h+2]<<8|l[h+3]}switch(j){case 3:a[f+k+1|0]=l[k+2];case 2:a[f+k+2|0]=l[k+1];case 1:a[f+k+3|0]=l[k]}};var l=function(b){switch(a.getDataType(b)){case"string":return i.bind(b);case"array":return j.bind(b);case"buffer":return j.bind(b);case"arraybuffer":return j.bind(new Uint8Array(b));case"view":return j.bind(new Uint8Array(b.buffer,b.byteOffset,b.byteLength));case"blob":return k.bind(b)}};var m=function(b,c){switch(a.getDataType(b)){case"string":return b.slice(c);case"array":return b.slice(c);case"buffer":return b.slice(c);case"arraybuffer":return b.slice(c);case"view":return b.buffer.slice(c)}};var n=function(a){var b,c,d="0123456789abcdef",e=[],f=new Uint8Array(a);for(b=0;b<f.length;b++){c=f[b];e[b]=d.charAt(c>>4&15)+d.charAt(c>>0&15)}return e.join("")};var o=function(a){var b;if(a<=65536)return 65536;if(a<16777216){for(b=1;b<a;b=b<<1);}else{for(b=16777216;b<a;b+=16777216);}return b};var p=function(a){if(a%64>0){throw new Error("Chunk size must be a multiple of 128 bit")}e.maxChunkLen=a;e.padMaxChunkLen=f(a);e.heap=new ArrayBuffer(o(e.padMaxChunkLen+320+20));e.h32=new Int32Array(e.heap);e.h8=new Int8Array(e.heap);e.core=new b._core({Int32Array:Int32Array,DataView:DataView},{},e.heap);e.buffer=null};p(d||64*1024);var q=function(a,b){var c=new Int32Array(a,b+320,5);c[0]=1732584193;c[1]=-271733879;c[2]=-1732584194;c[3]=271733878;c[4]=-1009589776};var r=function(a,b){var c=f(a);var d=new Int32Array(e.heap,0,c>>2);g(d,a);h(d,a,b);return c};var s=function(a,b,c){l(a)(e.h8,e.h32,b,c,0)};var t=function(a,b,c,d,f){var g=c;if(f){g=r(c,d)}s(a,b,c);e.core.hash(g,e.padMaxChunkLen)};var u=function(a,b){var c=new Int32Array(a,b+320,5);var d=new Int32Array(5);var e=new DataView(d.buffer);e.setInt32(0,c[0],false);e.setInt32(4,c[1],false);e.setInt32(8,c[2],false);e.setInt32(12,c[3],false);e.setInt32(16,c[4],false);return d};var v=this.rawDigest=function(a){var b=a.byteLength||a.length||a.size||0;q(e.heap,e.padMaxChunkLen);var c=0,d=e.maxChunkLen,f;for(c=0;b>c+d;c+=d){t(a,c,d,b,false)}t(a,c,b-c,b,true);return u(e.heap,e.padMaxChunkLen)};this.digest=this.digestFromString=this.digestFromBuffer=this.digestFromArrayBuffer=function(a){return n(v(a).buffer)}}b._core=function e(a,b,c){"use asm";var d=new a.Int32Array(c);function e(a,b){a=a|0;b=b|0;var c=0,e=0,f=0,g=0,h=0,i=0,j=0,k=0,l=0,m=0,n=0,o=0,p=0,q=0;f=d[b+320>>2]|0;h=d[b+324>>2]|0;j=d[b+328>>2]|0;l=d[b+332>>2]|0;n=d[b+336>>2]|0;for(c=0;(c|0)<(a|0);c=c+64|0){g=f;i=h;k=j;m=l;o=n;for(e=0;(e|0)<64;e=e+4|0){q=d[c+e>>2]|0;p=((f<<5|f>>>27)+(h&j|~h&l)|0)+((q+n|0)+1518500249|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;d[a+e>>2]=q}for(e=a+64|0;(e|0)<(a+80|0);e=e+4|0){q=(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])<<1|(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])>>>31;p=((f<<5|f>>>27)+(h&j|~h&l)|0)+((q+n|0)+1518500249|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;d[e>>2]=q}for(e=a+80|0;(e|0)<(a+160|0);e=e+4|0){q=(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])<<1|(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])>>>31;p=((f<<5|f>>>27)+(h^j^l)|0)+((q+n|0)+1859775393|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;d[e>>2]=q}for(e=a+160|0;(e|0)<(a+240|0);e=e+4|0){q=(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])<<1|(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])>>>31;p=((f<<5|f>>>27)+(h&j|h&l|j&l)|0)+((q+n|0)-1894007588|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;d[e>>2]=q}for(e=a+240|0;(e|0)<(a+320|0);e=e+4|0){q=(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])<<1|(d[e-12>>2]^d[e-32>>2]^d[e-56>>2]^d[e-64>>2])>>>31;p=((f<<5|f>>>27)+(h^j^l)|0)+((q+n|0)-899497514|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;d[e>>2]=q}f=f+g|0;h=h+i|0;j=j+k|0;l=l+m|0;n=n+o|0}d[b+320>>2]=f;d[b+324>>2]=h;d[b+328>>2]=j;d[b+332>>2]=l;d[b+336>>2]=n}return{hash:e}};if(typeof module!=="undefined"){module.exports=b}else if(typeof window!=="undefined"){window.Rusha=b}if(typeof FileReaderSync!=="undefined"){var c=new FileReaderSync,d=new b(4*1024*1024);self.onmessage=function f(a){var b,c=a.data.data;try{b=d.digest(c);self.postMessage({id:a.data.id,hash:b})}catch(e){self.postMessage({id:a.data.id,error:e.name})}}}})(); | ||
/*! rusha 2016-09-18 */ | ||
(function(){var a={getDataType:function(a){if(typeof a==="string"){return"string"}if(a instanceof Array){return"array"}if(typeof global!=="undefined"&&global.Buffer&&global.Buffer.isBuffer(a)){return"buffer"}if(a instanceof ArrayBuffer){return"arraybuffer"}if(a.buffer instanceof ArrayBuffer){return"view"}if(a instanceof Blob){return"blob"}throw new Error("Unsupported data type.")}};function b(d){"use strict";var e={fill:0};var f=function(a){for(a+=9;a%64>0;a+=1);return a};var g=function(a,b){for(var c=b>>2;c<a.length;c++)a[c]=0};var h=function(a,b,c){a[b>>2]|=128<<24-(b%4<<3);a[((b>>2)+2&~15)+14]=c/(1<<29)|0;a[((b>>2)+2&~15)+15]=c<<3};var i=function(a,b,c,d,e){var f=this,g,h=e%4,i=d%4,j=d-i;if(j>0){switch(h){case 0:a[e+3|0]=f.charCodeAt(c);case 1:a[e+2|0]=f.charCodeAt(c+1);case 2:a[e+1|0]=f.charCodeAt(c+2);case 3:a[e|0]=f.charCodeAt(c+3)}}for(g=h;g<j;g=g+4|0){b[e+g>>2]=f.charCodeAt(c+g)<<24|f.charCodeAt(c+g+1)<<16|f.charCodeAt(c+g+2)<<8|f.charCodeAt(c+g+3)}switch(i){case 3:a[e+j+1|0]=f.charCodeAt(c+j+2);case 2:a[e+j+2|0]=f.charCodeAt(c+j+1);case 1:a[e+j+3|0]=f.charCodeAt(c+j)}};var j=function(a,b,c,d,e){var f=this,g,h=e%4,i=d%4,j=d-i;if(j>0){switch(h){case 0:a[e+3|0]=f[c];case 1:a[e+2|0]=f[c+1];case 2:a[e+1|0]=f[c+2];case 3:a[e|0]=f[c+3]}}for(g=4-h;g<j;g=g+=4|0){b[e+g>>2]=f[c+g]<<24|f[c+g+1]<<16|f[c+g+2]<<8|f[c+g+3]}switch(i){case 3:a[e+j+1|0]=f[c+j+2];case 2:a[e+j+2|0]=f[c+j+1];case 1:a[e+j+3|0]=f[c+j]}};var k=function(a,b,d,e,f){var g=this,h,i=f%4,j=e%4,k=e-j;var l=new Uint8Array(c.readAsArrayBuffer(g.slice(d,d+e)));if(k>0){switch(i){case 0:a[f+3|0]=l[0];case 1:a[f+2|0]=l[1];case 2:a[f+1|0]=l[2];case 3:a[f|0]=l[3]}}for(h=4-i;h<k;h=h+=4|0){b[f+h>>2]=l[h]<<24|l[h+1]<<16|l[h+2]<<8|l[h+3]}switch(j){case 3:a[f+k+1|0]=l[k+2];case 2:a[f+k+2|0]=l[k+1];case 1:a[f+k+3|0]=l[k]}};var l=function(b){switch(a.getDataType(b)){case"string":return i.bind(b);case"array":return j.bind(b);case"buffer":return j.bind(b);case"arraybuffer":return j.bind(new Uint8Array(b));case"view":return j.bind(new Uint8Array(b.buffer,b.byteOffset,b.byteLength));case"blob":return k.bind(b)}};var m=function(b,c){switch(a.getDataType(b)){case"string":return b.slice(c);case"array":return b.slice(c);case"buffer":return b.slice(c);case"arraybuffer":return b.slice(c);case"view":return b.buffer.slice(c)}};var n=new Array(256);for(var o=0;o<256;o++){n[o]=(o<16?"0":"")+o.toString(16)}var p=function(a){var b=new Uint8Array(a);var c=new Array(a.byteLength);for(var d=0;d<c.length;d++){c[d]=n[b[d]]}return c.join("")};var q=function(a){var b;if(a<=65536)return 65536;if(a<16777216){for(b=1;b<a;b=b<<1);}else{for(b=16777216;b<a;b+=16777216);}return b};var r=function(a){if(a%64>0){throw new Error("Chunk size must be a multiple of 128 bit")}e.maxChunkLen=a;e.padMaxChunkLen=f(a);e.heap=new ArrayBuffer(q(e.padMaxChunkLen+320+20));e.h32=new Int32Array(e.heap);e.h8=new Int8Array(e.heap);e.core=new b._core({Int32Array:Int32Array,DataView:DataView},{},e.heap);e.buffer=null};r(d||64*1024);var s=function(a,b){var c=new Int32Array(a,b+320,5);c[0]=1732584193;c[1]=-271733879;c[2]=-1732584194;c[3]=271733878;c[4]=-1009589776};var t=function(a,b){var c=f(a);var d=new Int32Array(e.heap,0,c>>2);g(d,a);h(d,a,b);return c};var u=function(a,b,c){l(a)(e.h8,e.h32,b,c,0)};var v=function(a,b,c,d,f){var g=c;if(f){g=t(c,d)}u(a,b,c);e.core.hash(g,e.padMaxChunkLen)};var w=function(a,b){var c=new Int32Array(a,b+320,5);var d=new Int32Array(5);var e=new DataView(d.buffer);e.setInt32(0,c[0],false);e.setInt32(4,c[1],false);e.setInt32(8,c[2],false);e.setInt32(12,c[3],false);e.setInt32(16,c[4],false);return d};var x=this.rawDigest=function(a){var b=a.byteLength||a.length||a.size||0;s(e.heap,e.padMaxChunkLen);var c=0,d=e.maxChunkLen,f;for(c=0;b>c+d;c+=d){v(a,c,d,b,false)}v(a,c,b-c,b,true);return w(e.heap,e.padMaxChunkLen)};this.digest=this.digestFromString=this.digestFromBuffer=this.digestFromArrayBuffer=function(a){return p(x(a).buffer)}}b._core=function a(b,c,d){"use asm";var e=new b.Int32Array(d);function f(a,b){a=a|0;b=b|0;var c=0,d=0,f=0,g=0,h=0,i=0,j=0,k=0,l=0,m=0,n=0,o=0,p=0,q=0;f=e[b+320>>2]|0;h=e[b+324>>2]|0;j=e[b+328>>2]|0;l=e[b+332>>2]|0;n=e[b+336>>2]|0;for(c=0;(c|0)<(a|0);c=c+64|0){g=f;i=h;k=j;m=l;o=n;for(d=0;(d|0)<64;d=d+4|0){q=e[c+d>>2]|0;p=((f<<5|f>>>27)+(h&j|~h&l)|0)+((q+n|0)+1518500249|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;e[a+d>>2]=q}for(d=a+64|0;(d|0)<(a+80|0);d=d+4|0){q=(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])<<1|(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])>>>31;p=((f<<5|f>>>27)+(h&j|~h&l)|0)+((q+n|0)+1518500249|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;e[d>>2]=q}for(d=a+80|0;(d|0)<(a+160|0);d=d+4|0){q=(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])<<1|(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])>>>31;p=((f<<5|f>>>27)+(h^j^l)|0)+((q+n|0)+1859775393|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;e[d>>2]=q}for(d=a+160|0;(d|0)<(a+240|0);d=d+4|0){q=(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])<<1|(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])>>>31;p=((f<<5|f>>>27)+(h&j|h&l|j&l)|0)+((q+n|0)-1894007588|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;e[d>>2]=q}for(d=a+240|0;(d|0)<(a+320|0);d=d+4|0){q=(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])<<1|(e[d-12>>2]^e[d-32>>2]^e[d-56>>2]^e[d-64>>2])>>>31;p=((f<<5|f>>>27)+(h^j^l)|0)+((q+n|0)-899497514|0)|0;n=l;l=j;j=h<<30|h>>>2;h=f;f=p;e[d>>2]=q}f=f+g|0;h=h+i|0;j=j+k|0;l=l+m|0;n=n+o|0}e[b+320>>2]=f;e[b+324>>2]=h;e[b+328>>2]=j;e[b+332>>2]=l;e[b+336>>2]=n}return{hash:f}};if(typeof module!=="undefined"){module.exports=b}else if(typeof window!=="undefined"){window.Rusha=b}if(typeof FileReaderSync!=="undefined"){var c=new FileReaderSync,d=new b(4*1024*1024);self.onmessage=function a(b){var c,e=b.data.data;try{c=d.digest(e);self.postMessage({id:b.data.id,hash:c})}catch(a){self.postMessage({id:b.data.id,error:a.name})}}}})(); |
@@ -78,3 +78,5 @@ /* | ||
bin[chunkLen>>2] |= 0x80 << (24 - (chunkLen % 4 << 3)); | ||
bin[(((chunkLen >> 2) + 2) & ~0x0f) + 14] = msgLen >> 29; | ||
// To support msgLen >= 2 GiB, use a float division when computing the | ||
// high 32-bits of the big-endian message length in bits. | ||
bin[(((chunkLen >> 2) + 2) & ~0x0f) + 14] = (msgLen / (1 << 29)) |0; | ||
bin[(((chunkLen >> 2) + 2) & ~0x0f) + 15] = msgLen << 3; | ||
@@ -180,9 +182,14 @@ }; | ||
// Precompute 00 - ff strings | ||
var precomputedHex = new Array(256); | ||
for (var i = 0; i < 256; i++) { | ||
precomputedHex[i] = (i < 0x10 ? '0' : '') + i.toString(16); | ||
} | ||
// Convert an ArrayBuffer into its hexadecimal string representation. | ||
var hex = function (arrayBuffer) { | ||
var i, x, hex_tab = "0123456789abcdef", res = [], binarray = new Uint8Array(arrayBuffer); | ||
for (i = 0; i < binarray.length; i++) { | ||
x = binarray[i]; | ||
res[i] = hex_tab.charAt((x >> 4) & 0xF) + | ||
hex_tab.charAt((x >> 0) & 0xF); | ||
var binarray = new Uint8Array(arrayBuffer); | ||
var res = new Array(arrayBuffer.byteLength); | ||
for (var i = 0; i < res.length; i++) { | ||
res[i] = precomputedHex[binarray[i]]; | ||
} | ||
@@ -189,0 +196,0 @@ return res.join(''); |
@@ -53,2 +53,65 @@ (function () { | ||
// To execute the tests, run `npm test`, then open `test/test.html` in | ||
// Safari. The tests work in Firefox when loaded via HTTP, e.g. from | ||
// `localhost`. The 1 and 2 GiB tests fail in Chrome with `NotFoundError` | ||
// when loaded via HTTP. | ||
if (typeof Worker !== 'undefined') { | ||
describe('webworker', function () { | ||
it('1 kiB', function(done) { | ||
var rw = new Worker('../rusha.min.js') | ||
var zero1k = new Int8Array(1024); | ||
var blob = new Blob([zero1k]); | ||
rw.onmessage = function (e) { | ||
if (e.data.error) { | ||
throw e.data.error; | ||
} | ||
assert.strictEqual('60cacbf3d72e1e7834203da608037b1bf83b40e8', e.data.hash); | ||
done(); | ||
} | ||
rw.postMessage({ id: 0, data: blob }) | ||
}); | ||
it('1 MiB', function(done) { | ||
var rw = new Worker('../rusha.min.js') | ||
var zero1M = new Int8Array(1024 * 1024); | ||
var blob = new Blob([zero1M]); | ||
rw.onmessage = function (e) { | ||
if (e.data.error) { | ||
throw e.data.error; | ||
} | ||
assert.strictEqual('3b71f43ff30f4b15b5cd85dd9e95ebc7e84eb5a3', e.data.hash); | ||
done(); | ||
} | ||
rw.postMessage({ id: 0, data: blob }) | ||
}); | ||
it('1 GiB', function(done) { | ||
this.timeout(30 * 1000); | ||
var rw = new Worker('../rusha.min.js') | ||
var zero1M = new Int8Array(1024 * 1024); | ||
var blob = new Blob(Array(1024).fill(zero1M)); | ||
rw.onmessage = function (e) { | ||
if (e.data.error) { | ||
throw e.data.error; | ||
} | ||
assert.strictEqual('2a492f15396a6768bcbca016993f4b4c8b0b5307', e.data.hash); | ||
done(); | ||
} | ||
rw.postMessage({ id: 0, data: blob }) | ||
}); | ||
it('2 GiB', function(done) { | ||
this.timeout(60 * 1000); | ||
var rw = new Worker('../rusha.min.js') | ||
var zero1M = new Int8Array(1024 * 1024); | ||
var blob = new Blob(Array(2 * 1024).fill(zero1M)); | ||
rw.onmessage = function (e) { | ||
if (e.data.error) { | ||
throw e.data.error; | ||
} | ||
assert.strictEqual('91d50642dd930e9542c39d36f0516d45f4e1af0d', e.data.hash); | ||
done(); | ||
} | ||
rw.postMessage({ id: 0, data: blob }) | ||
}); | ||
}); | ||
} | ||
describe('digestFromString', function() { | ||
@@ -62,6 +125,6 @@ it('returns hex string from string', function() { | ||
it('returns hex string from buffer', function() { | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digest(abcBuffer)); | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digestFromBuffer(abcBuffer)); | ||
}); | ||
it('returns hex string from array', function() { | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digest(abcArray)); | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digestFromBuffer(abcArray)); | ||
}); | ||
@@ -72,3 +135,3 @@ }); | ||
it('returns hex string from ArrayBuffer', function() { | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digest(abcArrayBuffer)); | ||
assert.strictEqual('a9993e364706816aba3e25717850c26c9cd0d89d', r.digestFromArrayBuffer(abcArrayBuffer)); | ||
}); | ||
@@ -75,0 +138,0 @@ }); |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 2 instances in 1 package
High entropy strings
Supply chain riskContains high entropy strings. This could be a sign of encrypted data, leaked secrets or obfuscated code.
Found 1 instance in 1 package
Long strings
Supply chain riskContains long string literals, which may be a sign of obfuscated or packed code.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
444942
16
11176
14
7
2