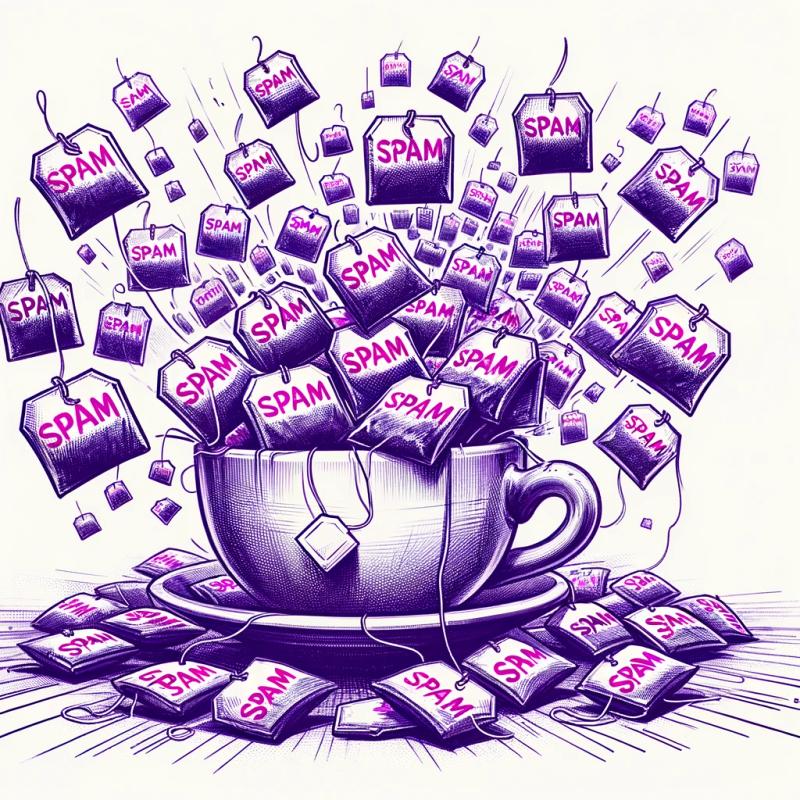
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
scroll-event-handler
Advanced tools
Readme
Scroll event handler is a NPM package est un package for reading scroll (desktop) or touch (mobile) events and their assigned a callback.
The online demo gives you an overview of what can be done with this package.
You can also see the use of this package on my portfolio.
For this npm ESLint is used with the basic parameters to have a clean code.
It can be installed from npm.
$ npm install scroll-event-handler
The minimal configuration for scroll listener is this bellow :
import ScrollListener from 'scroll-event-handler';
let newScrollListener = new ScrollListener({callback: () => console.log('scroll')});
With this configuration scroll listener will fire the callback regardless of the direction using the defaults settings. for further use an example are available below.
container
description :
Type | Description |
---|---|
string | Default value : 'main' Using a querySelector you will have to indicate the
container to listen to with the appropriate string. |
cancelOnDirectionChange
description :
Type | Description |
---|---|
boolean | Default value : true
Defined if the scroll counter should be reset if the direction of the scroll changes. |
callback
description :
Type | Description |
---|---|
function | Default value : () => {}
Allows you to define a global callback which will be called at each trigger before the other callbacks. |
scroll
description :
Type | Description | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
object | Either:
An object encapsulating the various configuration parameters for all possible scrolls (X / Y - next / prev).
|
touch
description :
Type | Description | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
object | Either:
An object encapsulating the various configuration parameters for all possible touch (X / Y - next / prev).
|
Several methods can be called after creating the listener. You will find them below.
removeScrollListener()
: allows to suppress the listener.
switchCancelOnDirectionChange()
: allows to switch the bolean value of cancelOnDirectionChange
.
changeSettings(Object)
: allows you to modify the parameters given when creating the listener. It takes the same options as when creating the listener.
You can find the way to call them in the examples section.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
});
// newScrollListener listen the scroll and touch event in your #myListenedElement.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
scroll: {
y: {
value: 5,
callback: () => console.log('Hello scroll world'),
},
},
touch: {
y: {
value: 200,
callback: () => console.log('Hello touch world'),
},
},
});
// now you console.log a string if user verticaly scroll/touch.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
scroll: {
x: {
prev: {
value: 5,
callback: () => console.log('prev scroll'),
},
next: {
value: 2,
callback: () => console.log('next scroll'),
},
},
},
touch: {
x: {
prev: {
value: 200,
callback: () => console.log('prev touch'),
},
next: {
value: 150,
callback: () => console.log('next touch'),
},
},
},
});
// sent you a different console.log depending on whether the user scrolls forward or backward on the horizontal axis
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
callback: () => console.log('my global callback'),
scroll: {
x: {
prev: {
value: 5,
callback: () => console.log('prev scroll'),
},
next: {
value: 2,
callback: () => console.log('next scroll'),
},
},
},
touch: {
x: {
prev: {
value: 200,
callback: () => console.log('prev touch'),
},
next: {
value: 150,
callback: () => console.log('next touch'),
},
},
},
});
// during each scroll listened to, your global callback function will be called before the others.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
});
newScrollListener.removeScrollListener();
//the listener is now removed.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
cancelOnDirectionChange: true
});
newScrollListener.switchCancelOnDirectionChange();
// now the cancelOnDirectionChange is false.
let newScrollListener = new ScrollListener({
container: '#myListenedElement',
scroll: {
x: {
prev: {
value: 5,
callback: () => console.log('prev scroll'),
},
next: {
value: 2,
callback: () => console.log('next scroll'),
},
},
},
touch: {
x: {
prev: {
value: 200,
callback: () => console.log('prev touch'),
},
next: {
value: 150,
callback: () => console.log('next touch'),
},
},
},
});
newScrollListener.changeSettings({
scroll: {
x: {
prev: {
value: 10,
},
},
},
touch: {
x: {
prev: {
value: 300,
},
},
},
});
// now scroll.x.prev value is 10 and the touch.x.prev.value is 300.
The tests were carried out thanks to Browserstack which offers us its services since our npm is opensource.
The npm is now run on all devices and browsers but it's possible that problems may be encountered on browsers that may be common. If this is the case, do not hesitate to open an issue.
If you encounter a problem or a bug due to the NPM package do not hesitate to open an issue with the corresponding label and a detailed description of your problem.
See also the list of contributors who participated in this project.
Want to contribute to the project ? First read your document on how to contribute effectively.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Scroll event handler is a NPM package est un package for reading scroll (desktop) or touch (mobile) events and their assigned a callback.
The npm package scroll-event-handler receives a total of 1 weekly downloads. As such, scroll-event-handler popularity was classified as not popular.
We found that scroll-event-handler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.