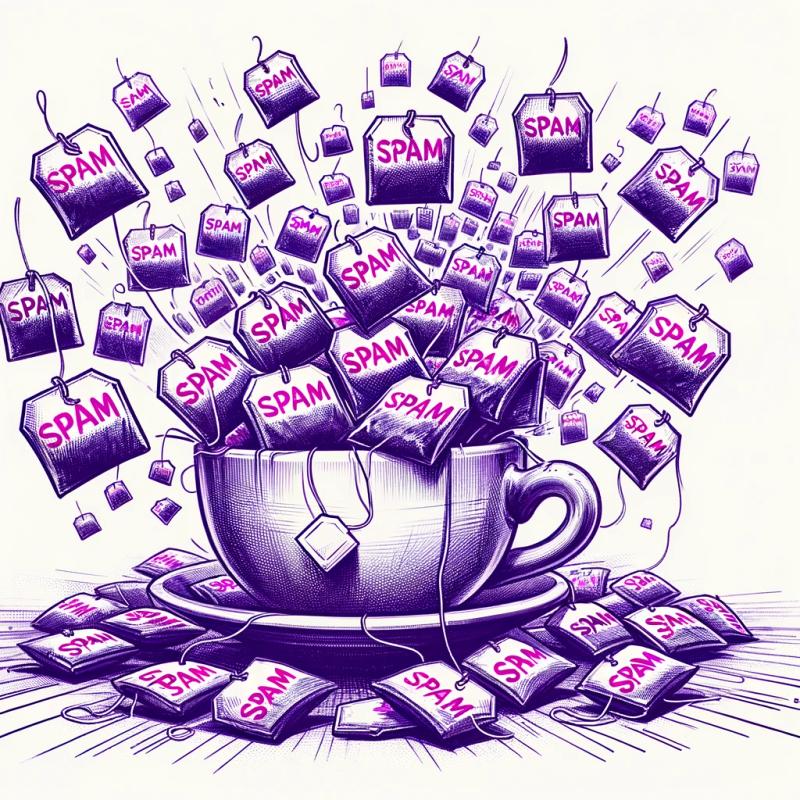
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
set-value
Advanced tools
Package description
The set-value npm package is a utility for setting deeply nested values within an object using dot-path notation or by providing a path array. It is useful for updating values in a mutable way without directly accessing properties, especially when dealing with complex or deeply nested objects.
Set nested values using dot-path
This feature allows you to set a value at a nested path within an object using a string dot-path notation.
const setValue = require('set-value');
const obj = {};
setValue(obj, 'a.b.c', 'value');
console.log(obj); //=> { a: { b: { c: 'value' } } }
Set nested values using array-path
This feature allows you to set a value at a nested path within an object using an array to describe the path.
const setValue = require('set-value');
const obj = {};
setValue(obj, ['a', 'b', 'c'], 'value');
console.log(obj); //=> { a: { b: { c: 'value' } } }
Set multiple values
This feature allows you to set multiple values at different paths within the same object.
const setValue = require('set-value');
const obj = {};
setValue(obj, 'a.b.c', 'value1');
setValue(obj, 'x.y.z', 'value2');
console.log(obj); //=> { a: { b: { c: 'value1' } }, x: { y: { z: 'value2' } } }
lodash.set is a method from the Lodash library that performs a similar function to set-value. It allows setting values at a given path of an object tree. Lodash is a more extensive utility library, and lodash.set is just one of its many functions.
dot-prop is another npm package that allows you to get, set, or delete properties from a nested object using dot-path notation. It is similar to set-value but also provides methods for getting and deleting properties.
deepdash is an extension for Lodash that adds deep object manipulation capabilities. It provides methods for deep setting, getting, and many other operations on nested objects. It is more feature-rich compared to set-value, which focuses solely on setting values.
Readme
Create nested values and any intermediaries using dot notation (
'a.b.c'
) paths.
Please consider following this project's author, Jon Schlinkert, and consider starring the project to show your :heart: and support.
Install with npm:
$ npm install --save set-value
Please update to version 3.0.1 or later, a critical bug was fixed in that version.
const set = require('set-value');
set(object, prop, value);
object
{object}: The object to set value
onprop
{string}: The property to set. Dot-notation may be used.value
{any}: The value to set on object[prop]
Updates and returns the given object:
const obj = {};
set(obj, 'a.b.c', 'd');
console.log(obj);
//=> { a: { b: { c: 'd' } } }
Escaping with backslashes
Prevent set-value from splitting on a dot by prefixing it with backslashes:
console.log(set({}, 'a\\.b.c', 'd'));
//=> { 'a.b': { c: 'd' } }
console.log(set({}, 'a\\.b\\.c', 'd'));
//=> { 'a.b.c': 'd' }
(benchmarks were run on a MacBook Pro 2.5 GHz Intel Core i7, 16 GB 1600 MHz DDR3).
set-value is more reliable and has more features than dot-prop, without sacrificing performance.
# deep (194 bytes)
deep-object x 629,744 ops/sec ±0.85% (88 runs sampled)
deep-property x 1,470,427 ops/sec ±0.94% (89 runs sampled)
deep-set x 1,401,089 ops/sec ±1.02% (91 runs sampled)
deephas x 590,005 ops/sec ±1.73% (86 runs sampled)
dot-prop x 1,261,408 ops/sec ±0.94% (90 runs sampled)
dot2val x 1,672,729 ops/sec ±1.12% (89 runs sampled)
es5-dot-prop x 1,313,018 ops/sec ±0.79% (91 runs sampled)
lodash-set x 1,074,464 ops/sec ±0.97% (93 runs sampled)
object-path-set x 961,198 ops/sec ±2.07% (74 runs sampled)
object-set x 258,438 ops/sec ±0.69% (90 runs sampled)
set-value x 1,976,843 ops/sec ±2.07% (89 runs sampled)
fastest is set-value (by 186% avg)
# medium (98 bytes)
deep-object x 3,249,287 ops/sec ±1.04% (93 runs sampled)
deep-property x 3,409,307 ops/sec ±1.28% (88 runs sampled)
deep-set x 3,240,776 ops/sec ±1.13% (93 runs sampled)
deephas x 960,504 ops/sec ±1.39% (89 runs sampled)
dot-prop x 2,776,388 ops/sec ±0.80% (94 runs sampled)
dot2val x 3,889,791 ops/sec ±1.28% (91 runs sampled)
es5-dot-prop x 2,779,604 ops/sec ±1.32% (91 runs sampled)
lodash-set x 2,791,304 ops/sec ±0.75% (90 runs sampled)
object-path-set x 2,462,084 ops/sec ±1.51% (91 runs sampled)
object-set x 838,569 ops/sec ±0.87% (90 runs sampled)
set-value x 4,767,287 ops/sec ±1.21% (91 runs sampled)
fastest is set-value (by 181% avg)
# shallow (101 bytes)
deep-object x 4,793,168 ops/sec ±0.75% (88 runs sampled)
deep-property x 4,669,218 ops/sec ±1.17% (90 runs sampled)
deep-set x 4,648,247 ops/sec ±0.73% (91 runs sampled)
deephas x 1,246,414 ops/sec ±1.67% (92 runs sampled)
dot-prop x 3,913,694 ops/sec ±1.23% (89 runs sampled)
dot2val x 5,428,829 ops/sec ±0.76% (92 runs sampled)
es5-dot-prop x 3,897,931 ops/sec ±1.19% (92 runs sampled)
lodash-set x 6,128,638 ops/sec ±0.95% (87 runs sampled)
object-path-set x 5,429,978 ops/sec ±3.31% (87 runs sampled)
object-set x 1,529,485 ops/sec ±2.37% (89 runs sampled)
set-value x 7,150,921 ops/sec ±1.58% (89 runs sampled)
fastest is set-value (by 172% avg)
Clone this library into a local directory:
$ git clone https://github.com/jonschlinkert/set-value.git
Then install devDependencies and run benchmarks:
$ npm install && node benchmark
These are just a few of the duplicate libraries on NPM.
this
being used improperly in the methods. I was able to patch it by binding the (plain) object to the methods, but it still fails 17 of 26 unit tests.Others that do the same thing, but use a completely different API
split
function to be passed on the options.If there are any regressions please create a bug report. Thanks!
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
You might also be interested in these projects:
'a.b.c'
) paths. | homepageCommits | Contributor |
---|---|
73 | jonschlinkert |
2 | mbelsky |
1 | doowb |
1 | GlennKintscher |
1 | vadimdemedes |
1 | wtgtybhertgeghgtwtg |
Jon Schlinkert
Copyright © 2020, Jon Schlinkert. Released under the MIT License.
This file was generated by verb-generate-readme, v0.8.0, on April 01, 2020.
FAQs
Set nested properties on an object using dot notation.
The npm package set-value receives a total of 13,051,183 weekly downloads. As such, set-value popularity was classified as popular.
We found that set-value demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.