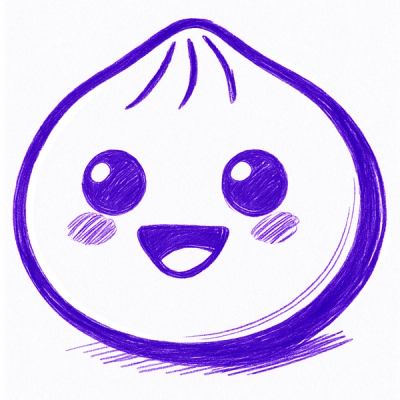
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
solid-bucket
Advanced tools
A universal API for Cloud Storage providers. Supports: Amazon AWS S3, Backblaze B2, Microsoft Azure Blob, DigitalOcean Spaces, Rackspace Cloud Storage, Wasabi Object Storage and any S3-Compatible cloud storage or Folder (e.g NAS)
Use the same API to communicate with every Cloud providers. We built a solid abstraction that you can rely on.
This version introduces a completely new API, so it contains code-breaking changes. We encorauge you to update, but please keep in mind that some refactoring will be required.
npm install solid-bucket
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('aws', {
accessKeyId: 'accessKeyId',
secretAccessKey: 'secretAccessKey',
region: 'us-east-1' // Optional: "us-east-1" by default
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('b2', {
accountId: 'accountId',
applicationKey: 'applicationKey'
})
const SolidBucket = require('solid-bucket')
// How to generate a credentials JSON file: https://cloud.google.com/storage/docs/authentication (To generate a private key in JSON format)
let provider = new SolidBucket('gcs', {
keyFilename: 'keyFilename'
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('digitalocean', {
accessKeyId: 'accessKeyId',
secretAccessKey: 'secretAccessKey',
region: 'nyc3' // Optional: "nyc3" by default
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('rackspace', {
username: 'username',
apiKey: 'apiKey',
region: 'IAD' // Optional: 'IAD' by default
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('wasabi', {
accessKeyId: 'accessKeyId',
secretAccessKey: 'secretAccessKey',
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('s3compatible', {
endpoint: 'endpoint',
accessKeyId: 'accessKeyId',
secretAccessKey: 'secretAccessKey',
})
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('folder', {
folderPath: 'folderPath'
})
provider.createBucket(bucketName) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
provider.createBucket(bucketName).then((resp) => {
if (resp.status === 201) {
console.log(resp.message)
// Output: Bucket "example" was created successfully
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.deleteBucket(bucketName) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
provider.deleteBucket(bucketName).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Bucket "example" was deleted successfully
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.uploadFile(bucketName, filePath) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
let filePath = '/tmp/file.bin'
provider.uploadFile(bucketName, filePath).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Bucket "example" was deleted successfully
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.deleteBucket(bucketName, remoteFilename downloadedFilePath) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
let remoteFilename = 'file.bin'
let downloadedFilePath = '/tmp'
provider.downloadFile(bucketName, remoteFilename, downloadedFilePath).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Bucket "example" was deleted successfully
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.createFileFromText(bucketName, remoteFilename, text) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
let remoteFilename = 'my_remote_object.txt'
let text = 'This is a text that I would like to upload'
provider.createFileFromText(bucketName, remoteFilename, text).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Object "example.txt" was saved successfully in bucket "example"
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.deleteFile(bucketName, remoteFilename) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
let remoteFilename = 'my_remote_object.txt'
provider.deleteFile(bucketName, remoteFilename).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Object "example.txt" was deleted successfully from bucket "example"
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.readFile(bucketName, remoteFilename) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
let remoteFilename = 'my_remote_object.txt'
provider.readFile(bucketName, remoteFilename).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: Object "example.txt" was fetched successfully from bucket "example"
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
provider.getListOfFiles(bucketName) // returns a Promise
const SolidBucket = require('solid-bucket')
let provider = new SolidBucket('azure', {
accountName: 'accountName',
accountKey: 'accountKey'
})
let bucketName = 'example'
provider.getListOfFiles(bucketName).then((resp) => {
if (resp.status === 200) {
console.log(resp.message)
// Output: The list of objects was fetched successfully from bucket "example"
}
}).catch((resp) => {
if (resp.status === 400){
console.log(resp.message)
// Output: Some error coming from the provider...
}
})
Required Environmental vars depending on the provider:
Afterwards, just run:
npm run compile && npm test
MIT
FAQs
A universal API for Cloud Storage providers. Supports: Amazon AWS S3, Backblaze B2, Microsoft Azure Blob, DigitalOcean Spaces, Rackspace Cloud Storage, Wasabi Object Storage and any S3-Compatible cloud storage or Folder (e.g NAS)
The npm package solid-bucket receives a total of 33 weekly downloads. As such, solid-bucket popularity was classified as not popular.
We found that solid-bucket demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.