spacetrim
Advanced tools
Comparing version 0.6.2 to 0.7.0
@@ -28,7 +28,7 @@ /*! ***************************************************************************** | ||
function topTrim(str) { | ||
function topTrim(content) { | ||
var e_1, _a; | ||
var linesWithContent = []; | ||
var contentStarted = false; | ||
var lines = str.split('\n'); | ||
var lines = content.split('\n'); | ||
try { | ||
@@ -55,20 +55,27 @@ for (var lines_1 = __values(lines), lines_1_1 = lines_1.next(); !lines_1_1.done; lines_1_1 = lines_1.next()) { | ||
function verticalTrim(str) { | ||
str = topTrim(str); | ||
str = topTrim(str.split('\n').reverse().join('\n')) | ||
function verticalTrim(content) { | ||
content = topTrim(content); | ||
content = topTrim(content.split('\n').reverse().join('\n')) | ||
.split('\n') | ||
.reverse() | ||
.join('\n'); | ||
return str; | ||
return content; | ||
} | ||
function spaceTrim(str /* TODO: , char: RegExp = /\s/*/) { | ||
function spaceTrim(content) { | ||
var isFunctional = false; | ||
if (typeof content === 'function') { | ||
isFunctional = true; | ||
content = content(function (blockContent) { | ||
return blockContent.split('\n').join('__NEWLINE__'); | ||
}); | ||
} | ||
// ✂️ Trimming from top and bottom | ||
str = verticalTrim(str); | ||
content = verticalTrim(content); | ||
// ✂️ Trimming from left and right | ||
var lines = str.split('\n'); | ||
var lines = content.split('\n'); | ||
var lineStats = lines | ||
.filter(function (line) { return line.trim() !== ''; }) | ||
.map(function (line) { | ||
var contentStart = line.length - line.trimLeft().length; | ||
var contentStart = line.length - line.trimStart().length; | ||
var contentEnd = contentStart + line.trim().length; | ||
@@ -93,9 +100,23 @@ return { contentStart: contentStart, contentEnd: contentEnd }; | ||
}), minContentStart = _a.minContentStart, maxContentEnd = _a.maxContentEnd; | ||
str = lines | ||
.map(function (line) { return line.substring(minContentStart, maxContentEnd); }) | ||
.join('\n'); | ||
return str; | ||
var linesHorizontalyTrimmed = lines.map(function (line) { | ||
return line.substring(minContentStart, maxContentEnd); | ||
}); | ||
if (isFunctional) { | ||
linesHorizontalyTrimmed = linesHorizontalyTrimmed.map(function (line) { | ||
var sublines = line.split('__NEWLINE__'); | ||
var firstSubine = sublines[0]; | ||
var contentStart = firstSubine.length - firstSubine.trimStart().length; | ||
var indentation = ' '.repeat(contentStart); | ||
return sublines | ||
.map(function (subline) { return "" + indentation + subline.trimStart(); }) | ||
.join('\n'); | ||
}); | ||
} | ||
return linesHorizontalyTrimmed.join('\n'); | ||
} | ||
/** | ||
* TODO: Allow to change split char , char: RegExp = /\s/ | ||
*/ | ||
export { spaceTrim as default, spaceTrim }; | ||
//# sourceMappingURL=spaceTrim.js.map |
export default spaceTrim; | ||
export declare function spaceTrim(str: string): string; | ||
export declare function spaceTrim(content: string | ((block: (blockContent: string) => string) => string)): string; | ||
/** | ||
* TODO: Allow to change split char , char: RegExp = /\s/ | ||
*/ |
@@ -1,1 +0,1 @@ | ||
export declare function topTrim(str: string): string; | ||
export declare function topTrim(content: string): string; |
@@ -1,1 +0,1 @@ | ||
export declare function verticalTrim(str: string): string; | ||
export declare function verticalTrim(content: string): string; |
@@ -34,7 +34,7 @@ (function (global, factory) { | ||
function topTrim(str) { | ||
function topTrim(content) { | ||
var e_1, _a; | ||
var linesWithContent = []; | ||
var contentStarted = false; | ||
var lines = str.split('\n'); | ||
var lines = content.split('\n'); | ||
try { | ||
@@ -61,20 +61,27 @@ for (var lines_1 = __values(lines), lines_1_1 = lines_1.next(); !lines_1_1.done; lines_1_1 = lines_1.next()) { | ||
function verticalTrim(str) { | ||
str = topTrim(str); | ||
str = topTrim(str.split('\n').reverse().join('\n')) | ||
function verticalTrim(content) { | ||
content = topTrim(content); | ||
content = topTrim(content.split('\n').reverse().join('\n')) | ||
.split('\n') | ||
.reverse() | ||
.join('\n'); | ||
return str; | ||
return content; | ||
} | ||
function spaceTrim(str /* TODO: , char: RegExp = /\s/*/) { | ||
function spaceTrim(content) { | ||
var isFunctional = false; | ||
if (typeof content === 'function') { | ||
isFunctional = true; | ||
content = content(function (blockContent) { | ||
return blockContent.split('\n').join('__NEWLINE__'); | ||
}); | ||
} | ||
// ✂️ Trimming from top and bottom | ||
str = verticalTrim(str); | ||
content = verticalTrim(content); | ||
// ✂️ Trimming from left and right | ||
var lines = str.split('\n'); | ||
var lines = content.split('\n'); | ||
var lineStats = lines | ||
.filter(function (line) { return line.trim() !== ''; }) | ||
.map(function (line) { | ||
var contentStart = line.length - line.trimLeft().length; | ||
var contentStart = line.length - line.trimStart().length; | ||
var contentEnd = contentStart + line.trim().length; | ||
@@ -99,7 +106,21 @@ return { contentStart: contentStart, contentEnd: contentEnd }; | ||
}), minContentStart = _a.minContentStart, maxContentEnd = _a.maxContentEnd; | ||
str = lines | ||
.map(function (line) { return line.substring(minContentStart, maxContentEnd); }) | ||
.join('\n'); | ||
return str; | ||
var linesHorizontalyTrimmed = lines.map(function (line) { | ||
return line.substring(minContentStart, maxContentEnd); | ||
}); | ||
if (isFunctional) { | ||
linesHorizontalyTrimmed = linesHorizontalyTrimmed.map(function (line) { | ||
var sublines = line.split('__NEWLINE__'); | ||
var firstSubine = sublines[0]; | ||
var contentStart = firstSubine.length - firstSubine.trimStart().length; | ||
var indentation = ' '.repeat(contentStart); | ||
return sublines | ||
.map(function (subline) { return "" + indentation + subline.trimStart(); }) | ||
.join('\n'); | ||
}); | ||
} | ||
return linesHorizontalyTrimmed.join('\n'); | ||
} | ||
/** | ||
* TODO: Allow to change split char , char: RegExp = /\s/ | ||
*/ | ||
@@ -106,0 +127,0 @@ exports["default"] = spaceTrim; |
export default spaceTrim; | ||
export declare function spaceTrim(str: string): string; | ||
export declare function spaceTrim(content: string | ((block: (blockContent: string) => string) => string)): string; | ||
/** | ||
* TODO: Allow to change split char , char: RegExp = /\s/ | ||
*/ |
@@ -1,1 +0,1 @@ | ||
export declare function topTrim(str: string): string; | ||
export declare function topTrim(content: string): string; |
@@ -1,1 +0,1 @@ | ||
export declare function verticalTrim(str: string): string; | ||
export declare function verticalTrim(content: string): string; |
{ | ||
"name": "spacetrim", | ||
"version": "0.6.2", | ||
"version": "0.7.0", | ||
"description": "Trimming string from all 4 sides", | ||
@@ -5,0 +5,0 @@ "sideEffects": false, |
# ✂️ Space trim | ||
[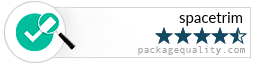](https://packagequality.com/#?package=spacetrim) | ||
[](https://packagequality.com/#?package=spacetrim) | ||
[](https://www.npmjs.com/package/spacetrim) | ||
@@ -47,1 +47,35 @@ [](https://github.com/hejny/spacetrim/actions/workflows/test.yml) | ||
``` | ||
_See more examples in [simple spaceTrim tests](./src/spaceTrim.test.ts)._ | ||
## Nesting | ||
This is very usefull when you want to trim multiline strings inside multiline strings. | ||
```typescript | ||
import { spaceTrim } from 'spacetrim'; | ||
const trimmed = spaceTrim( | ||
(block) => ` | ||
Numbers | ||
${block(['1', '2', '3'].join('\n'))} | ||
Chars | ||
${block(['A', 'B', 'C'].join('\n'))} | ||
`, | ||
); | ||
console.log(trimmed); | ||
/*Numbers | ||
1 | ||
2 | ||
3 | ||
Chars | ||
A | ||
B | ||
C*/ | ||
``` | ||
_See more examples in [nesting tests](./src/nesting.test.ts)._ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
47411
26
303
81