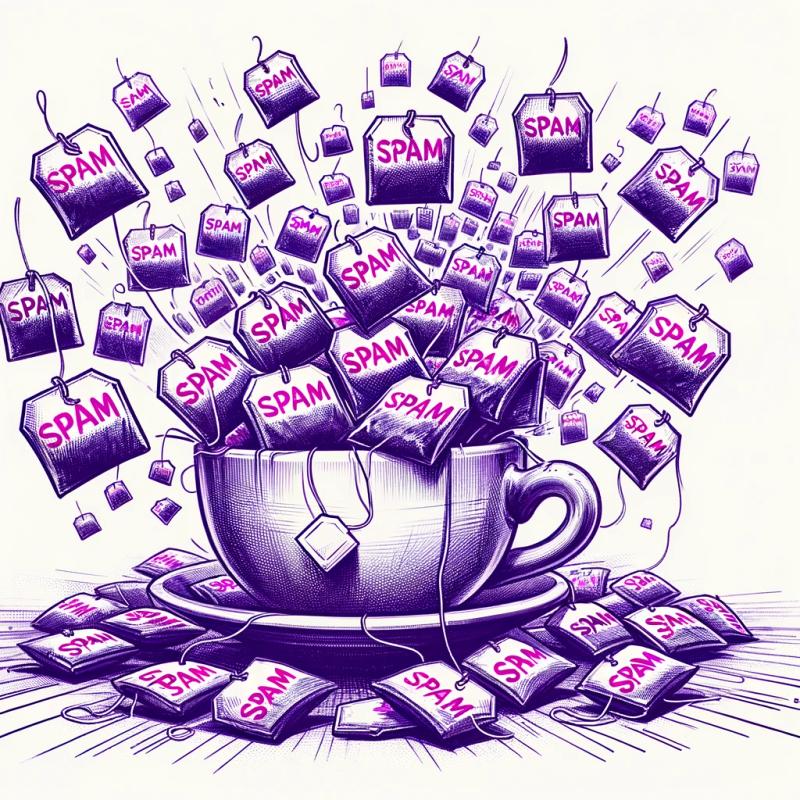
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
sqrolls
Advanced tools
Readme
A simple sql-like expression builder that makes it easy to build sql-like expressions using a hierarchical builder pattern. The resulting statements should work for most sql databases however it was primarily written to support working with sqlite3.
Currently only simple update, insert, and select queries are being supported.
The usage is written for a sqlite3 db. We will be writing example queries for a users
table with the following schema. The sqroll query builders don't interface directly with
a db but rather only build a valid sql expressions with substitutions in place of user
arguments. Each query builder has a toSQL
method that returns a tuple of the final
sql expression and the arguments that can be given as inputs directly to a db connection.
CREATE TABLE users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
email TEXT NOT NULL,
archived_at NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
);
Selecting a specific user's name and email.
const qb = Select.builder()
.select('name', 'email')
.from('users')
.whereIsEqual('id', 1);
const [ stmt, args ] = qb.toSQL();
// stmt: 'SELECT * FROM users WHERE id = ?'
// args: [1]
Selecting all users that haven't been archived.
const qb = Select.builder()
.select('*')
.from('users')
.whereIsNotNull('archived_at');
const [ stmt, args ] = qb.toSQL();
// stmt: 'SELECT * FROM users WHERE archived_at IS NOT NULL'
// args: []
Selecting all users with names that start with "Frank".
const qb = Select.builder()
.select('*')
.from('users')
.whereIsLike('name', 'Frank%')
const [ stmt, args ] = qb.toSQL();
// stmt: 'SELECT * FROM users WHERE name LIKE ?'
// args: ['Frank%']
We sometimes need to query a table based on a search filter with optional fields.
The conditional where methods can help with this. Searching for archived users if
isArchived
filter is true.
const filter = {
isArchived: false,
};
const qb = Select.builder()
.select('*')
.from('users')
.whereIsNotNullIf('archived_at', filter.isArchived);
const [ stmt, args ] = qb.toSQL();
// stmt: 'SELECT * FROM users'
// args: []
Selecting all users with ids in the given list.
const qb = Select.builder()
.select('*')
.from('users')
.whereIn('id', 1, 2, 3, 4)
const [ stmt, args ] = qb.toSQL();
// stmt: 'SELECT * FROM users WHERE id IN (?,?,?,?)'
// args: [1, 2, 3, 4]
Inserting a single user.
const qb = Insert.builder()
.into('users')
.setColumns('name', 'email')
.addValues('frank', 'warthog@wolfcola.com');
const [ stmt, args ] = qb.toSQL();
// stmt: 'INSERT INTO users (name, email) VALUES (?, ?)'
// args: ['frank', 'warthog@wolfcola.com']
Bulk inserting multiple users.
const qb = Insert.builder()
.into('users')
.setColumns('name', 'email')
.addValues('frank', 'warthog@wolfcola.com')
.addValues('dennis', 'dennis@paddys.com')
.addValues('charlie', 'charlie@paddys.com');
const [ stmt, args ] = qb.toSQL();
// stmt: 'INSERT INTO users (name, email) VALUES (?, ?), (?, ?), (?, ?)'
// args: ['frank', 'warthog@wolfcola.com', 'dennis', 'dennis@paddys.com', 'mac', 'mac@paddys.com']
Updating all users in the table to have the same name.
const qb = Update.builder()
.setTable('users')
.set('name', 'mac');
const [ stmt, args ] = qb.toSQL();
// stmt: 'UPDATE users SET name = ?'
// args: ['mac']
Updating user matching the given predicates.
const qb = Update.builder()
.setTable('users')
.set('name', 'mac')
.whereIsEqual('id', 4)
.whereIsNotNull('archived_at');
const [ stmt, args ] = qb.toSQL();
// stmt: 'UPDATE users SET name = ? WHERE id = ? AND archived_at IS NOT NULL'
// args: ['mac', 4]
FAQs
sql builder library
The npm package sqrolls receives a total of 1 weekly downloads. As such, sqrolls popularity was classified as not popular.
We found that sqrolls demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.