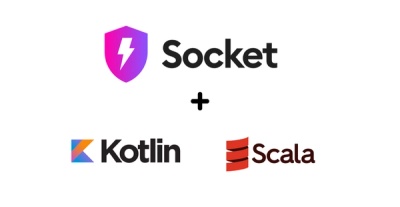
Product
Introducing Scala and Kotlin Support in Socket
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
string-format
Advanced tools
The string-format npm package is a utility for formatting strings in a variety of ways. It allows you to insert values into placeholders within a string, making it easier to create dynamic and readable strings.
Basic Formatting
This feature allows you to insert values into placeholders within a string. The placeholders are denoted by curly braces {}.
const format = require('string-format');
const result = format('Hello, {}!', 'World');
console.log(result); // Output: Hello, World!
Named Placeholders
Named placeholders allow you to use keys from an object to insert values into the string. This makes the code more readable and easier to manage.
const format = require('string-format');
const result = format('Hello, {name}!', { name: 'Alice' });
console.log(result); // Output: Hello, Alice!
Indexed Placeholders
Indexed placeholders use numerical indices to insert values into the string. This is useful when you have an array of values to insert.
const format = require('string-format');
const result = format('Hello, {0} {1}!', 'John', 'Doe');
console.log(result); // Output: Hello, John Doe!
The sprintf-js package provides a similar functionality to string-format but follows the sprintf syntax commonly used in C. It offers more advanced formatting options, such as padding and precision control.
The util.format function is a built-in Node.js utility that provides basic string formatting capabilities. It is less feature-rich compared to string-format but is sufficient for simple use cases.
The lodash.template function from the lodash library offers a more powerful and flexible templating system. It supports custom delimiters and more complex logic within the templates, making it suitable for more advanced use cases.
string-format is a small JavaScript library for formatting strings, based on
Python's str.format()
. For example:
'"{firstName} {lastName}" <{email}>'.format(user)
// => '"Jane Smith" <jsmith@example.com>'
The equivalent concatenation:
'"' + user.firstName + ' ' + user.lastName + '" <' + user.email + '>'
// => '"Jane Smith" <jsmith@example.com>'
Install:
$ npm install string-format
Require:
const format = require('string-format')
Define window.format
:
<script src="path/to/string-format.js"></script>
string-format can be used in two modes: function mode and method mode.
format('Hello, {}!', 'Alice')
// => 'Hello, Alice!'
In this mode the first argument is a template string and the remaining arguments are values to be interpolated.
'Hello, {}!'.format('Alice')
// => 'Hello, Alice!'
In this mode values to be interpolated are supplied to the format
method
of a template string. This mode is not enabled by default. The method must
first be defined via format.extend
:
format.extend(String.prototype, {})
format(template, $0, $1, …, $N)
and template.format($0, $1, …, $N)
can then
be used interchangeably.
format(template, $0, $1, …, $N)
Returns the result of replacing each {…}
placeholder in the template string
with its corresponding replacement.
Placeholders may contain numbers which refer to positional arguments:
'{0}, you have {1} unread message{2}'.format('Holly', 2, 's')
// => 'Holly, you have 2 unread messages'
Unmatched placeholders produce no output:
'{0}, you have {1} unread message{2}'.format('Steve', 1)
// => 'Steve, you have 1 unread message'
A format string may reference a positional argument multiple times:
"The name's {1}. {0} {1}.".format('James', 'Bond')
// => "The name's Bond. James Bond."
Positional arguments may be referenced implicitly:
'{}, you have {} unread message{}'.format('Steve', 1)
// => 'Steve, you have 1 unread message'
A format string must not contain both implicit and explicit references:
'My name is {} {}. Do you like the name {0}?'.format('Lemony', 'Snicket')
// => ValueError: cannot switch from implicit to explicit numbering
{{
and }}
in format strings produce {
and }
:
'{{}} creates an empty {} in {}'.format('dictionary', 'Python')
// => '{} creates an empty dictionary in Python'
Dot notation may be used to reference object properties:
const bobby = {firstName: 'Bobby', lastName: 'Fischer'}
const garry = {firstName: 'Garry', lastName: 'Kasparov'}
'{0.firstName} {0.lastName} vs. {1.firstName} {1.lastName}'.format(bobby, garry)
// => 'Bobby Fischer vs. Garry Kasparov'
0.
may be omitted when referencing a property of {0}
:
const repo = {owner: 'davidchambers', slug: 'string-format'}
'https://github.com/{owner}/{slug}'.format(repo)
// => 'https://github.com/davidchambers/string-format'
If the referenced property is a method, it is invoked with no arguments to determine the replacement:
const sheldon = {
firstName: 'Sheldon',
lastName: 'Cooper',
dob: new Date('1970-01-01'),
fullName: function() { return this.firstName + ' ' + this.lastName },
quip: function() { return 'Bazinga!' },
}
'{fullName} was born at precisely {dob.toISOString}'.format(sheldon)
// => 'Sheldon Cooper was born at precisely 1970-01-01T00:00:00.000Z'
"I've always wanted to go to a goth club. {quip.toUpperCase}".format(sheldon)
// => "I've always wanted to go to a goth club. BAZINGA!"
format.create(transformers)
This function takes an object mapping names to transformers and returns a
formatting function. A transformer is applied if its name appears, prefixed
with !
, after a field name in a template string.
const fmt = format.create({
escape: s => s.replace(/[&<>"'`]/g, c => '&#' + c.charCodeAt(0) + ';'),
upper: s => s.toUpperCase(),
})
fmt('Hello, {!upper}!', 'Alice')
// => 'Hello, ALICE!'
const restaurant = {name: 'Anchor & Hope', url: 'http://anchorandhopesf.com/'}
fmt('<a href="{url!escape}">{name!escape}</a>', restaurant)
// => '<a href="http://anchorandhopesf.com/">Anchor & Hope</a>'
format.extend(prototype, transformers)
This function takes a prototype (presumably String.prototype
) and an object
mapping names to transformers, and defines a format
method on the prototype.
A transformer is applied if its name appears, prefixed with !
, after a field
name in a template string.
format.extend(String.prototype, {
escape: s => s.replace(/[&<>"'`]/g, c => '&#' + c.charCodeAt(0) + ';'),
upper: s => s.toUpperCase(),
})
'Hello, {!upper}!'.format('Alice')
// => 'Hello, ALICE!'
const restaurant = {name: 'Anchor & Hope', url: 'http://anchorandhopesf.com/'}
'<a href="{url!escape}">{name!escape}</a>'.format(restaurant)
// => '<a href="http://anchorandhopesf.com/">Anchor & Hope</a>'
$ npm install
$ npm test
FAQs
String formatting inspired by Python's str.format()
The npm package string-format receives a total of 650,346 weekly downloads. As such, string-format popularity was classified as popular.
We found that string-format demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.