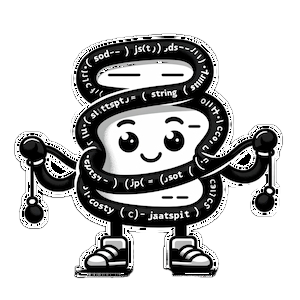
Stringy ( _S )
✨ Lodash but for Strings!

Overview
Stringy is a swiss army knife of string manipulation methods.
Designed to ace string manipulation, a crucial aspect in many applications. It's a go to tool for formatting user-generated content, ensuring uniformity in blogs or comments. E-commerce sites use it to enhance product descriptions for SEO and readability. Data analysts find it invaluable for cleaning datasets, like trimming whitespace or standardizing formats.
Table of Contents
Installation
Stringy goes by the name _S in npm, Install it and watch your strings do backflips:
npm install _S
Or if you're more of a yarn person:
yarn add _S
Usage
-
Got numbers hiding in your text? We'll find them. It's like hide and seek, but with digits.
import { extractNumbers } from "_S";
const text = "Order 500 units of item 1234.";
const numbers = extractNumbers(text);
-
Check for Balanced Brackets
Ever wonder if your brackets are socially balanced? Let's find out together.
import { isBalancedBrackets } from "_S";
const validString = "(Hello [World])";
const isValid = isBalancedBrackets(validString);
-
Masking Sensitive Data
Run a user data centric app? Give your id's a subtle disguise. You choose the disguise.
We cover emails, phones, and cards!
const email = "user@example.com";
const maskedEmail = maskEmail(email, "*");
const dashedEmail = maskEmail(email, "-");
console.log(maskedEmail);
console.log(dashedEmail);
-
Moderate Content
Ensure your text stays in the safe zone with moderate. This function is like the diligent editor of your strings, replacing those not-so-appropriate words with a mask of your choice, ensuring every sentence is audience-friendly.
import { moderate } from "_S";
const originalText = "Stringy is awesome but some words need moderation.";
const wordsToCensor = ["awesome", "moderation"];
const safeText = moderate(originalText, wordsToCensor, "*");
console.log(safeText);
-
Turn into a Title
Give your text the royal treatment – each word's first letter ascends to its uppercase throne.
import { toTitleCase } from "_S";
const humbleText = "i am stringy";
const titled = toTitleCase(humbleText);
-
Trim Whitespace
Give your string a neat trim, because everyone loves a well-groomed text.
import { trimWhitespace } from "_S";
const messy = " Too much space? ";
const neat = trimWhitespace(messy);
-
Word Wrapper
Wrap your text neatly with wordWrap, the function that ensures your strings never spill out of their bounds. Ideal for crafting perfectly formatted paragraphs, be it in a console, an article, or anywhere text needs to stay within the lines.
const longText =
"This is a long string that needs to be wrapped at a specific width.";
const wrappedText = wordWrap(longText, 20);
console.log(wrappedText);
-
Shorten
trims your string to the desired length and tastefully tops it off with an ellipsis (...) and a custom ending, hinting there's more for the eager reader.
import { shorten } from "_S";
const blog =
"Meet Stringy, the latest JavaScript library designed to revolutionize how we handle strings. Whether you're a seasoned developer";
const shortened = shorten(blog, 40, "Read More");
-
Create a Random String
Create a custom string with a sprinkle of unpredictability. Perfect for generating unique IDs, playful codes, or testing your apps with diverse inputs.
import { randomString } from "_S";
const desiredLength = 10;
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const uniqueString = randomString(desiredLength, characters);
Dive into a world where each call brings a surprise, tailor-made just for you! 🎲✨🔡
-
Hunt a Word
a treasure hunt in your strings, where you discover the hidden postions of your sought-after substrings.
import { findPositions } from "_S";
const narrative = "Echoes in the echoes in the echoes";
const echoLocations = findPositions(narrative, "echoes");
-
Number Makeover
Spruce up your digits with formatNumber – it's like a spa day for your numbers, turning them from plain to absolutely fabulous, all dressed up with commas and style.
import { formatNumber } from "_S";
const largeNumber = 1234567;
const beautifiedNumber = formatNumber(largeNumber);
-
Time Makeover
Step into the time machine with formatDateTime. Transform dates and times from mundane to meaningful, showcasing them in a format that speaks to humans, not just machines.
import { formatDateTime } from "_S";
const eventTimestamp = new Date("2023-07-21T19:30:00");
const formattedDateTime = formatDateTime(eventTimestamp);
-
Bracket Balancer
Navigate the tightrope of syntax with isBalancedBrackets. It's like a gymnast gracefully ensuring every leap has a landing – every open bracket finds its closing match.
import { isBalancedBrackets } from "_S";
const codeSnippet = "{[()]}";
const isBalanced = isBalancedBrackets(codeSnippet);
-
The Levenshtein Distance
Measure the steps between strings with levenshteinDistance. It's like having a pedometer for your text, counting the edits required to transition from one string to another.
import { levenshteinDistance } from "_S";
const stringA = "kitten";
const stringB = "sitting";
const editDistance = levenshteinDistance(stringA, stringB);
All Available Methods
capitalize
: Capitalizes the first letter of a string.camelCase
: Converts a string to camel case.snakeCase
: Converts a string to snake case.toAlternateCase
: Converts a string to alternate case (e.g., "hElLo WoRlD").capitalizeFirstLetter
: Capitalizes the first letter of each word in a string.toTitleCase
: Converts a string to title case.invertCase
: Inverts the case of each character in a string.reverseString
: Reverses the characters in a string.isBlank
: Checks if a string is blank or consists only of whitespace.truncate
: Shortens a string to a specified length and adds an ellipsis.countVowels
: Counts the number of vowels in a string.findPositions
: Finds all occurrences of a substring and returns their indices.formatNumber
: Formats a number within a string for readability.formatDateTime
: Formats a date and time string into a more readable format.isBalancedBrackets
: Checks if brackets in a string are balanced.levenshteinDistance
: Calculates the Levenshtein distance between two strings.toggleCase
: Toggles the case of each character in a string.sliceString
: Extracts a portion of a string.toUpperCase
: Converts a string to uppercase.toLowerCase
: Converts a string to lowercase.countWords
: Counts the number of words in a string.highlightPattern
: Highlights occurrences of a pattern within a string.shuffleString
: Randomizes the order of characters in a string.matchesPattern
: Checks if a string matches a regular expression pattern.spliceString
: Modifies a string by removing, replacing, or inserting characters.
How to Contribute
Got ideas? Tricks up your sleeve? Join the _S circus! Fork the repo, make your feature branch, and let the pull requests fly!
Check out our contribution guide for more details.
License
This code is freer than a string in the wild. Check out [appropriate license name] for the details.
Support
Running into strings attached? Let us know! Open an issue or send a pull request. We're here to untangle the mess.