svelte-fast-marquee
Advanced tools
Comparing version 0.3.2 to 0.4.0
@@ -108,2 +108,6 @@ (function (global, factory) { | ||
} | ||
function listen(node, event, handler, options) { | ||
node.addEventListener(event, handler, options); | ||
return () => node.removeEventListener(event, handler, options); | ||
} | ||
function attr(node, attribute, value) { | ||
@@ -118,2 +122,55 @@ if (value == null) | ||
} | ||
// unfortunately this can't be a constant as that wouldn't be tree-shakeable | ||
// so we cache the result instead | ||
let crossorigin; | ||
function is_crossorigin() { | ||
if (crossorigin === undefined) { | ||
crossorigin = false; | ||
try { | ||
if (typeof window !== 'undefined' && window.parent) { | ||
void window.parent.document; | ||
} | ||
} | ||
catch (error) { | ||
crossorigin = true; | ||
} | ||
} | ||
return crossorigin; | ||
} | ||
function add_resize_listener(node, fn) { | ||
const computed_style = getComputedStyle(node); | ||
if (computed_style.position === 'static') { | ||
node.style.position = 'relative'; | ||
} | ||
const iframe = element('iframe'); | ||
iframe.setAttribute('style', 'display: block; position: absolute; top: 0; left: 0; width: 100%; height: 100%; ' + | ||
'overflow: hidden; border: 0; opacity: 0; pointer-events: none; z-index: -1;'); | ||
iframe.setAttribute('aria-hidden', 'true'); | ||
iframe.tabIndex = -1; | ||
const crossorigin = is_crossorigin(); | ||
let unsubscribe; | ||
if (crossorigin) { | ||
iframe.src = "data:text/html,<script>onresize=function(){parent.postMessage(0,'*')}</script>"; | ||
unsubscribe = listen(window, 'message', (event) => { | ||
if (event.source === iframe.contentWindow) | ||
fn(); | ||
}); | ||
} | ||
else { | ||
iframe.src = 'about:blank'; | ||
iframe.onload = () => { | ||
unsubscribe = listen(iframe.contentWindow, 'resize', fn); | ||
}; | ||
} | ||
append(node, iframe); | ||
return () => { | ||
if (crossorigin) { | ||
unsubscribe(); | ||
} | ||
else if (unsubscribe && iframe.contentWindow) { | ||
unsubscribe(); | ||
} | ||
detach(iframe); | ||
}; | ||
} | ||
@@ -341,3 +398,3 @@ let current_component; | ||
function add_css(target) { | ||
append_styles(target, "svelte-9h83db", ".marquee-container.svelte-9h83db.svelte-9h83db{display:flex;width:100%;overflow-x:hidden;flex-direction:row;position:relative}.marquee-container.svelte-9h83db:hover .marquee.svelte-9h83db{animation-play-state:var(--pause-on-hover)}.marquee-container.svelte-9h83db:active .marquee.svelte-9h83db{animation-play-state:var(--pause-on-click)}.marquee.svelte-9h83db.svelte-9h83db{flex:0 0 auto;min-width:100%;z-index:1;display:flex;flex-direction:row;align-items:center;animation:svelte-9h83db-scroll 10s linear 0s infinite;animation-play-state:running;animation-direction:normal;animation-direction:var(--direction)}@keyframes svelte-9h83db-scroll{0%{transform:translateX(0%)}100%{transform:translateX(-100%)}}"); | ||
append_styles(target, "svelte-xy1rj0", ".marquee-container.svelte-xy1rj0.svelte-xy1rj0{display:flex;width:100%;overflow-x:hidden;flex-direction:row;position:relative}.marquee-container.svelte-xy1rj0:hover .marquee.svelte-xy1rj0{animation-play-state:var(--pause-on-hover)}.marquee-container.svelte-xy1rj0:active .marquee.svelte-xy1rj0{animation-play-state:var(--pause-on-click)}.marquee.svelte-xy1rj0.svelte-xy1rj0{flex:0 0 auto;min-width:100%;z-index:1;display:flex;flex-direction:row;align-items:center;animation:svelte-xy1rj0-scroll var(--duration) linear 0s infinite;animation-play-state:var(--play);animation-direction:normal;animation-direction:var(--direction)}@keyframes svelte-xy1rj0-scroll{0%{transform:translateX(0%)}100%{transform:translateX(-100%)}}"); | ||
} | ||
@@ -350,7 +407,8 @@ | ||
let div1; | ||
let div2_resize_listener; | ||
let current; | ||
const default_slot_template = /*#slots*/ ctx[6].default; | ||
const default_slot = create_slot(default_slot_template, ctx, /*$$scope*/ ctx[5], null); | ||
const default_slot_template_1 = /*#slots*/ ctx[6].default; | ||
const default_slot_1 = create_slot(default_slot_template_1, ctx, /*$$scope*/ ctx[5], null); | ||
const default_slot_template = /*#slots*/ ctx[10].default; | ||
const default_slot = create_slot(default_slot_template, ctx, /*$$scope*/ ctx[9], null); | ||
const default_slot_template_1 = /*#slots*/ ctx[10].default; | ||
const default_slot_1 = create_slot(default_slot_template_1, ctx, /*$$scope*/ ctx[9], null); | ||
@@ -365,8 +423,9 @@ return { | ||
if (default_slot_1) default_slot_1.c(); | ||
attr(div0, "class", "marquee svelte-9h83db"); | ||
attr(div0, "style", /*_marqueeStyle*/ ctx[0]); | ||
attr(div1, "class", "marquee svelte-9h83db"); | ||
attr(div1, "style", /*_marqueeStyle*/ ctx[0]); | ||
attr(div2, "class", "marquee-container svelte-9h83db"); | ||
attr(div2, "style", /*_style*/ ctx[1]); | ||
attr(div0, "class", "marquee svelte-xy1rj0"); | ||
attr(div0, "style", /*_marqueeStyle*/ ctx[1]); | ||
attr(div1, "class", "marquee svelte-xy1rj0"); | ||
attr(div1, "style", /*_marqueeStyle*/ ctx[1]); | ||
attr(div2, "class", "marquee-container svelte-xy1rj0"); | ||
attr(div2, "style", /*_style*/ ctx[2]); | ||
add_render_callback(() => /*div2_elementresize_handler*/ ctx[11].call(div2)); | ||
}, | ||
@@ -388,2 +447,3 @@ m(target, anchor) { | ||
div2_resize_listener = add_resize_listener(div2, /*div2_elementresize_handler*/ ctx[11].bind(div2)); | ||
current = true; | ||
@@ -393,23 +453,23 @@ }, | ||
if (default_slot) { | ||
if (default_slot.p && (!current || dirty & /*$$scope*/ 32)) { | ||
update_slot(default_slot, default_slot_template, ctx, /*$$scope*/ ctx[5], !current ? -1 : dirty, null, null); | ||
if (default_slot.p && (!current || dirty & /*$$scope*/ 512)) { | ||
update_slot(default_slot, default_slot_template, ctx, /*$$scope*/ ctx[9], !current ? -1 : dirty, null, null); | ||
} | ||
} | ||
if (!current || dirty & /*_marqueeStyle*/ 1) { | ||
attr(div0, "style", /*_marqueeStyle*/ ctx[0]); | ||
if (!current || dirty & /*_marqueeStyle*/ 2) { | ||
attr(div0, "style", /*_marqueeStyle*/ ctx[1]); | ||
} | ||
if (default_slot_1) { | ||
if (default_slot_1.p && (!current || dirty & /*$$scope*/ 32)) { | ||
update_slot(default_slot_1, default_slot_template_1, ctx, /*$$scope*/ ctx[5], !current ? -1 : dirty, null, null); | ||
if (default_slot_1.p && (!current || dirty & /*$$scope*/ 512)) { | ||
update_slot(default_slot_1, default_slot_template_1, ctx, /*$$scope*/ ctx[9], !current ? -1 : dirty, null, null); | ||
} | ||
} | ||
if (!current || dirty & /*_marqueeStyle*/ 1) { | ||
attr(div1, "style", /*_marqueeStyle*/ ctx[0]); | ||
if (!current || dirty & /*_marqueeStyle*/ 2) { | ||
attr(div1, "style", /*_marqueeStyle*/ ctx[1]); | ||
} | ||
if (!current || dirty & /*_style*/ 2) { | ||
attr(div2, "style", /*_style*/ ctx[1]); | ||
if (!current || dirty & /*_style*/ 4) { | ||
attr(div2, "style", /*_style*/ ctx[2]); | ||
} | ||
@@ -432,2 +492,3 @@ }, | ||
if (default_slot_1) default_slot_1.d(detaching); | ||
div2_resize_listener(); | ||
} | ||
@@ -438,2 +499,3 @@ }; | ||
function instance($$self, $$props, $$invalidate) { | ||
let duration; | ||
let _style; | ||
@@ -445,13 +507,27 @@ let _marqueeStyle; | ||
let { direction = 'left' } = $$props; | ||
let { speed = 100 } = $$props; | ||
let { play = true } = $$props; | ||
let containerWidth; | ||
function div2_elementresize_handler() { | ||
containerWidth = this.clientWidth; | ||
$$invalidate(0, containerWidth); | ||
} | ||
$$self.$$set = $$props => { | ||
if ('pauseOnHover' in $$props) $$invalidate(2, pauseOnHover = $$props.pauseOnHover); | ||
if ('pauseOnClick' in $$props) $$invalidate(3, pauseOnClick = $$props.pauseOnClick); | ||
if ('direction' in $$props) $$invalidate(4, direction = $$props.direction); | ||
if ('$$scope' in $$props) $$invalidate(5, $$scope = $$props.$$scope); | ||
if ('pauseOnHover' in $$props) $$invalidate(3, pauseOnHover = $$props.pauseOnHover); | ||
if ('pauseOnClick' in $$props) $$invalidate(4, pauseOnClick = $$props.pauseOnClick); | ||
if ('direction' in $$props) $$invalidate(5, direction = $$props.direction); | ||
if ('speed' in $$props) $$invalidate(6, speed = $$props.speed); | ||
if ('play' in $$props) $$invalidate(7, play = $$props.play); | ||
if ('$$scope' in $$props) $$invalidate(9, $$scope = $$props.$$scope); | ||
}; | ||
$$self.$$.update = () => { | ||
if ($$self.$$.dirty & /*pauseOnHover, pauseOnClick*/ 12) { | ||
$$invalidate(1, _style = ` | ||
if ($$self.$$.dirty & /*containerWidth, speed*/ 65) { | ||
$$invalidate(8, duration = containerWidth / speed); | ||
} | ||
if ($$self.$$.dirty & /*pauseOnHover, pauseOnClick*/ 24) { | ||
$$invalidate(2, _style = ` | ||
--pause-on-hover: ${pauseOnHover ? 'paused' : 'running'}; | ||
@@ -462,5 +538,7 @@ --pause-on-click: ${pauseOnClick ? 'paused' : 'running'}; | ||
if ($$self.$$.dirty & /*direction*/ 16) { | ||
$$invalidate(0, _marqueeStyle = ` | ||
if ($$self.$$.dirty & /*play, direction, duration*/ 416) { | ||
$$invalidate(1, _marqueeStyle = ` | ||
--play: ${play ? 'running' : 'paused'}; | ||
--direction: ${direction === 'left' ? 'normal' : 'reverse'}; | ||
--duration: ${duration}s; | ||
`); | ||
@@ -470,3 +548,16 @@ } | ||
return [_marqueeStyle, _style, pauseOnHover, pauseOnClick, direction, $$scope, slots]; | ||
return [ | ||
containerWidth, | ||
_marqueeStyle, | ||
_style, | ||
pauseOnHover, | ||
pauseOnClick, | ||
direction, | ||
speed, | ||
play, | ||
duration, | ||
$$scope, | ||
slots, | ||
div2_elementresize_handler | ||
]; | ||
} | ||
@@ -485,5 +576,7 @@ | ||
{ | ||
pauseOnHover: 2, | ||
pauseOnClick: 3, | ||
direction: 4 | ||
pauseOnHover: 3, | ||
pauseOnClick: 4, | ||
direction: 5, | ||
speed: 6, | ||
play: 7 | ||
}, | ||
@@ -490,0 +583,0 @@ add_css |
{ | ||
"name": "svelte-fast-marquee", | ||
"version": "0.3.2", | ||
"version": "0.4.0", | ||
"description": "A Marquee component for Svelte inspired by react-fast-marquee.", | ||
@@ -5,0 +5,0 @@ "main": "dist/index.js", |
# Svelte Fast Marquee | ||
A Marquee component for Svelte inspired by [react-fast-marquee](https://github.com/justin-chu/react-fast-marquee). | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
[](https://bundlephobia.com/result?p=svelte-fast-marquee) | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
[](https://bundlephobia.com/result?p=svelte-fast-marquee) | ||
[](https://www.npmjs.com/package/svelte-fast-marquee) | ||
@@ -9,0 +9,0 @@ [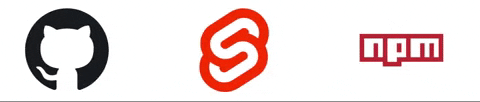](https://media.giphy.com/media/eKiQ1t5UuSj76KFNqg/giphy.gif) |
@@ -9,3 +9,3 @@ /// <reference types="svelte" /> | ||
*/ | ||
pauseOnHover?: booleaan; | ||
pauseOnHover?: boolean; | ||
@@ -19,6 +19,18 @@ /** | ||
/** | ||
* Specify the kind of button | ||
* Animation direction | ||
* @default 'left' | ||
*/ | ||
direction?: "left" | "right"; | ||
/** | ||
* Animation speed calculated as pixels/second | ||
* @default 100 | ||
*/ | ||
speed?: number; | ||
/** | ||
* Animation state | ||
* @default true | ||
*/ | ||
play?: boolean; | ||
} | ||
@@ -25,0 +37,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
44776
1136