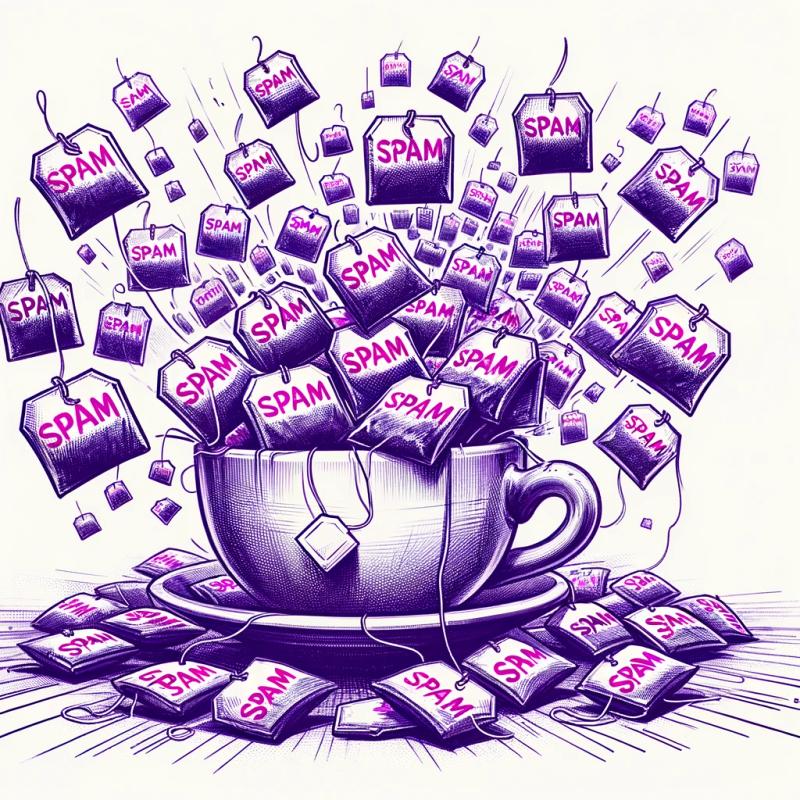
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
swagger-endpoint-validator
Advanced tools
Readme
A validator of API endpoints to check that input and/or output match with the swagger specification for the API.
This is based on express-swagger-generator, so it is important that each endpoints is properly documented so that the library can do the validation.
npm install --save swagger-endpoint-validator
validator.init(app, validatorOptions, format);
where:
app
is the Express app instance.format
is an string to choose the format we want to create the swagger docs: jsdoc
or yaml
. Default jsdoc
.validatorOptions
is a configuration object that has different format depending on format
:validatorOptions
for jsdoc
const validatorOptions = {
swaggerDefinition: {
info: {
description: 'Documentation for Service API',
title: 'Service API',
version: '1.0.0',
contact: { email: 'your_email@guidesmiths.com' },
},
host: 'localhost:5000',
basePath: '/',
produces: ['application/json'],
schemes: ['http'],
securityDefinitions: {
JWT: {
type: 'apiKey',
in: 'header',
name: 'Authorization',
description: '',
},
},
},
basedir: process.cwd(), // app absolute path
files: ['./test/**/**.js'], // path to the API handle folder, related to basedir
route: {
url: '/test/docs/api',
docs: '/test/docs/api.json',
},
};
validatorOptions
for yaml
const validatorOptions = {
swaggerDefinition: {
info: {
description: 'Documentation for Service API',
title: 'Service API',
version: '1.0.0',
contact: { email: 'your_email@guidesmiths.com' },
},
basePath: '/',
},
apis: ['./test/**/**.js'], // paths to the API files
url: '/test/docs/api', // optional path to serve the API documentation
};
validator.validateAPIInput(input, request);
where:
input
is the payload to be validated.request
is request object.It will use the configuration used in the initialization to look for the endpoint and the schema to validate.
JSDOC Example
/**
* @typedef Input
* @property {string} name.required
*/
/**
* @typedef Output
* @property {string} name.required
* @property {string} result.required
*/
/**
* @route GET /test-invalid-output
* @summary Create a new group
* @group test - Everything about tests
* @param {Input.model} body.body.required
* @returns {Output.model} 200 - Successful operation
* @returns {Error.model} <any> - Error message
* @security JWT
*/
app.get('/test-invalid-input', (req, res) => {
try {
validator.validateAPIInput({}, req);
} catch (error) {
res.status(404).json({ error });
}
});
YAML Example
/**
* @swagger
* /test-invalid-input:
* post:
* description: Test POST /test-invalid-input
* tags: [Test]
* produces:
* - application/json
* parameters:
* - name: body
* description: Input payload
* required: true
* type: object
* schema:
* - $ref: '#/definitions/Input'
* responses:
* 200:
* description: successful operation
*/
app.post('/test-invalid-input', (req, res) => {
try {
const result = validator.validateAPIInput({}, req);
res.status(200).json(result);
} catch (error) {
res.status(404).json({ error });
}
});
validator.validateAPIOutput(output, request);
where:
output
is the payload to be validated.request
is request object.It will use the configuration used in the initialization to look for the endpoint and the schema to validate.
JSDOC Example
/**
* @typedef Input
* @property {string} name.required
*/
/**
* @typedef Output
* @property {string} name.required
* @property {string} result.required
*/
/**
* @route GET /test-invalid-output
* @summary Create a new group
* @group test - Everything about tests
* @param {Input.model} body.body.required
* @returns {Output.model} 200 - Successful operation
* @returns {Error.model} <any> - Error message
* @security JWT
*/
app.get('/test-invalid-output', (req, res) => {
const validInputModel = { name: 'Name is required' };
try {
validator.validateAPIInput(validInputModel, req);
validator.validateAPIOutput({}, req);
} catch (error) {
res.status(404).json({ error });
}
});
YAML Example
/**
* @swagger
* /test-invalid-output:
* get:
* description: Test GET /test-invalid-output
* tags: [Test]
* produces:
* - application/json
* responses:
* 200:
* description: successful operation
* schema:
* $ref: '#/definitions/Output'
*/
app.get('/test-invalid-output', (req, res) => {
try {
const result = validator.validateAPIOutput({}, req);
res.status(200).json(result);
} catch (error) {
res.status(404).json({ error });
}
});
JSDOC
/**
* @typedef Input
* @property {string} name.required
*/
/**
* @typedef Output
* @property {string} name.required
* @property {string} result.required
*/
/**
* @route GET /test-valid
* @summary Create a new group
* @group test - Everything about tests
* @param {Input.model} body.body.required
* @returns {Output.model} 200 - Successful operation
* @returns {Error.model} <any> - Error message
* @security JWT
*/
app.get('/test-valid', (req, res) => {
const validInputModel = { name: 'Name is required' };
const validOutputModel = { name: 'Name is required', result: 'Valid result' };
validator.validateAPIInput(validInputModel, req);
validator.validateAPIOutput(validOutputModel, req);
res.status(200).json({ success: true });
});
YAML
/**
* @swagger
* /test-valid-input:
* post:
* description: Test POST /test-valid-input
* tags: [Test]
* produces:
* - application/json
* parameters:
* - name: body
* description: Input payload
* required: true
* type: object
* schema:
* - $ref: '#/definitions/Input'
* responses:
* 200:
* description: successful operation
*/
app.post('/test-valid-input', (req, res) => {
const validInputModel = { name: 'Name is required' };
try {
const result = validator.validateAPIInput(validInputModel, req);
res.status(200).json(result);
} catch (error) {
res.status(404).json({ error });
}
});
/**
* @swagger
* /test-valid-output:
* get:
* description: Test GET /test-valid-output
* tags: [Test]
* produces:
* - application/json
* parameters:
* - name: body
* description: Input payload
* required: true
* type: object
* schema:
* - $ref: '#/definitions/Input'
* responses:
* 200:
* description: successful operation
* schema:
* $ref: '#/definitions/Output'
*/
app.get('/test-valid-output', (req, res) => {
const validInputModel = { name: 'Name is required' };
const validOutputModel = { name: 'Name is required', result: 'Valid result' };
try {
validator.validateAPIInput(validInputModel, req);
const result = validator.validateAPIOutput(validOutputModel, req);
res.status(200).json(result);
} catch (error) {
res.status(404).json({ error });
}
});
FAQs
A validator of API endpoints to check that input and output match with the swagger specification for the API
The npm package swagger-endpoint-validator receives a total of 92 weekly downloads. As such, swagger-endpoint-validator popularity was classified as not popular.
We found that swagger-endpoint-validator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.