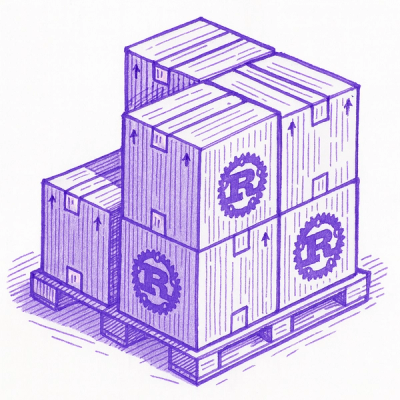
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
swagger-parser
Advanced tools
Parses a JSON or YAML Swagger spec, validates it against the Swagger schema, and dereferences all $ref pointers
swagger-parser is a powerful npm package that allows you to parse, validate, and dereference Swagger (OpenAPI) definitions. It helps in ensuring that your API definitions are correct and can be used to resolve $ref pointers to simplify the API documentation.
Parse
This feature allows you to parse a Swagger (OpenAPI) definition file. The code sample demonstrates how to parse a Swagger file and log the API name and version.
const SwaggerParser = require('swagger-parser');
SwaggerParser.parse('path/to/your/swagger.yaml')
.then(api => {
console.log('API name: %s, Version: %s', api.info.title, api.info.version);
})
.catch(err => {
console.error(err);
});
Validate
This feature allows you to validate a Swagger (OpenAPI) definition file. The code sample demonstrates how to validate a Swagger file and log whether the API is valid or not.
const SwaggerParser = require('swagger-parser');
SwaggerParser.validate('path/to/your/swagger.yaml')
.then(api => {
console.log('API is valid:', api);
})
.catch(err => {
console.error('API is invalid:', err);
});
Dereference
This feature allows you to dereference $ref pointers in a Swagger (OpenAPI) definition file. The code sample demonstrates how to dereference a Swagger file and log the dereferenced API.
const SwaggerParser = require('swagger-parser');
SwaggerParser.dereference('path/to/your/swagger.yaml')
.then(api => {
console.log('Dereferenced API:', api);
})
.catch(err => {
console.error(err);
});
Bundle
This feature allows you to bundle all external $ref pointers into a single file. The code sample demonstrates how to bundle a Swagger file and log the bundled API.
const SwaggerParser = require('swagger-parser');
SwaggerParser.bundle('path/to/your/swagger.yaml')
.then(api => {
console.log('Bundled API:', api);
})
.catch(err => {
console.error(err);
});
openapi-schema-validator is a package that validates OpenAPI 3.0 schemas. It focuses on schema validation and does not provide parsing or dereferencing functionalities like swagger-parser.
swagger-jsdoc is a package that generates Swagger (OpenAPI) definitions from JSDoc comments in your code. It is more focused on generating documentation from code comments rather than parsing and validating existing Swagger files.
swagger-client is a package that provides a JavaScript client for interacting with Swagger (OpenAPI) APIs. It includes functionalities for making API requests and handling responses, but it does not focus on parsing or validating Swagger definitions.
swagger.parser.parse("path/to/my/swagger.yaml", function(err, swagger) {
if (err) {
console.error("There's an error in the Swagger file: " + err.message);
return;
}
console.log("Your API is " + swagger.info.title + ", version " + swagger.info.version);
});
The swagger
parameter that is passed to the callback function is a fully-parsed, validated, and dereferenced Swagger Object.
npm install swagger-parser
Then add this to your Node script:
var parser = require("swagger-parser");
parser.parse('path/to/my/swagger.yaml', function(err, swagger) { ... });
bower install swagger-parser
Then add this to your HTML page:
<script src="bower_components/swagger-parser/dist/swagger-parser.js"></script>
<script>
swagger.parser.parse('http://mysite.com/path/to/my/swagger.yaml', function(err, swagger) { ... });
</script>
Just add swagger-parser
to your AMD module's dependencies, or require("swagger-parser")
explicitly.
define("myModule", ["swagger-parser"], function(parser) {
parser.parse('http://mysite.com/path/to/my/swagger.yaml', function(err, swagger) { ... });
});
The parse
function accepts an optional options
parameter, like this:
var options = {
dereferencePointers: false,
validateSpec: false
};
parser.parse("path/to/my/swagger.yaml", options, function(err, swaggerObject) {
...
});
Available options are as follows:
parseYaml (default: true) -
Determines whether the parser will allow Swagger specs in YAML format. If set to false
, then only JSON will be allowed.
dereferencePointers (default: true) -
Determines whether $ref
pointers will be dereferenced. If set to false
, then the resulting SwaggerObject will contain Reference Objects instead of the objects they reference.
dereferenceExternalPointers (default: true) -
Determines whether $ref
pointers will be dereferenced if they point to external files (e.g. "http://company.com/my/schema.yaml"). If set to false
then the resulting SwaggerObject will contain Reference Objects for external pointers instead of the objects they reference.
validateSpec (default: true) -
Determines whether your Swagger spec will be validated against the official Swagger schema. If set to false
, then the resulting Swagger Object may be missing properties, have properties of the wrong data type, etc.
I welcome any contributions, enhancements, and bug-fixes. File an issue on GitHub and submit a pull request. Use JSHint to make sure your code passes muster. (see .jshintrc).
Here are some things currently on the to-do list:
$ref
pointers are not currently supported. So something like this won't work:person:
properties:
name:
type: string
spouse:
type:
$ref: person
Swagger-Parser is 100% free and open-source, under the MIT license. Use it however you want.
FAQs
Swagger 2.0 and OpenAPI 3.0 parser and validator for Node and browsers
The npm package swagger-parser receives a total of 908,055 weekly downloads. As such, swagger-parser popularity was classified as popular.
We found that swagger-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.