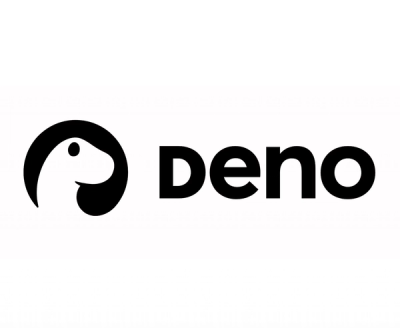
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
synapsefi-dev-js-api-wrapper
Advanced tools
## Installation ```sh npm installation synapsefi-ui axios lodash ```
npm installation synapsefi-ui axios lodash
synapsefi-js-api-wrapper
was built to simplify api requests to synapsefi's core public apis.
ApiWrapper generates instance of apiWrapper. We decide to name an instance as apiWrapper because all it does is firing api calls. User of this library can declare variable with diffrent naming convention.
import ApiWrapper from 'synapsefi-js-api-wrapper'; // for client
// const ApiWrapper = require('synapsefi-js-api-wrapper'); // for node
const platformUserApiWrapper = new ApiWrapper({
host: 'sandbox or production host(ex: https://uat-api.synapsefi.com)',
client_id: '<clinet id>',
client_secret: '<clinet secret>',
fingerprint: '<fingerprint>',
ip_address: '<user_id> of platform',
oauth_key: '<oauth_key>',
user_id: '<user_id> of platform',
refresh_token: '<refresh_token> of platform',
});
const endUserApiWrapper = new ApiWrapper({
host: platformUserApiWrapper.host,
client_id: platformUserApiWrapper.client_id,
client_secret: platformUserApiWrapper.client_secret,
ip_address: platformUserApiWrapper.ip_address,
user_id: '<user_id> of the end user',
fingerprint: '<finger print when this user is created>',
refresh_token: '<refresh_token> of the end user',
oauth_key: '<oauth_key> of the end user',
});
with no argument
platformUserApiWrapper.GET_ALL_CLIENT_USERS().then(({data}) => {
console.log('data: ', data);
});
search by name or email using query
platformUserApiWrapper.GET_ALL_CLIENT_USERS({ query: 'John Doe' }).then(({data}) => {
console.log('data: ', data);
});
specific page and per page (page, per_page)
platformUserApiWrapper
.GET_ALL_CLIENT_USERS({
page: 2,
per_page: 3,
})
.then(({ data }) => {
console.log('data: ', data);
});
conbining query, page, and per_page
platformUserApiWrapper
.GET_ALL_CLIENT_USERS({
query: 'sean@gmail.com',
page: 1,
per_page: 2,
})
.then(({ data }) => {
console.log('data: ', data);
});
platformUserApiWrapper
.POST_CREATE_USER({
logins: [{ email: 'email@email.com' }],
phone_numbers: ['123.123.1233'],
legal_names: ['John Doe'],
})
.then(({ data }) => {
console.log('data: ', data);
});
platformUserApiWrapper.GET_USER().then(({ data }) => {
console.log('data: ', data);
});
const personalDocuments = [{
email: 'personal@email.com',
phone_number: '1231231233',
ip: '127.0.0.1',
name: 'Personal Name',
alias: 'Test',
entity_type: 'M',
entity_scope: 'Arts & Entertainment',
day: 2,
month: 5,
year: 1989,
address_street: '101 2nd St',
address_city: 'SF',
address_subdivision: 'CA',
address_postal_code: '94105',
address_country_code: 'US',
social_docs: [
{
document_value: 'https://www.facebook.com/validasdf',
document_type: 'FACEBOOK',
},
],
}];
platformUserApiWrapper
.PATCH_ADD_NEW_DOCUMENTS({
documents: personalDocuments,
})
.then(({ data }) => {
console.log('data: ', data);
});
update base doc
platformUserApiWrapper
.PATCH_UPDATE_DOCUMENTS([{
documents: {
id: '<initialBaseDocId>',
email: 'updated@gmail.com',
},
}])
.then(({ data }) => {
console.log('data: ', data);
});
update sub docs
platformUserApiWrapper
.PATCH_UPDATE_DOCUMENTS({
documents: [{
id: '<initialBaseDocId>',
social_docs: [
{
id: '<facebookDocId>',
document_value: 'https://www.facebook.com/afterUpdate',
document_type: 'FACEBOOK',
},
],
},
}])
.then(({ data }) => {
console.log('data: ', data);
});
platformUserApiWrapper
.PATCH_DELETE_BASE_DOC({ baseDocId: '<document id of base doc>' })
.then(({ data }) => {
console.log('data: ', data);
});
platformUserApiWrapper
.PATCH_DELETE_SUB_DOCS({
baseDocId: '<base_doc_id>',
socialDocIds: [
'<social_doc_id 1>',
'<social_doc_id 2>',
'fda60784d6375bc44eda...',
'28d9177b22c127d9a51d...',
],
})
.then(({ data }) => {
console.log('data: ', data);
});
update legal name, login email, password, and phone number
platformUserApiWrapper
.PATCH_UPDATE_USER({
updateObj: {
legal_name: 'After User',
login: { email: 'after@email.com' },
phone_number: '9879879877',
remove_legal_name: 'Before User',
remove_login: { email: 'before@email.com' },
remove_phone_number: '1231231233',
},
})
.then(({ data }) => {
console.log('data: ', data);
});
update cip_tag
platformUserApiWrapper
.PATCH_UPDATE_USER({
updateObj: {
legal_name: 'After User',
cip_tag: 2,
// public_note: 'Eask just updated public note ~~~',
},
})
.then(({ data }) => {
console.log('data: ', data);
});
If you include public note then cip tag will not be updated (bug ?)
lock user
endUserApiWrapper
.PATCH_USER_PERMISSION({
permission: 'LOCKED',
})
.then(({ data }) => {
console.log('data: ', data);
});
delete user
endUserApiWrapper
.PATCH_USER_PERMISSION({
permission: 'MAKE-IT-GO-AWAY',
})
.then(({ data }) => {
console.log('data: ', data);
});
platformUserApiWrapper.oauth_key = 'fake oauth key';
console.log('platformUserApiWrapper.oauth_key: ', platformUserApiWrapper.oauth_key); // fake oauth key
platformUserApiWrapper.POST_OAUTH_USER().then(({ data }) => {
console.log('data: ', data);
});
// Calling "POST_OAUTH_USER" set new oauth_key to platformUserApiWrapper.
// platformUserApiWrapper.oauth_key === data.oauth_key
console.log('platformUserApiWrapper.oauth_key: ', platformUserApiWrapper.oauth_key); // data.oauth_key
FAQs
## Installation ```sh npm installation synapsefi-ui axios lodash ```
The npm package synapsefi-dev-js-api-wrapper receives a total of 2 weekly downloads. As such, synapsefi-dev-js-api-wrapper popularity was classified as not popular.
We found that synapsefi-dev-js-api-wrapper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.