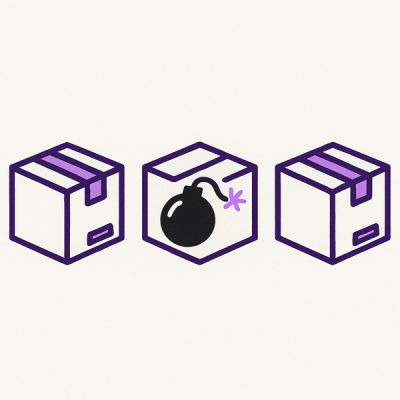
Research
Security News
The Landscape of Malicious Open Source Packages: 2025 Mid‑Year Threat Report
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
testcontainers
Advanced tools
Testcontainers is a NodeJS library that supports tests, providing lightweight, throwaway instances of common databases, Selenium web browsers, or anything else that can run in a Docker container
Supply Chain Security
Vulnerability
Quality
Maintenance
License
Network access
Supply chain riskThis module accesses the network.
Found 1 instance in 1 package
Shell access
Supply chain riskThis module accesses the system shell. Accessing the system shell increases the risk of executing arbitrary code.
Found 1 instance in 1 package
Socket optimized override available
The testcontainers npm package is a library that provides lightweight, throwaway instances of common databases, Selenium web browsers, or anything else that can run in a Docker container. It is primarily used for integration testing, allowing developers to run tests against real instances of services without the overhead of managing those services manually.
Running a PostgreSQL container
This feature allows you to run a PostgreSQL container for testing purposes. The code sample demonstrates how to start a PostgreSQL container with a specified password and expose the default port.
const { GenericContainer } = require('testcontainers');
(async () => {
const container = await new GenericContainer('postgres')
.withEnv('POSTGRES_PASSWORD', 'password')
.withExposedPorts(5432)
.start();
console.log(`PostgreSQL started on port ${container.getMappedPort(5432)}`);
})();
Running a Redis container
This feature allows you to run a Redis container for testing purposes. The code sample demonstrates how to start a Redis container and expose the default port.
const { GenericContainer } = require('testcontainers');
(async () => {
const container = await new GenericContainer('redis')
.withExposedPorts(6379)
.start();
console.log(`Redis started on port ${container.getMappedPort(6379)}`);
})();
Running a custom Docker container
This feature allows you to run any custom Docker container for testing purposes. The code sample demonstrates how to start a custom Docker container and expose a specified port.
const { GenericContainer } = require('testcontainers');
(async () => {
const container = await new GenericContainer('your-custom-image')
.withExposedPorts(8080)
.start();
console.log(`Custom container started on port ${container.getMappedPort(8080)}`);
})();
Dockerode is a Docker client for Node.js. It provides a way to interact with Docker containers programmatically. While it offers more control and flexibility over Docker operations, it lacks the high-level abstractions and ease of use that testcontainers provides for integration testing.
Node-docker-api is another Docker client for Node.js. Similar to dockerode, it allows for programmatic interaction with Docker containers. However, it does not provide the same level of convenience and specialized features for testing as testcontainers.
See LICENSE.
Copyright (c) 2018 - 2023 Cristian Greco and other authors.
See CONTRIBUTING.md for how to contribute to this repo.
See contributors for all contributors.
Join our Slack workspace | Testcontainers OSS | Testcontainers Cloud
FAQs
Testcontainers is a NodeJS library that supports tests, providing lightweight, throwaway instances of common databases, Selenium web browsers, or anything else that can run in a Docker container
The npm package testcontainers receives a total of 630,190 weekly downloads. As such, testcontainers popularity was classified as popular.
We found that testcontainers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.