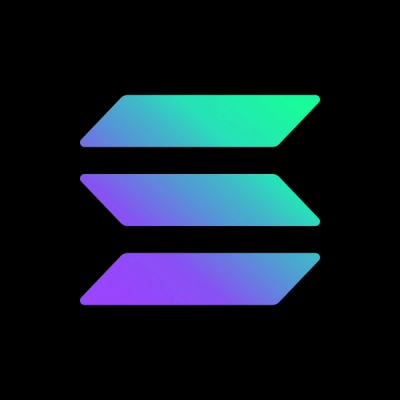
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
This module is written in TypeScript with the type declaration files included. Provides an easy-to-use interface to request information from the IGDB API. To use this API you will need to register at https://api.igdb.com/.
The module can be easily installed via node package manager
npm install ts-igdb --save
The package includes the type declaration files for TypeScript; you don't need to install them separately.
First, you have to set up an options object which will be used to construct the query url against IGDB.
When the object is ready, you can call the module's specific endpoint method, passing this object as a parameter to it. These methods will always return a promise.
Javascript example:
// index.js
const IGDB = require('ts-igdb').default;
const igdb = new IGDB('<YOUR API KEY>');
// Fetch data using the GAME endpoint
igdb.endpoint.game({
search : 'uncharted 4', // searching for a game
fields: ['id', 'name', 'cover'], // return these fields
limit: 5, // set the maximum number of results
order: { // set the ordering
field: 'name', // by the name field
direction: 'asc' // in ascending order
}
})
.then(
games => {
// 'games' will contain all results as an array
console.log(games);
}
)
.catch(
error => {
// in case of an error, the error object will be returned
console.log(error.message);
}
)
Typescript example:
// index.ts
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
search : 'uncharted 4', // searching for a game
fields: ['id', 'name', 'cover'], // return these fields
limit: 5, // set the maximum number of results
order: { // set the ordering
field: 'name', // by the name field
direction: 'asc' // in ascending order
}
}
igdb.endpoint.game(options)
.then(
(games: GameEndpointResponse[]) => {
// 'games' will contain all results as an array
console.log(games);
}
)
.catch(
(error: any) => {
// in case of an error, the error object will be returned
console.log(error.message);
}
)
Every endpoint method will expect an options
object which will set the request's parameters. The object can contain the following keys:
id [optional]
: One ore more ID's as an array. When ID is provided, the search parameter will be ignored.search [optional]
: The query will search the name field looking for this value as a string. If id is provided in the same options object, than this value will be ignored.fields [optional]
: The fields you want to see in the result array. If every field is required an array with a single star element can be set ( search: ['*']
). If not provided only the id field will be returned.limit [optional]
: The maximum number of results in a single query. This value must be a number between 1 and 50. If not provided, IGDB's default value will be used (at the time of writing the default limit is 10)offset [optional]
: This will start the result list at the provided value and will give limit number of results. This value must be a number (0 or greater). By default the results will be returned from the first element (0).expand [optional]
: The expander feature is used to combine multiple requests. Have to be provided as an array of strings.filters [optional]
: Filters are used to swift through results to get what you want. You can exclude and include results based on their properties. The parameter have to be provided as an array. The elements have to be objects with the following parameters (these are not optional, all three parameters are required):
field
: The name of the field you want to apply the filter topostfix
: The postfix you want to use with the filter. The values can be
eq
: Equal: Exact match equal.not_eq
: Not Equal: Exact match equal.gt
: Greater than works only on numbers.gte
: Greater than or equal to works only on numbers.lt
: Less than works only on numbers.lte
: Less than or equal to works only on numbers.prefix
: Prefix of a value only works on strings.exists
: The value is not null.not_exists
: The value is null.in
: The value exists within the (comma separated) array (AND between values).not_in
: The values must not exists within the (comma separated) array (AND between values).any
: The value has any within the (comma separated) array (OR between values).value
: The value of the filter. This can be a string, a number or an array.order [optional]
: Ordering (sorting) is used to order results by a specific field. When not provided, the results will be ordered ASCENDING by ID. This have to be an object with the following parameters:
field
: The field you want to do the ordering by as a stringdirection
: The direction of the ordering. This can have one of the following values:
asc
: ascending orderingdesc
: descending orderingsubfilter [optional]
: You can apply this optional subfilter for even more complex ordering. Available subfilters are:
min
max
avg
sum
median
count [optional]
: This is a boolean value whether you want to get the results, or the sum of all the records that match the provided filters. In case of boolean TRUE only a single object will be returned with a count
property, containing the number of the matched results. If count is provided in the options object, only the filters
parameter will be processed (if presents). If no filter is passed, everything on that endpoint will be counted.Data can be requested from most of the IGDB endpoints. For more information on the returned data can be found in the IGDB Endpoint documentation
Every endpoint method expects an options
object to set up the request and returns a Promise
. In case of a successful request the Promise
will resolve the results as an array. If the request failed it will reject the Got module's error object.
IGDB.endpoint.achievements(options:
IGDBOptions
): Promise<
AchievementsEndpointResponse
[]>
For more information check the IGDB Achievements Endpoint Documentation.
IGDB.endpoint.character(options:
IGDBOptions
): Promise<
CharacterEndpointResponse
[]>
For more information check the IGDB Character Endpoint Documentation.
IGDB.endpoint.collection(options:
IGDBOptions
): Promise<
CollectionEndpointResponse
[]>
For more information check the IGDB Collection Endpoint Documentation.
IGDB.endpoint.company(options:
IGDBOptions
): Promise<
CompanyEndpointResponse
[]>
For more information check the IGDB Company Endpoint Documentation.
IGDB.endpoint.credit(options:
IGDBOptions
): Promise<
CreditEndpointResponse
[]>
For more information check the IGDB Credit Endpoint Documentation.
IGDB.endpoint.externalReview(options:
IGDBOptions
): Promise<
ExternalReviewEndpointResponse
[]>
For more information check the IGDB External Review Endpoint Documentation.
IGDB.endpoint.externalReviewSource(options:
IGDBOptions
): Promise<
ExternalReviewSourceEndpointResponse
[]>
For more information check the IGDB External Review Source Endpoint Documentation.
IGDB.endpoint.feed(options:
IGDBOptions
): Promise<
FeedEndpointResponse
[]>
For more information check the IGDB Feed Endpoint Documentation.
IGDB.endpoint.franchise(options:
IGDBOptions
): Promise<
FranchiseEndpointResponse
[]>
For more information check the IGDB Franchise Endpoint Documentation.
IGDB.endpoint.game(options:
IGDBOptions
): Promise<
GameEndpointResponse
[]>
For more information check the IGDB Game Endpoint Documentation.
IGDB.endpoint.gameEngine(options:
IGDBOptions
): Promise<
GameEngineEndpointResponse
[]>
For more information check the IGDB Game Engine Endpoint Documentation.
IGDB.endpoint.gameMode(options:
IGDBOptions
): Promise<
GameModeEndpointResponse
[]>
For more information check the IGDB Game Mode Endpoint Documentation.
IGDB.endpoint.gameVersion(options:
IGDBOptions
): Promise<
GameVersionEndpointResponse
[]>
For more information check the IGDB Game Version Endpoint Documentation.
IGDB.endpoint.genre(options:
IGDBOptions
): Promise<
GenreEndpointResponse
[]>
For more information check the IGDB Genre Endpoint Documentation.
IGDB.endpoint.keyword(options:
IGDBOptions
): Promise<
KeywordEndpointResponse
[]>
For more information check the IGDB Keyword Endpoint Documentation.
IGDB.endpoint.page(options:
IGDBOptions
): Promise<
PageEndpointResponse
[]>
For more information check the IGDB Page Endpoint Documentation.
IGDB.endpoint.person(options:
IGDBOptions
): Promise<
PersonEndpointResponse
[]>
For more information check the IGDB Person Endpoint Documentation.
IGDB.endpoint.platform(options:
IGDBOptions
): Promise<
PlatformEndpointResponse
[]>
For more information check the IGDB Platform Endpoint Documentation.
IGDB.endpoint.playerPerspecitve(options:
IGDBOptions
): Promise<
PlayerPerspecitveEndpointResponse
[]>
For more information check the IGDB Player Perspecitve Endpoint Documentation.
IGDB.endpoint.pulse(options:
IGDBOptions
): Promise<
PulseEndpointResponse
[]>
For more information check the IGDB Pulse Endpoint Documentation.
IGDB.endpoint.pulseGroup(options:
IGDBOptions
): Promise<
PulseGroupEndpointResponse
[]>
For more information check the IGDB Pulse Group Endpoint Documentation.
IGDB.endpoint.pulseSource(options:
IGDBOptions
): Promise<
PulseSourceEndpointResponse
[]>
For more information check the IGDB Pulse Source Endpoint Documentation.
IGDB.endpoint.releaseDate(options:
IGDBOptions
): Promise<
ReleaseDateEndpointResponse
[]>
For more information check the IGDB Release Date Endpoint Documentation.
IGDB.endpoint.review(options:
IGDBOptions
): Promise<
ReviewEndpointResponse
[]>
For more information check the IGDB Review Endpoint Documentation.
IGDB.endpoint.theme(options:
IGDBOptions
): Promise<
ThemeEndpointResponse
[]>
For more information check the IGDB Theme Endpoint Documentation.
IGDB.endpoint.title(options:
IGDBOptions
): Promise<
TitleEndpointResponse
[]>
For more information check the IGDB Title Endpoint Documentation.
IGDB.endpoint.userProfile(options:
IGDBOptions
): Promise<
UserProfileEndpointResponse
[]>
For more information check the IGDB User Profile Endpoint Documentation.
This is an URL helper method. Full or partial URL's can be retrieved.
IGDB.url.queryString(options: IGDBOptions): string
This method expects an options
object and returns the parsed query string with the parameters in it as a string.
const options = {
search : 'uncharted 4', // searching for a game
fields: ['id', 'name', 'cover'], // return these fields
limit: 5, // set the maximum number of results
order: { // set the ordering
field: 'name', // by the name field
direction: 'asc' // in ascending order
}
};
const queryString = igdb.url.queryString(options);
console.log(queryString);
Output:
?search=uncharted%204&fields=id,name,cover&limit=5&order=name:asc
IGDB.url.apiUrl(): string
This method will return the IGDB API's URL
const url = igdb.url.apiUrl();
console.log(url);
Output:
https://api-endpoint.igdb.com
IGDB.url.queryUrL(endpoint: string, options: IGDBOptions): string
This method will return the full query url. Expects two parameters:
endpoint
: The endpoint to send the request tooptions
: An options objectReturns a string with the full url
const options = {
search : 'uncharted 4', // searching for a game
fields: ['id', 'name', 'cover'], // return these fields
limit: 5, // set the maximum number of results
order: { // set the ordering
field: 'name', // by the name field
direction: 'asc' // in ascending order
}
};
const queryUrl = igdb.url.queryUrl('game', options);
console.log(queryUrl);
Output:
https://api-endpoint.igdb.com/game/?search=uncharted%204&fields=id,name,cover&limit=5&order=name:asc
IGDB.url.image(image: Image, size: string): string
The method helps convert the Image objects from the results to an URL to the image. Expects two parameters:
image
: an image object from the resultssize
: The image size. These values can be the following:
cover_small
: Fit (90 x 128)cover_small_2x
: Fit (retina DPR 2.0 size)screenshot_med
: Lfill, Center gravity (569 x 320)screenshot_med_2x
: Lfill, Center gravity (retina DPR 2.0 size)cover_big
: Fit (264 x 374)cover_big_2x
: Fit (retina DPR 2.0 size)logo_med
: Fit (284 x 160)logo_med_2x
: Fit (retina DPR 2.0 size)screenshot_big
: Lfill, Center gravity (889 x 500)screenshot_big_2x
: Lfill, Center gravity (retina DPR 2.0 size)screenshot_huge
: Lfill, Center gravity (1280 x 720)screenshot_huge_2x
: Lfill, Center gravity (retina DPR 2.0 size)thumb
: Thumb, Center gravity (90 x 90)thumb_2x
: Thumb, Center gravity (retina DPR 2.0 size)micro
: Thumb, Center gravity (35 x 35)micro_2x
: Thumb, Center gravity (retina DPR 2.0 size)720p
: Fit, Center gravity (1280 x 720)720p_2x
: Fit, Center gravity (retina DPR 2.0 size)1080p
: Fit, Center gravity (1920 x 1080)1080p_2x
: Fit, Center gravity (retina DPR 2.0 size).then(
games => {
console.log(games[0].cover);
/*
Output of the first game's cover object:
{
url: '//images.igdb.com/igdb/image/upload/t_thumb/g7ty6o78s8dp3lclojxy.jpg',
cloudinary_id: 'g7ty6o78s8dp3lclojxy',
width: 460,
height: 215
}
*/
// Passing the first game's cover object
const coverUrl = igdb.url.image(games[0].cover, 'screenshot_med');
console.log(coverUrl);
}
)
Output:
https://images.igdb.com/igdb/image/upload/g7ty6o78s8dp3lclojxy/screenshot_med.png
According to the IGDB Image Documentation only the url parameter of the Image object is mandatory which can be a non-IGDB url. If the cloudinary_id
is present in the result, the url will be returned by the passed size. If only the url exists in the object, then this url is returned.
The following interfaces can be imported when the module is used in a TypeScript project:
import { <INTERFACES> } from 'ts-igdb/interface/igdb';
IGDBOptions can be imported from here which is declaring the type of the options object. It is enough to import only the IGDBOptions interface, since FilterOption and OrderOption is part of the IGDB object. However you can import them if required.
import { <INTERFACE> } from 'ts-igdb/interface/endpoint-response';
Every endpoint will return an array with elements from the related type. These interfaces can be imported from interface/endpoint-response.
import { <INTERFACE> } from 'ts-igdb/interface/miscellaneous';
Miscellaneous objects are entities which don’t have individual IDs and are only present in fields either as an object or as an array of objects.
For more information check the IGDB Miscellaneous Objects Documentation
These examples are available in the examples folder of this project on GitHub. The examples are taken from IGDB's example page.
/*
Get all information from a specific game
/games/1942?fields=*
1942, is the ID of the game.
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOU API KEY>');
const options: IGDBOptions = {
id: [1942],
fields: ['*']
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Get all games from specific genres
/genres/12,9,11?fields=*
Notice you can comma separate multiple IDs (12, 9 and 11). You can do this with games, companies and anything else.
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GenreEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
id: [12, 9, 11],
fields: ['*']
};
igdb.endpoint.genre(options)
.then(
(response: GenreEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Count total games that have a rating higher than 75
/games/count?filter[rating][gt]=75
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
count: true,
filters: [
{
field: 'rating',
postfix: 'gt',
value: 75
}
]
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
// When using count, the response will be an array with one element with only one property: count
console.log(response[0].count);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Count total games from a certain platform (Playstation 4 , id=48)
/games/count?filter[release_dates.platform][eq]=48
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
count: true,
filters: [
{
field: 'release_dates.platform',
postfix: 'eq',
value: 48
}
]
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
// When using count, the response will be an array with one element with only one property: count
console.log(response[0].count);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Order by popularity
Popularity parameter for games. You can access it like this: /games/?fields=name,popularity&order=popularity:desc
The popularity number is calculated using usage statistics of game pages at https://www.igdb.com
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
fields: ['name', 'popularity'],
order: {
field: 'popularity',
direction: 'desc'
}
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Coming soon games for Playstation 4
/release_dates/?fields=*&filter[platform][eq]=48&order=date:asc&filter[date][gt]=1500619813000&expand=game
1500619813000: Is the timestamp in milliseconds of today (This you need to generate yourself) 48 Is the platform id of Playstation 4.
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { ReleaseDateEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
fields: ['*'],
filters: [
{
field: 'platform',
postfix: 'eq',
value: 48
},
{
field: 'date',
postfix: 'gt',
value: new Date().getTime()
}
],
order: {
field: 'date',
direction: 'asc'
},
expand: ['game']
};
igdb.endpoint.release_date(options)
.then(
(response: ReleaseDateEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Search, return certain fields.
/games/?search=Halo
This will return search results and the IDs of the games. If you want to return certain fields of the game or even all, do the following:
/games/?search=Halo&fields=name,publishers /games/?search=Halo&fields=*
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
search: 'halo',
fields: ['*']
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Search games but exclude versions (editions)
/games/?search=Assassins%20Creed&fields=name&filter[version_parent][not_exists]=1
This will return search results with ID and name of the game but exclude editions such as “Collectors Edition”.
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
search: 'Assassins Creed',
fields: ['name'],
filters: [
{
field: 'version_parent',
postfix: 'not_exists',
value: 1
}
]
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Get versions (editions) of a game
/game_versions/?fields=games,games.name&filter[game][eq]=28540&expand=games
This resulting object will contain a list of version ids and names.
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameVersionEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
fields: ['games', 'games.name'],
filters: [
{
field: 'game',
postfix: 'eq',
value: 28540
}
],
expand: ['games']
};
igdb.endpoint.game_version(options)
.then(
(response: GameVersionEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
/*
Get the parent game for a version
/games/39047?fields=version_parent
This resulting object will contain the id of the parent game (version_parent).
*/
import IGDB from 'ts-igdb';
import { IGDBOptions } from 'ts-igdb/interface/igdb';
import { GameEndpointResponse } from 'ts-igdb/interface/endpoint-response';
const igdb = new IGDB('<YOUR API KEY>');
const options: IGDBOptions = {
id: [39047],
fields: ['version_parent']
};
igdb.endpoint.game(options)
.then(
(response: GameEndpointResponse[]) => {
console.log(response);
}
)
.catch(
(error: any) => console.log(error.message)
)
FAQs
Node.js module for IGDB written in TypeScript
The npm package ts-igdb receives a total of 2 weekly downloads. As such, ts-igdb popularity was classified as not popular.
We found that ts-igdb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.