ts-pattern
Advanced tools
Comparing version 5.0.0-rc.1 to 5.0.0-rc.2
@@ -537,3 +537,3 @@ import * as symbols from './internals/symbols.js'; | ||
* | ||
* [Read documentation for `P.bigint.between` on GitHub](https://github.com/gvergnaud/ts-pattern#Pbigintbetween) | ||
* [Read documentation for `P.bigint.between` on GitHub](https://github.com/gvergnaud/ts-pattern#Pnumberbetween) | ||
* | ||
@@ -610,3 +610,3 @@ * @example | ||
* | ||
* [Read documentation for `P.bigint.between` on GitHub](https://github.com/gvergnaud/ts-pattern#Pbigintbetween) | ||
* [Read documentation for `P.bigint.between` on GitHub](https://github.com/gvergnaud/ts-pattern#Pnumberbetween) | ||
* | ||
@@ -651,3 +651,3 @@ * @example | ||
* | ||
* [Read documentation for `P.bigint.gte` on GitHub](https://github.com/gvergnaud/ts-pattern#Pbigintgte) | ||
* [Read documentation for `P.bigint.gte` on GitHub](https://github.com/gvergnaud/ts-pattern#Pnumbergte) | ||
* | ||
@@ -662,3 +662,3 @@ * @example | ||
* | ||
* [Read documentation for `P.bigint.positive` on GitHub](https://github.com/gvergnaud/ts-pattern#Pbigintpositive) | ||
* [Read documentation for `P.bigint.positive` on GitHub](https://github.com/gvergnaud/ts-pattern#Pnumberpositive) | ||
* | ||
@@ -673,3 +673,3 @@ * @example | ||
* | ||
* [Read documentation for `P.bigint.negative` on GitHub](https://github.com/gvergnaud/ts-pattern#Pbigintnegative) | ||
* [Read documentation for `P.bigint.negative` on GitHub](https://github.com/gvergnaud/ts-pattern#Pnumbernegative) | ||
* | ||
@@ -676,0 +676,0 @@ * @example |
{ | ||
"name": "ts-pattern", | ||
"version": "5.0.0-rc.1", | ||
"version": "5.0.0-rc.2", | ||
"description": " The exhaustive Pattern Matching library for TypeScript.", | ||
@@ -61,10 +61,10 @@ "type": "module", | ||
"devDependencies": { | ||
"@types/jest": "^27.0.2", | ||
"jest": "^27.4.5", | ||
"@types/jest": "^29.5.2", | ||
"jest": "^29.5.0", | ||
"microbundle": "^0.15.1", | ||
"prettier": "^2.8.8", | ||
"rimraf": "^5.0.0", | ||
"ts-jest": "^27.1.2", | ||
"typescript": "^5.0.4" | ||
"rimraf": "^5.0.1", | ||
"ts-jest": "^29.1.0", | ||
"typescript": "^5.1.3" | ||
} | ||
} |
348
README.md
@@ -20,4 +20,2 @@ <h1 align="center">TS-Pattern</h1> | ||
```ts | ||
@@ -48,2 +46,3 @@ import { match, P } from 'ts-pattern'; | ||
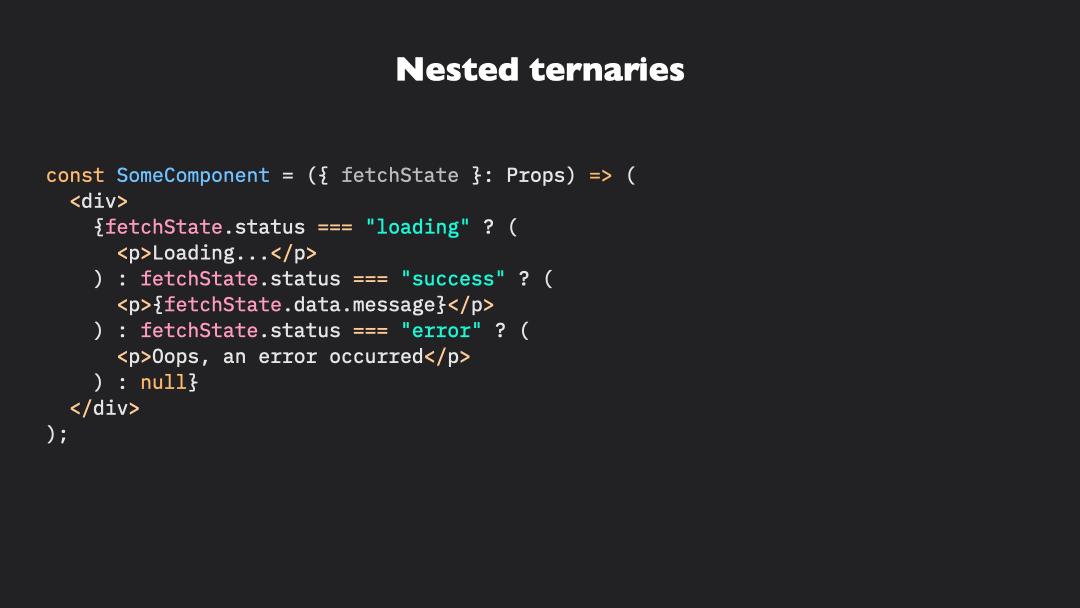 | ||
<p align="center"><i>Animation by <a target="_blank" href="https://twitter.com/nicoespeon/status/1644342570389061634?s=20">@nicoespeon</a></i></p> | ||
@@ -88,10 +87,9 @@ | ||
| ts-pattern | TypeScript v4.5+ | TypeScript v4.2+ | TypeScript v4.1+ | | ||
| --------------------------------------------------------------------------------------------------------------------------------------- | ---------------- | ---------------- | ---------------- | | ||
| v4.x [(Docs)](#documentation) [(Migration Guide)](https://github.com/gvergnaud/ts-pattern/tree/master/docs/v3-to-v4-migration-guide.md) | ✅ | ❌ | ❌ | | ||
| v3.x [(Docs)](https://github.com/gvergnaud/ts-pattern/tree/v3#documentation) | ✅ | ✅ | ⚠️ | | ||
| v2.x [(Docs)](https://github.com/gvergnaud/ts-pattern/tree/v2#documentation) | ✅ | ✅ | ✅ | | ||
| ts-pattern | TypeScript v5+ | TypeScript v4.5+ | TypeScript v4.2+ | | ||
| --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------- | ---------------- | ---------------- | | ||
| v5.x [(Docs)](#documentation) [(Migration Guide)](https://github.com/gvergnaud/ts-pattern/tree/master/docs/v4-to-v5-migration-guide.md) | ✅ | ❌ | ❌ | | ||
| v4.x [(Docs)](https://github.com/gvergnaud/ts-pattern/tree/v4##documentation) [(Migration Guide)](https://github.com/gvergnaud/ts-pattern/tree/master/docs/v3-to-v4-migration-guide.md) | ✅ | ✅ | ❌ | | ||
| v3.x [(Docs)](https://github.com/gvergnaud/ts-pattern/tree/v3#documentation) | ✅ | ✅ | ✅ | | ||
- ✅ Full support | ||
- ⚠️ Partial support, All features except passing multiple patterns to `.with()`. | ||
- ❌ Not supported | ||
@@ -747,2 +745,57 @@ | ||
### Objects | ||
Patterns can be objects containing sub-patterns. An object pattern will match | ||
If and only if the input value **is an object**, contains **all properties** the pattern defines | ||
and each property **matches** the corresponding sub-pattern. | ||
```ts | ||
import { match } from 'ts-pattern'; | ||
type Input = | ||
| { type: 'user'; name: string } | ||
| { type: 'image'; src: string } | ||
| { type: 'video'; seconds: number }; | ||
let input: Input = { type: 'user', name: 'Gabriel' }; | ||
const output = match(input) | ||
.with({ type: 'image' }, () => 'image') | ||
.with({ type: 'video', seconds: 10 }, () => 'video of 10 seconds.') | ||
.with({ type: 'user' }, ({ name }) => `user of name: ${name}`) | ||
.otherwise(() => 'something else'); | ||
console.log(output); | ||
// => 'user of name: Gabriel' | ||
``` | ||
### Tuples (arrays) | ||
In TypeScript, [Tuples](https://en.wikipedia.org/wiki/Tuple) are arrays with a fixed | ||
number of elements which can be of different types. You can pattern-match on tuples | ||
using a tuple pattern. A tuple pattern will match if the input value **is an array of the same length**, | ||
and each item match the corresponding sub-pattern. | ||
```ts | ||
import { match, P } from 'ts-pattern'; | ||
type Input = | ||
| [number, '+', number] | ||
| [number, '-', number] | ||
| [number, '*', number] | ||
| ['-', number]; | ||
const input: Input = [3, '*', 4]; | ||
const output = match(input) | ||
.with([P._, '+', P._], ([x, , y]) => x + y) | ||
.with([P._, '-', P._], ([x, , y]) => x - y) | ||
.with([P._, '*', P._], ([x, , y]) => x * y) | ||
.with(['-', P._], ([, x]) => -x) | ||
.otherwise(() => NaN); | ||
console.log(output); | ||
// => 12 | ||
``` | ||
### Wildcards | ||
@@ -881,57 +934,2 @@ | ||
### Objects | ||
Patterns can be objects containing sub-patterns. An object pattern will match | ||
If and only if the input value **is an object**, contains **all properties** the pattern defines | ||
and each property **matches** the corresponding sub-pattern. | ||
```ts | ||
import { match } from 'ts-pattern'; | ||
type Input = | ||
| { type: 'user'; name: string } | ||
| { type: 'image'; src: string } | ||
| { type: 'video'; seconds: number }; | ||
let input: Input = { type: 'user', name: 'Gabriel' }; | ||
const output = match(input) | ||
.with({ type: 'image' }, () => 'image') | ||
.with({ type: 'video', seconds: 10 }, () => 'video of 10 seconds.') | ||
.with({ type: 'user' }, ({ name }) => `user of name: ${name}`) | ||
.otherwise(() => 'something else'); | ||
console.log(output); | ||
// => 'user of name: Gabriel' | ||
``` | ||
### Tuples (arrays) | ||
In TypeScript, [Tuples](https://en.wikipedia.org/wiki/Tuple) are arrays with a fixed | ||
number of elements which can be of different types. You can pattern-match on tuples | ||
using a tuple pattern. A tuple pattern will match if the input value **is an array of the same length**, | ||
and each item match the corresponding sub-pattern. | ||
```ts | ||
import { match, P } from 'ts-pattern'; | ||
type Input = | ||
| [number, '+', number] | ||
| [number, '-', number] | ||
| [number, '*', number] | ||
| ['-', number]; | ||
const input: Input = [3, '*', 4]; | ||
const output = match(input) | ||
.with([P._, '+', P._], ([x, , y]) => x + y) | ||
.with([P._, '-', P._], ([x, , y]) => x - y) | ||
.with([P._, '*', P._], ([x, , y]) => x * y) | ||
.with(['-', P._], ([, x]) => -x) | ||
.otherwise(() => NaN); | ||
console.log(output); | ||
// => 12 | ||
``` | ||
### `P.array` patterns | ||
@@ -964,2 +962,23 @@ | ||
### Matching variadic tuples with `P.array` | ||
In TypeScript, [Variadic Tuple Types](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-4-0.html#variadic-tuple-types) are array types created with the `...` spread operator, like `[string, ...string[]]`, `[number, ...boolean[], string]` etc. You can match against variadic tuple types using array literals containing `...P.array(subpattern)`: | ||
```ts | ||
import { match, P } from 'ts-pattern'; | ||
type Input = (number | string)[]; | ||
declare const input: Input; | ||
const output = match(input) | ||
// P.array's parameter is optional | ||
.with([P.string, ...P.array()], (input) => input) // input: [string, ...(number | string)[]] | ||
.with(['print', ...P.array(P.string)], (input) => input) // input: ['print', ...string[]] | ||
// you can put patterns on either side of `...P.array()`: | ||
.with([...P.array(P.string), 'end'], (input) => input) // input: [...string[], 'end'] | ||
.with(['start', ...P.array(P.string), 'end'], (input) => input) // input: ['start', ...string[], 'end'] | ||
.otherwise((input) => input); | ||
``` | ||
### `P.set` patterns | ||
@@ -1258,2 +1277,205 @@ | ||
## `P.string` helper patterns | ||
`P.string` has a number of methods to help you match on specific strings. | ||
### `P.string.startsWith` | ||
`P.string.startsWith(str)` matches strings that start with the provided string. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.startsWith('TS'), () => '🎉') | ||
.otherwise(() => '❌'); | ||
console.log(fn('TS-Pattern')); // logs '🎉' | ||
``` | ||
### `P.string.endsWith` | ||
`P.string.endsWith(str)` matches strings that end with the provided string. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.endsWith('!'), () => '🎉') | ||
.otherwise(() => '❌'); | ||
console.log(fn('Hola!')); // logs '🎉' | ||
``` | ||
### `P.string.minLength` | ||
`P.string.minLength(min)` matches strings with at least `min` characters. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.minLength(2), () => '🎉') | ||
.otherwise(() => '❌'); | ||
console.log(fn('two')); // logs '🎉' | ||
``` | ||
### `P.string.maxLength` | ||
`P.string.maxLength(max)` matches strings with at most `max` characters. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.minLength(5), () => '🎉') | ||
.otherwise(() => 'too long'); | ||
console.log(fn('is this too long?')); // logs 'too long' | ||
``` | ||
### `P.string.includes` | ||
`P.string.includes(str)` matches strings that contain the provided substring. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.includes('!'), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn('Good job! 🎉')); // logs '✅' | ||
``` | ||
### `P.string.regex` | ||
`P.string.regex(RegExp)` matches strings if they match the provided regular expression. | ||
```ts | ||
const fn = (input: string) => | ||
match(input) | ||
.with(P.string.regex(/^[a-z]$/), () => 'single word') | ||
.otherwise(() => 'other strings'); | ||
console.log(fn('gabriel')); // logs 'single word' | ||
``` | ||
## `P.number` and `P.bigint` helper patterns | ||
`P.number` and `P.bigint` have several of methods to help you match on specific numbers and bigints. | ||
### `P.number.between` | ||
`P.number.between(min, max)` matches numbers between `min` and `max`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.between(1, 5), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(3), fn(1), fn(5), fn(7)); // logs '✅ ✅ ✅ ❌' | ||
``` | ||
### `P.number.lt` | ||
`P.number.lt(max)` matches numbers smaller than `max`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.lt(7), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(2), fn(7)); // logs '✅ ❌' | ||
``` | ||
### `P.number.gt` | ||
`P.number.gt(min)` matches numbers greater than `min`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.gt(7), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(12), fn(7)); // logs '✅ ❌' | ||
``` | ||
### `P.number.lte` | ||
`P.number.lte(max)` matches numbers smaller than or equal to `max`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.lte(7), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(7), fn(12)); // logs '✅ ❌' | ||
``` | ||
### `P.number.gte` | ||
`P.number.gte(min)` matches numbers greater than or equal to `min`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.gte(7), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(7), fn(2)); // logs '✅ ❌' | ||
``` | ||
### `P.number.int` | ||
`P.number.int()` matches integers. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.int(), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(12), fn(-3.141592)); // logs '✅ ❌' | ||
``` | ||
### `P.number.finite` | ||
`P.number.finite()` matches all numbers except `Infinity` and `-Infinity`. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.finite(), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(-3.141592), fn(Infinity)); // logs '✅ ❌' | ||
``` | ||
### `P.number.positive` | ||
`P.number.positive()` matches positive numbers. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.positive(), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(7), fn(-3.141592)); // logs '✅ ❌' | ||
``` | ||
### `P.number.negative` | ||
`P.number.negative()` matches negative numbers. | ||
```ts | ||
const fn = (input: number) => | ||
match(input) | ||
.with(P.number.negative(), () => '✅') | ||
.otherwise(() => '❌'); | ||
console.log(fn(-3.141592), fn(7)); // logs '✅ ❌' | ||
``` | ||
## Types | ||
@@ -1260,0 +1482,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
471619
1610