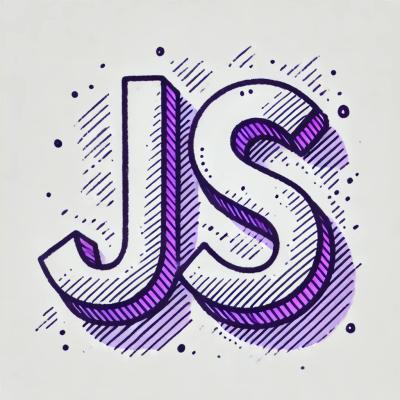
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
The tslib package is a runtime library for TypeScript that includes helper functions used by TypeScript emitted code when using various advanced features of the language such as async/await, for...of, spread, rest, and decorators. It is intended to be used with TypeScript's `importHelpers` flag to reduce code duplication and save on bundle size.
Async/Await
Provides a helper function for handling asynchronous operations using async/await syntax in environments that do not natively support these features.
__awaiter(this, void 0, void 0, function* () { const result = yield someAsyncFunction(); return result; })
Spread and Rest
Offers a helper function to handle spread and rest operations with arrays, allowing for the combination and copying of arrays.
__spreadArrays([1, 2], [3, 4])
Decorators
Includes helper functions for applying decorators to classes and methods, which are a TypeScript feature for adding annotations and a meta-programming syntax for class declarations and members.
__decorate([decoratorsArray], target, key, desc)
Generators
Provides a helper function for working with generators and the yield keyword, enabling the use of generator functions in environments that do not support them natively.
__generator(this, function (_a) { switch (_a.label) { case 0: _a.trys.push([0, 2, , 3]); return [4 /*yield*/, someGeneratorFunction()]; case 1: _a.sent(); return [3 /*break*/, 3]; case 2: _a.sent(); return [3 /*break*/, 3]; case 3: return [2 /*return*/]; } });
A modular standard library for JavaScript, core-js includes polyfills for ECMAScript features. It is similar to tslib in that it provides functionality to support newer language features on older environments, but it is more comprehensive and includes polyfills for a wider range of ECMAScript features.
Part of the Babel toolchain, babel-runtime is similar to tslib in that it provides a set of helpers for Babel-transpiled code to avoid duplicating helper code across multiple files. It is used in conjunction with Babel's transform-runtime plugin.
A standalone runtime for Regenerator-compiled generator and async functions. It is similar to tslib in providing support for generators and async functions, but it is focused specifically on the transformation of these features by the Regenerator compiler.
This is a runtime library for TypeScript that contains all of the TypeScript helper functions.
This library is primarily used by the --importHelpers
flag in TypeScript.
When using --importHelpers
, a module that uses helper functions like __extends
and __assign
in the following emitted file:
var __assign = (this && this.__assign) || Object.assign || function(t) {
for (var s, i = 1, n = arguments.length; i < n; i++) {
s = arguments[i];
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
t[p] = s[p];
}
return t;
};
exports.x = {};
exports.y = __assign({}, exports.x);
will instead be emitted as something like the following:
var tslib_1 = require("tslib");
exports.x = {};
exports.y = tslib_1.__assign({}, exports.x);
Because this can avoid duplicate declarations of things like __extends
, __assign
, etc., this means delivering users smaller files on average, as well as less runtime overhead.
For optimized bundles with TypeScript, you should absolutely consider using tslib
and --importHelpers
.
For the latest stable version, run:
# TypeScript 3.9.2 or later
npm install tslib
# TypeScript 3.8.4 or earlier
npm install tslib@^1
# TypeScript 2.3.2 or earlier
npm install tslib@1.6.1
# TypeScript 3.9.2 or later
yarn add tslib
# TypeScript 3.8.4 or earlier
yarn add tslib@^1
# TypeScript 2.3.2 or earlier
yarn add tslib@1.6.1
# TypeScript 3.9.2 or later
bower install tslib
# TypeScript 3.8.4 or earlier
bower install tslib@^1
# TypeScript 2.3.2 or earlier
bower install tslib@1.6.1
# TypeScript 3.9.2 or later
jspm install tslib
# TypeScript 3.8.4 or earlier
jspm install tslib@^1
# TypeScript 2.3.2 or earlier
jspm install tslib@1.6.1
Set the importHelpers
compiler option on the command line:
tsc --importHelpers file.ts
or in your tsconfig.json:
{
"compilerOptions": {
"importHelpers": true
}
}
You will need to add a paths
mapping for tslib
, e.g. For Bower users:
{
"compilerOptions": {
"module": "amd",
"importHelpers": true,
"baseUrl": "./",
"paths": {
"tslib" : ["bower_components/tslib/tslib.d.ts"]
}
}
}
For JSPM users:
{
"compilerOptions": {
"module": "system",
"importHelpers": true,
"baseUrl": "./",
"paths": {
"tslib" : ["jspm_packages/npm/tslib@2.x.y/tslib.d.ts"]
}
}
}
package.json
and bower.json
git tag [version]
git push --tags
Done.
There are many ways to contribute to TypeScript.
FAQs
Runtime library for TypeScript helper functions
The npm package tslib receives a total of 199,761,740 weekly downloads. As such, tslib popularity was classified as popular.
We found that tslib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.