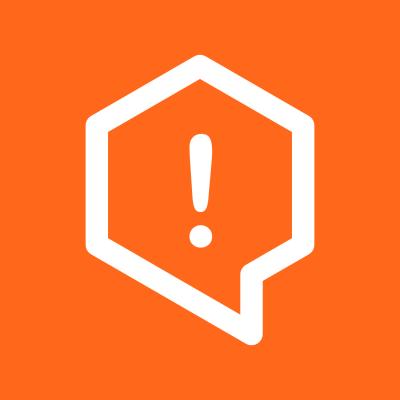
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
typescript-collections
Advanced tools
A complete, fully tested data structure library written in TypeScript.
It is a complete, fully tested data structure library written in TypeScript.
This project uses TypeScript Generics so you need TS 0.9 and above.
This projects supports UMD (Universal Module Definition)
It also includes several functions for manipulating arrays.
npm install typescript-collections --save
ES6 import ... from
import * as Collections from 'typescript-collections';
or TypeScript import ... require
import Collections = require('typescript-collections');
or JavaScript var ... require
var Collections = require('typescript-collections');
Visual Studio or other TypeScript IDE, will provide you with complete Intellisense (autocomplete) for your types. The compiler will ensure that the collections contain the correct elements.
A sample Visual Studio project is in the demo folder.
Also available on NuGet : http://www.nuget.org/packages/typescript.collections/ Thanks to https://github.com/georgiosd
import * as Collections from 'typescript-collections';
var mySet = new Collections.Set<number>();
mySet.add(123);
mySet.add(123); // Duplicates not allowed in a set
// The following will give error due to wrong type:
// mySet.add("asdf"); // Can only add numbers since that is the type argument.
var myQueue = new Collections.Queue();
myQueue.enqueue(1);
myQueue.enqueue(2);
console.log(myQueue.dequeue()); // prints 1
console.log(myQueue.dequeue()); // prints 2
Remember to set "moduleResolution": "node"
, so TypeScript compiler can resolve typings in the node_modules/typescript-collections
directory.
You should include umd.js
or umd.min.js
from dist/lib/
directory.
<script src="[server public path]/typescript-collections/dist/lib/umd.min.js"></script>
Equality is important for hashing (e.g. dictionary / sets). Javascript only allows strings to be keys for the base dictionary {}. This is why the implementation for these data structures uses the item's toString() method.
A simple function is provided for you when you need a quick toString that uses all properties. E.g:
import * as Collections from 'typescript-collections';
class Car {
constructor(public company: string, public type: string, public year: number) {
}
toString() {
// Short hand. Adds each own property
return Collections.util.makeString(this);
}
}
console.log(new Car("BMW", "A", 2016).toString());
Output:
{company:BMW,type:A,year:2016}
import * as Collections from 'typescript-collections';
class Person {
constructor(public name: string, public yearOfBirth: number,public city?:string) {
}
toString() {
return this.name + "-" + this.yearOfBirth; // City is not a part of the key.
}
}
class Car {
constructor(public company: string, public type: string, public year: number) {
}
toString() {
// Short hand. Adds each own property
return Collections.util.makeString(this);
}
}
var dict = new Collections.Dictionary<Person, Car>();
dict.setValue(new Person("john", 1970,"melbourne"), new Car("honda", "city", 2002));
dict.setValue(new Person("gavin", 1984), new Car("ferrari", "F50", 2006));
console.log("Orig");
console.log(dict);
// Changes the same john, since city is not part of key
dict.setValue(new Person("john", 1970, "sydney"), new Car("honda", "accord", 2006));
// Add a new john
dict.setValue(new Person("john", 1971), new Car("nissan", "micra", 2010));
console.log("Updated");
console.log(dict);
// Showing getting / setting a single car:
console.log("Single Item");
var person = new Person("john", 1970);
console.log("-Person:");
console.log(person);
var car = dict.getValue(person);
console.log("-Car:");
console.log(car.toString());
Output:
Orig
{
john-1970 : {company:honda,type:city,year:2002}
gavin-1984 : {company:ferrari,type:F50,year:2006}
}
Updated
{
john-1970 : {company:honda,type:accord,year:2006}
gavin-1984 : {company:ferrari,type:F50,year:2006}
john-1971 : {company:nissan,type:micra,year:2010}
}
Single Item
-Person:
john-1970
-Car:
{company:honda,type:accord,year:2006}
Also known as Factory Dictionary
[ref.]
If a key doesn't exist, the Default Dictionary automatically creates it with setDefault(defaultValue)
.
Default Dictionary is a @michaelneu contribution which copies Python's defaultDict.
Compile, test and check coverage
npm run all
If it supports JavaScript, it probably supports this library.
bas AT basarat.com
Project is based on the excellent original javascript version called buckets
FAQs
A complete, fully tested data structure library written in TypeScript.
The npm package typescript-collections receives a total of 62,300 weekly downloads. As such, typescript-collections popularity was classified as popular.
We found that typescript-collections demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.