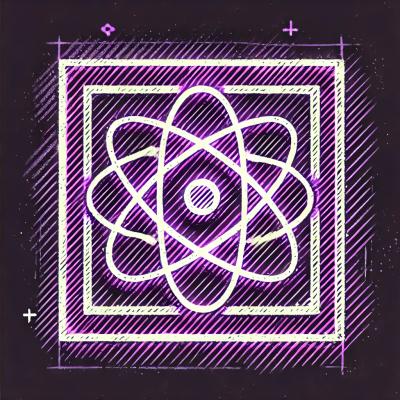
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Lightweight JSON UDP socket API written in JavaScript for NodeJS. It provides a simple protocol on top of UDP to send/receive JSON objects. It does not handle UDP unreliability.
A UDP datagram consists of a datagram header and a data section. In the data section of each UDP datagram, udp-json
adds another header and a new data section. The header consists of 3 fields, each of which is 4 bytes (32 bits):
+----------+----------------------+------------------------+
| ID (4 B) | Datagram count (4 B) | Current datagram (4 B) |
+----------+----------------------+------------------------+
| |
| Partial Data |
| |
+----------+----------------------+------------------------+
This field identifies to which data refers this partial data.
This field specifies the number of UDP datagrams needed to complete the data.
This field identifies this UDP datagram location inside all the UDP datagrams to complete the data in the correct order.
Fragment of JSON stringified data encoded in utf-8
.
Example of sending the object { attribute: "dummy" }
split in two datagrams.
+----------+----------------------+------------------------+
| ID = 333 | Datagram count = 2 | Current datagram = 0 |
+----------+----------------------+------------------------+
| |
| {attr |
| |
+----------+----------------------+------------------------+
+----------+----------------------+------------------------+
| ID = 333 | Datagram count = 2 | Current datagram = 1 |
+----------+----------------------+------------------------+
| |
| ibute: "dummy"} |
| |
+----------+----------------------+------------------------+
See examples folder for more info.
const JSONSocket = require('udp-json');
// Listener socket
const socket = dgram.createSocket('udp4');
socket.bind(56554, '127.0.0.1');
const jsonSocket = new JSONSocket(socket)
jsonSocket.on('message-complete', (msg, rinfo) => {
console.log('Message received', rinfo, msg);
})
// Sender socket
const socket2 = dgram.createSocket('udp4');
const jsonSocket2 = new JSONSocket(socket2, {maxPayload: 496, timeout: 1000});
jsonSocket2.send({ dummy: 'Dummy Text' }, 56554, '127.0.0.1', (e) => {
if (e) {
console.log('error', e);
return;
}
console.log('Message sent');
});
Here are listed all methods implemented in udp-json
.
JSONSocket()
JSONSocket(Socket[, options])
. Creates a JSON socket using the given options
.
JSONSocket: options
Available options for creating a socket. It must be an object
with the following properties:
Property | Type | Description |
---|---|---|
maxPayload | <number> | Max payload size (in bytes). If the resulting byte array exceeds this size, it will be splitted in chunks sent in different datagrams. Default: 496 . |
timeout | <number> | Max time (in ms) between receiving a new datagram and the last datagram received. Default: 1000 . |
send()
send(obj, port[, address][, callback])
. Broadcasts an object on the socket. The destination port
must be specified. An optional callback
function may be specified to as a way of reporting errors or for determining when all datagrams have been sent.
'message-complete'
The 'message-complete'
event is emitted when a new object is available on a socket. The event handler function is passed two arguments: obj
and rinfo
.
'message-error'
The 'message-error'
event is emitted whenever any error occurs. The event handler function is passed a single Error
object.
'message-timeout'
The 'message-timeout'
event is emitted if a partial message times out from not receiving a new datagram. The event handler function is passed a single Error
object.
The library is released under the MIT license. For more information see LICENSE
.
FAQs
Lightweight JSON UDP socket API written in JavaScript for NodeJS
The npm package udp-json receives a total of 0 weekly downloads. As such, udp-json popularity was classified as not popular.
We found that udp-json demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.