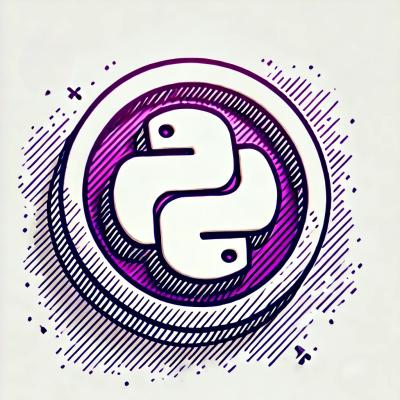
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
unified-errors-handler
Advanced tools
Unified Errors Handler is A Powerful Error Handling Library for Node.js that unify error structure across application. it can unify database errors.
Unified Errors Handler is A Powerful Error Handling Library for Node.js that unify error structure across application. it can unify database errors.
$ npm i unified-errors-handler
const express = require('express');
const { expressExceptionHandler } = require('unified-errors-handler');
const app = express();
/**
response in case of error will be
{
errors: [
{
code: 'USER_NOT_FOUND',
message: 'user not found',
},
],
}
with status code 404
*/
app.post('/test', function (req, res) {
const isFound = // ...
if (isFound) {
// return response
} else {
throw new NotFoundException([
{
code: 'USER_NOT_FOUND',
message: 'user not found',
},
]);
}
});
app.use(expressExceptionHandler());
const express = require('express');
const { exceptionMapper } = require('unified-errors-handler');
const app = express();
/**
response in case of error will be
{
errors: [
{
code: 'USER_NOT_FOUND',
message: 'user not found',
},
],
}
with status code 404
*/
app.post('/test', function (req, res) {
const isFound = // ...
if (isFound) {
// return response
} else {
throw new NotFoundException([
{
code: 'USER_NOT_FOUND',
message: 'user not found',
},
]);
}
});
app.use((err: Error, req: any, res: any, next: any) => {
const mappedError = exceptionMapper(err);
res.status(mappedError.statusCode).send({
errors: mappedError.serializeErrors(),
});
});
const { exceptionMapper } = require('unified-errors-handler');
@Catch()
export class AllExceptionsFilter implements ExceptionFilter {
catch(exception: unknown, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const error = exceptionMapper(exception);
const statusCode = error.statusCode;
response.status(statusCode).json({
errors: error.serializeErrors(),
});
}
}
You can add options to (enable/disable) parsing for database errors (depends on your ORM) this is disabled by default, See supported ORMs
const options = {
mapDBExceptions: true, // deprecated
parseSequelizeExceptions: true,
parseMongooseExceptions: true,
parseTypeORMExceptions: true,
parseObjectionJSExceptions: true,
parseKnexJSExceptions: false,
}
expressExceptionHandler(options)
// or
const mappedError = exceptionMapper(err, options);
{
errors: [{
fields: ['name', 'password'], // optional
code: 'YOUR_CODE',
message: 'your message'
details: { // optional - more details about error
key: value
}
}]
}
throw new BadRequestException({
fields: ['password'], // optional
code: 'INVALID_PASSWORD', // optional
message: 'invalid password'
details: { // optional
// ... more details
}
});
throw new UnauthorizedException({
code: 'UNAUTHORIZED',
message: 'You are not authorized'
});
throw new ForbiddenException({
code: 'FORBIDDEN',
message: 'You have no access'
});
throw new NotFoundException([
{
code: 'USER_NOT_FOUND',
message: 'user not found',
},
]);
throw new ServerException();
// output
[
{
fields: ['name'],
code: 'DATA_ALREADY_EXIST',
message: 'name already exist',
},
]
// output
// foreign key is not exist as primary key in another table
// trying insert value with invalid foreign key
[
code: 'INVALID_DATA',
message: 'Invalid data',
details: {
reason: 'violates foreign key constraint',
constraint: 'pet_user_id_foreign',
},
]
// foreign key has reference in another table
[
code: 'DATA_HAS_REFERENCE',
message: 'Data has reference',
details: {
reason: 'violates foreign key constraint',
constraint: 'pet_user_id_foreign',
},
]
// output
[
{
fields: ['age'],
code: 'INVALID_DATA',
message: 'age is invalid',
details: { reason: 'age must not be NULL' },
},
]
// output
[{
code: 'INVALID_VALUES',
message: 'Invalid Values',
details: {
constraint: 'user_gender_check',
},
}]
// output
[{
{
code: 'OUT_OF_RANGE',
message: 'Out of range',
},
}]
// output
[
{
fields: ['name'],
values: ['Ahmed'],
code: 'DATA_ALREADY_EXIST',
message: 'name already exist',
},
]
// output
[
// field is required
{
fields: ['age'],
message: 'Path `age` is required.',
code: 'MONGODB_VALIDATION_ERROR',
details: {
reason: 'age is required',
violate: 'required_validation'
},
},
// field's value violate enum values
{
fields: ['gender'],
message: '`MALEE` is not a valid enum value for path `gender`.',
code: 'MONGODB_VALIDATION_ERROR',
details: {
reason: "gender's value must be one of MALE, FEMALE",
violate: 'enum_validation'
},
},
// field's value violate max value
{
fields: ['age'],
message: 'Path `age` (300) is more than maximum allowed value (50).',
code: 'MONGODB_VALIDATION_ERROR',
details: {
reason: `age's value exceed maximum allowed value (50)`,
violate: 'max_validation'
},
},
// field's value violate min value
{
fields: ['age'],
message: 'Path `age` (3) is less than minimum allowed value (20).',
code: 'MONGODB_VALIDATION_ERROR',
details: {
reason: `age's value less than minimum allowed value (20)`,
violate: 'min_validation'
},
},
// field's value violate type of field
{
fields: ['age'],
message: 'age is invalid',
code: 'MONGODB_CASTING_ERROR',
},
]
You can create your own exceptions by extend BaseException
export class MyCustomException extends BaseException {
statusCode = 400;
constructor(private message: string) {
super(message);
Object.setPrototypeOf(this, MyCustomException.prototype);
}
serializeErrors() {
return [{
message,
code: 'CUSTOM_CODE'
}];
}
}
const options = {
loggerOptions: {
console: {
format: ':time :message', // optional - default message only
colored: true, // optional - default no color
},
},
}
expressExceptionHandler(options)
// or
const mappedError = exceptionMapper(err, options);
implement ILogger interface
import { ILogger } from 'unified-errors-handler';
class CustomLogger implements ILogger {
log(error: any): void {
console.log(error.message);
}
}
// in options pass this object
const options = {
loggerOptions: {
custom: new CustomLogger(),
},
}
expressExceptionHandler(options)
// or
const mappedError = exceptionMapper(err, options);
To run the test suite,
docker-comose up
or set your own connection URLs for postgres database and mysql database in .envnpm test
:$ npm install
$ npm test
Feel free to open issues on github.
FAQs
Unified Errors Handler is A Powerful Error Handling Library for Node.js that unify error structure across application. it can unify database errors.
The npm package unified-errors-handler receives a total of 31 weekly downloads. As such, unified-errors-handler popularity was classified as not popular.
We found that unified-errors-handler demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.