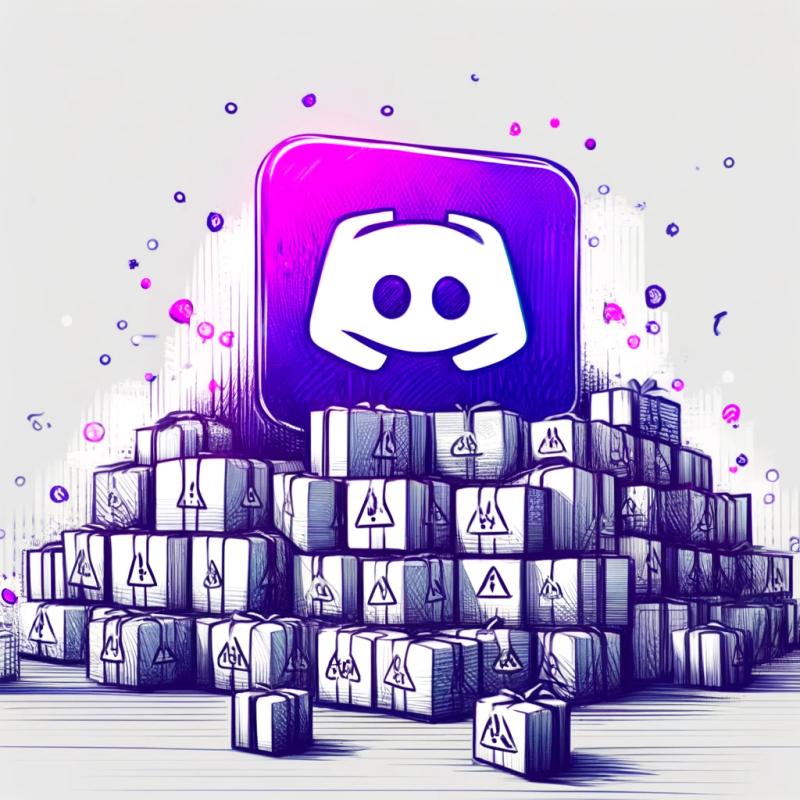
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
unist-util-is
Advanced tools
Package description
The unist-util-is npm package is a utility library for working with Unist nodes. Unist (Universal Syntax Tree) is part of the unified ecosystem, which provides a way to work with syntax trees for content such as Markdown, HTML, or plain text. unist-util-is specifically helps in testing and filtering nodes in these trees based on certain conditions.
Test nodes with a condition
This feature allows you to test if a node matches a specific type or condition. In the example, `is` is used to check if the root node is a 'leaf' (which it isn't), and then if the first child of the root is a 'leaf' (which it is).
const is = require('unist-util-is');
const u = require('unist-builder');
const tree = u('root', [
u('leaf', 'first leaf'),
u('node', [u('leaf', 'nested leaf')])
]);
const test = is(tree, 'leaf'); // false
const testLeaf = is(tree.children[0], 'leaf'); // true
Filter nodes by type
This feature demonstrates how to filter nodes by type using `is` in combination with `unist-util-select`. It selects all 'leaf' nodes from the tree and filters them to ensure they are of type 'leaf'.
const is = require('unist-util-is');
const u = require('unist-builder');
const select = require('unist-util-select');
const tree = u('root', [
u('leaf', 'first leaf'),
u('node', [u('leaf', 'nested leaf')])
]);
const leaves = select.selectAll('leaf', tree).filter(node => is(node, 'leaf'));
This package is similar to unist-util-is in that it is used to work with Unist nodes. However, unist-util-visit focuses on visiting nodes within a tree, optionally filtering nodes, and applying a function to each node. It differs from unist-util-is by providing traversal capabilities rather than just testing or filtering.
unist-util-select is used to select nodes from a Unist tree using CSS-like selectors. It complements unist-util-is by providing a way to retrieve nodes based on complex queries, whereas unist-util-is is more focused on testing nodes against specified conditions.
Readme
unist utility to check if nodes pass a test.
This package is a small utility that checks that a node is a certain node.
Use this small utility if you find yourself repeating code for checking what nodes are.
A similar package, hast-util-is-element
, works on hast
elements.
For more advanced tests, unist-util-select
can be used
to match against CSS selectors.
This package is ESM only. In Node.js (version 14.14+ and 16.0+), install with npm:
npm install unist-util-is
In Deno with esm.sh
:
import {is} from 'https://esm.sh/unist-util-is@5'
In browsers with esm.sh
:
<script type="module">
import {is} from 'https://esm.sh/unist-util-is@5?bundle'
</script>
import {is} from 'unist-util-is'
const node = {type: 'strong'}
const parent = {type: 'paragraph', children: [node]}
is() // => false
is({children: []}) // => false
is(node) // => true
is(node, 'strong') // => true
is(node, 'emphasis') // => false
is(node, node) // => true
is(parent, {type: 'paragraph'}) // => true
is(parent, {type: 'strong'}) // => false
is(node, test) // => false
is(node, test, 4, parent) // => false
is(node, test, 5, parent) // => true
function test(node, n) {
return n === 5
}
This package exports the identifiers convert
and is
.
There is no default export.
is(node[, test[, index, parent[, context]]])
Check if node
is a Node
and whether it passes the given test.
node
(unknown
)
— thing to check, typically Node
test
(Test
or PredicateTest
, optional)
— a check for a specific elementindex
(number
, optional)
— the node’s position in its parentparent
(Node
, optional)
— the node’s parentcontext
(any
, optional)
— context object (this
) to call test
withWhether node
is a Node
and passes a test (boolean
).
When an incorrect test
, index
, or parent
is given.
There is no error thrown when node
is not a node.
convert(test)
Generate a check from a test.
Useful if you’re going to test many nodes, for example when creating a utility where something else passes a compatible test.
The created function is a bit faster because it expects valid input only:
a node
, index
, and parent
.
test
(Test
or PredicateTest
, optional)
— a check for a specific nodeAn assertion (AssertAnything
or
AssertPredicate
).
AssertAnything
Check that an arbitrary value is a node, unaware of TypeScript inferral (TypeScript type).
node
(unknown
)
— anything (typically a node)index
(number
, optional)
— the node’s position in its parentparent
(Node
, optional)
— the node’s parentWhether this is a node and passes a test (boolean
).
AssertPredicate
Check that an arbitrary value is a specific node, aware of TypeScript (TypeScript type).
Kind
(Node
)
— node typenode
(unknown
)
— anything (typically a node)index
(number
, optional)
— the node’s position in its parentparent
(Node
, optional)
— the node’s parentWhether this is a node and passes a test (node is Kind
).
Test
Check for an arbitrary node, unaware of TypeScript inferral (TypeScript type).
type Test =
| null
| undefined
| string
| Record<string, unknown>
| TestFunctionAnything
| Array<string | Record<string, unknown> | TestFunctionAnything>
Checks that the given thing is a node, and then:
string
, checks that the node has that tag namefunction
, see TestFunctionAnything
object
, checks that all keys in test are in node, and that they have
(strictly) equal valuesArray
, checks if one of the subtests passTestFunctionAnything
Check if a node passes a test, unaware of TypeScript inferral (TypeScript type).
node
(Node
)
— a nodeindex
(number
, optional)
— the node’s position in its parentparent
(Node
, optional)
— the node’s parentWhether this node passes the test (boolean
).
PredicateTest
Check for a node that can be inferred by TypeScript (TypeScript type).
type PredicateTest<Kind extends Node> =
| Kind['type']
| Partial<Kind>
| TestFunctionPredicate<Kind>
| Array<Kind['type'] | Partial<Kind> | TestFunctionPredicate<Kind>>
TestFunctionPredicate
Check if a node passes a certain node test (TypeScript type).
Kind
(Node
)
— node typenode
(Node
)
— a nodeindex
(number
, optional)
— the node’s position in its parentparent
(Node
, optional)
— the node’s parentWhether this node passes the test (node is Kind
).
convert
import {u} from 'unist-builder'
import {convert} from 'unist-util-is'
const test = convert('leaf')
const tree = u('tree', [
u('node', [u('leaf', '1')]),
u('leaf', '2'),
u('node', [u('leaf', '3'), u('leaf', '4')]),
u('leaf', '5')
])
const leafs = tree.children.filter((child, index) => test(child, index, tree))
console.log(leafs)
Yields:
[{type: 'leaf', value: '2'}, {type: 'leaf', value: '5'}]
This package is fully typed with TypeScript.
It exports the additional types AssertAnything
,
AssertPredicate
, Test
,
TestFunctionAnything
,
TestFunctionPredicate
, and
PredicateTest
.
Projects maintained by the unified collective are compatible with all maintained versions of Node.js. As of now, that is Node.js 14.14+ and 16.0+. Our projects sometimes work with older versions, but this is not guaranteed.
unist-util-find-after
— find a node after another nodeunist-util-find-before
— find a node before another nodeunist-util-find-all-after
— find all nodes after another nodeunist-util-find-all-before
— find all nodes before another nodeunist-util-find-all-between
— find all nodes between two nodesunist-util-filter
— create a new tree with nodes that pass a checkunist-util-remove
— remove nodes from treeSee contributing.md
in syntax-tree/.github
for
ways to get started.
See support.md
for ways to get help.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
FAQs
unist utility to check if a node passes a test
We found that unist-util-is demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.