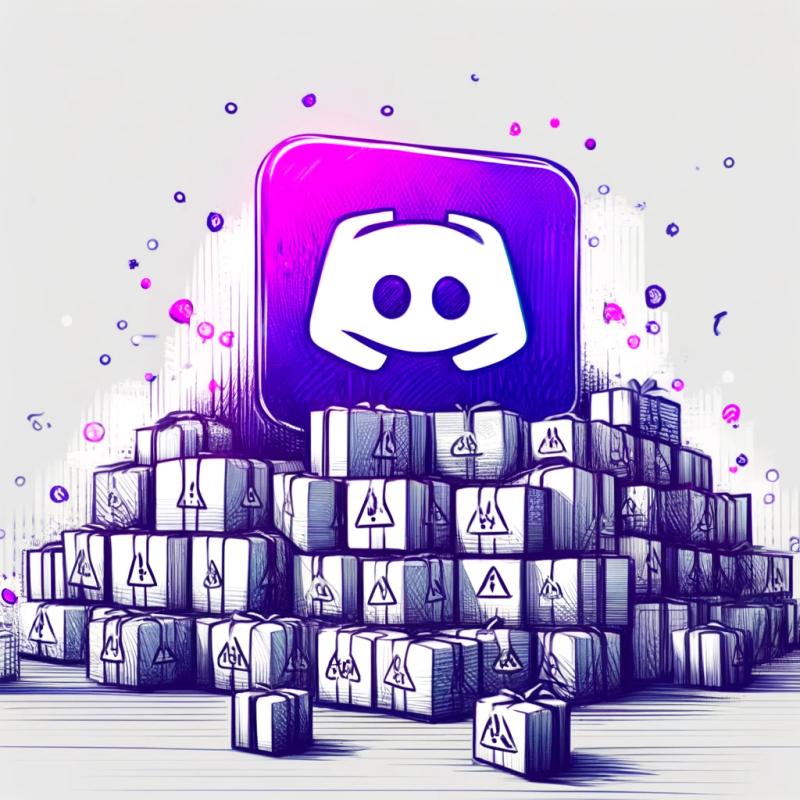
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
venezia
Advanced tools
Readme
A simple framework to easily create Web Component
"Why Venezia ?
- No special reason, I like this city... So why not ?
First, add venezia to your project :
npm install venezia
Then, create and register your component :
import Venezia from 'venezia';
class Test extends Venezia {
render() {
return `<span>Hello World</span>`;
}
}
Test.component('my-test');
Finaly, use this component in your HTML page :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Venezia sample</title>
<script type="module" src="my-test.js"></script>
</head>
<body>
<my-test></my-test>
</body>
</html>
constructor()
: This is the contructor of your component class. If you add a constructor to your component, you must call super()
!
willConnect()
: This function is called when the element is connected to the DOM for the first time, before rendering.
rendered()
: This function is called when the element has been rendered. Since the render
function can be async, this function can be called after the connected
function.
connected()
: This function is called when the element is connected to the DOM for the first time, juste aften the render
function has been called.
<property>Changed(oldValue, newValue)
: This function is called when the property
change. See Properties.
adopted()
: This function is called when the element is moved to a new document.
disconnected()
: This function is called when the element is disconnected from the DOM.
render
(and reRender
)The render
function must return the HTML code of your component.
This function can be sync:
class Sample extends Venezia {
render() {
return `<span>Hello Sync World!</span>`;
}
}
Async:
class Sample extends Venezia {
async render() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(`<span>Hello Async World!</span>`);
}, 1000);
});
}
}
An async generator:
class Sample extends Venezia {
async *render() {
yield `<span>Changing...</span>`;
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(`<span>Hello Async Generated World!</span>`);
}, 1000);
});
}
}
You can manually update a component by calling the reRender()
function.
stylesheet
If you want to add CSS to your component, you can use the the stylesheet
function. It must return the CSS code for your component:
class Sample extends Venezia {
stylesheet() {
return `
span {
background-color: red;
color: #fff;
}
`;
}
}
All properties added to a component are stored in the props
attributes. For example, if you declare:
<my-component status="example"></my-component>
You can access to the value of the status
attribute with this.props['status']
(or this.props.status
).
If you want to be informed when an attribute is added, removed or updated, you can create a <property>Changed
function. This function will receive the old and the new value for the attribute. So if you wan't to be informed if the status
attribut change (in the previous example), you can do this:
class Sample extends Venezia {
statusChanhed(oldValue, newValue) {
// Do something with oldValue and newValue...
}
}
:warning: The
<property>Changed()
functions are only called when the attribute change. But not if you modify the corresponding property (this.props.status
in our example). However, be aware that when the<attribute>Changed
function is called; the corresponding property (this.props.status
in our example) has already been modified byVenezia
.
If you want to capture an interaction event in your component, no need to create the corresponding event listener. You just need to create a function for the corresponding event. For example, to capture a click event, create a click()
function:
class Sample extends Venezia {
click(event) {
console.log('click', event);
}
}
To register your component, simply call the static function component
by passing it the name for your component.
class Sample extends Venezia {
// ...
}
Sample.component('my-sample');
:warning: Web components require a name with two or more parts.
Copyright (c) 2021 Grégoire Lejeune
All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
FAQs
Simple framework to create Web Component
We found that venezia demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.