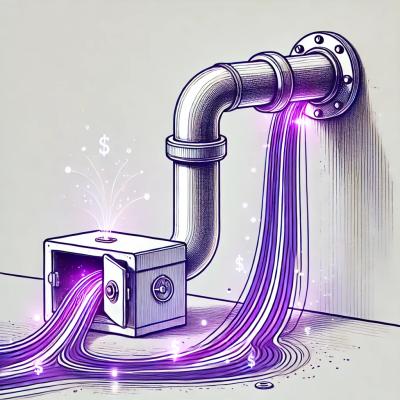
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
visa2discord
Advanced tools
For your discord utilities
To install this package, you can use the following command:
npm i visa2discord
To install this package, you can also use the following command:
yarn add visa2discord
The sleep
function is used to introduce a delay in the execution of code. It can be useful in scenarios where you want to pause the execution for a specific period of time.
Usage
const { sleep } = require('visa2discord');
async function example() {
console.log('Before sleep');
await sleep(2000); // Sleep for 2000 milliseconds (2 seconds)
console.log('After sleep');
}
example();
The passGen
function is used to generate a random password.
Usage
const { passGen } = require('visa2discord');
const password = passGen(6) // Generate a password with 6 characters
console.log('Generated password:', password);
Example output
Generated password: 5a2d3f
The AutoThreadJoiner
function is used to automatically join threads.
Usage
const { Client } = require('discord.js');
const client = new Client({ intents: ['GUILDS', 'GUILD_MESSAGES', 'GUILD_MESSAGE_REACTIONS'] });
const { AutoThreadJoiner } = require('visa2discord');
AutoThreadJoiner(client) // Automatically join threads
The cleanCode
function is used to escape mentions and codeblocks in a given text string.
Usage
const { cleanCode } = require('visa2discord');
const code = 'const message = "Hello, this is a test message."';
const cleanedCode = cleanCode(code);
console.log('Cleaned code:', cleanedCode);
Example output
Cleaned code: const message = "Hello, this is a test message."
The splitMessageRegex
function is used to split a message into multiple messages to avoid the 2000 character limit by Discord.
Parameters
text
: The message to split into parts.optional
): An object containing the following properties:
maxLength
: The maximum length of each part (default: 2000
).regex
: The regular expression to use as the delimiter (default: /\n/g
).prepend
: The string to prepend to each part (default: ''
).append
: The string to append to each part (default: ''
).Usage
const { splitMessageRegex } = require('visa2discord');
const message = `This is a long message that needs to be split into multiple parts.\nIt contains multiple lines and exceeds the maximum message length.`;
const parts = splitMessageRegex(message, {
maxLength: 50,
regex: /\n/g,
prepend: '```',
append: '```'
});
console.log('Message parts:', parts);
Example output
Message parts: [
'```This is a long message that needs to be split into multiple parts.```',
'```It contains multiple lines and exceeds the maximum message length.```'
]
The discordTimestamp
function is used to generate a discord timestamp.
Usage
const { discordTimestamp } = require('visa2discord');
const time = Date.now(); // Current time
const type = 'f'; // type of timestamp
const timestamp = discordTimestamp(time, type);
console.log('Discord timestamp:', timestamp);
Here are the types to use and their outputs on Discord:
d
=> 03/05/2023
D
=> March 5, 2023
t
=> 2:22 PM
T
=> 2:22:00 PM
f
=> March 5, 2023 2:22 PM
F
=> Sunday, March 5, 2023 2:22 PM
R
=> A minute ago
The generateActivity
function is used to generate a discord activity.
Usage
const { generateActivity } = require('visa2discord');
const customActivity = await generateActivity(client, channel, { custom: "814288819477020702" })
const nameActivity = await generateActivity(client, channel, { name: "youtube" })
console.log('Generated activity:', `discord.gg/${customActivity.code}`);
console.log('Generated activity:', `discord.gg/${nameActivity.code}`);
Here are the names that are supported:
youtube
=> Youtube Togetherpoker
=> Poker Nightbetrayal
=> Betrayal.iofishing
=> Fishington.iochess
=> Chess in the Parkcheckers
=> Checkers.doodlecrew
=> Doodle Crewlettertile
=> Letter Tilespellcast
=> Spellcastwordsnacks
=> Word Snackswatchtogether
=> Watch Togetherocho
=> Ochosketchheads
=> Sketch Headschessdev
=> Chess in the Park (Dev)fishingdev
=> Fishington.io (Dev)pokerdev
=> Poker Night (Dev)letterleague
=> Letter Leaguewatch
=> WatchdisableButtons
function is used to disable all buttons in a messageconst { disableButtons } = require('visa2discord');
const message = await channel.send({ content: 'This is a test message', components: [row] });
message.edit({ components: disableButtons(message) });
transcripts
function is used to generate a transcript of a Discord channelquickExport
quickExport
function generates a transcript of messages from a Discord channel. It takes the following parameters:
channel
(required): The channel to export the transcript from.messages
(optional): An array of specific messages to include in the transcript. By default, it includes all messages.guild
(optional): The guild associated with the channel, if available.client
(optional): The Discord client used for fetching messages, if available.const { AttachmentBuilder } = require('discord.js');
const { quickExport } = require('visa2discord');
const channel = client.channels.cache.get("channel_id") || message.channel;
const transcript = await quickTranscript(channel);
//Gets latest 100 messages
channel.send({ files: [new AttachmentBuilder(transcript, { name: 'transcript.html'})] });
exportChat
exportChat
function exports the chat transcript from a Discord channel. It is an asynchronous function that returns a Promise. It takes the following parameters:
channel
(required): The channel to export the chat from.limit
(optional): The maximum number of messages to export. If not specified, all messages will be exported.tz_info
(optional): The timezone information for the transcript. Defaults to 'UTC'.guild
(optional): The guild associated with the channel, if available.client
(optional): The Discord client object, if available.military_time
(optional): Whether to use military time format for timestamps. Defaults to true.fancy_times
(optional): Whether to use fancy formatting for timestamps. Defaults to true.before
(optional): Limit the exported messages to those created before this date.after
(optional): Limit the exported messages to those created after this date.support_dev
(optional): Whether to include developer support information in the transcript. Defaults to true.const { AttachmentBuilder } = require('discord.js');
const { exportChat } = require('visa2discord');
const channel = client.channels.cache.get("channel_id") || message.channel;
const transcript = await exportChat(channel);
channel.send({ files: [new AttachmentBuilder(Buffer.from(transcript, 'utf-8'), { name: 'transcript.html'})] });
rawExport
The rawExport
function exports the raw chat transcript from a Discord channel. It is an asynchronous function that returns a Promise. It takes the following parameters:
channel
(required): The channel to export the chat from.messages
(required): The specific messages to include in the export.tz_info
(optional): The timezone information for the transcript. Defaults to 'UTC'.guild
(optional): The guild associated with the channel, if available.client
(optional): The Discord client object, if available.military_time
(optional): Whether to use military time format for timestamps. Defaults to false.fancy_times
(optional): Whether to use fancy formatting for timestamps. Defaults to true.support_dev
(optional): Whether to include developer support information in the transcript. Defaults to true.The function returns a Promise that resolves to the raw HTML content of the exported chat transcript. If an error occurs while exporting the chat transcript, an Error is thrown.
Usage
const { AttachmentBuilder } = require('discord.js');
const { rawExport } = require('visa2discord');
const channel = client.channels.cache.get("channel_id") || message.channel;
const messages = await channel.messages.fetch({ limit: 100 });
const transcript = await rawExport(channel, messages);
channel.send({ files: [new AttachmentBuilder(Buffer.from(transcript, 'utf-8'), { name: 'transcript.html'})] });
Transcript
Transcript
class represents a chat transcript export and extends the TranscriptDAO
class.const { prettyBytes } = require('visa2discord');
const bytes = 1000000000;
const formatted = prettyBytes(bytes);
console.log('Formatted bytes:', formatted);
Example output
Formatted bytes: 1 GB
visa2code#1747
, you can join my discord server.FAQs
A package for your discord needs
The npm package visa2discord receives a total of 78 weekly downloads. As such, visa2discord popularity was classified as not popular.
We found that visa2discord demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.