What is vscode-html-languageservice?
The vscode-html-languageservice npm package provides a set of tools and services for working with HTML in Visual Studio Code extensions. It offers functionalities such as HTML validation, completion, hover information, and more, making it easier to build and enhance HTML editing capabilities in VS Code.
What are vscode-html-languageservice's main functionalities?
HTML Validation
This feature allows you to validate HTML documents and get a list of diagnostics (errors, warnings, etc.). The code sample demonstrates how to create a document and use the HTML language service to perform validation.
const { getLanguageService } = require('vscode-html-languageservice');
const htmlLanguageService = getLanguageService();
const document = { uri: 'test://test/test.html', languageId: 'html', version: 1, getText: () => '<div></div>' };
const diagnostics = htmlLanguageService.doValidation(document);
console.log(diagnostics);
HTML Completion
This feature provides auto-completion suggestions for HTML documents. The code sample shows how to get completion items at a specific position in the document.
const { getLanguageService } = require('vscode-html-languageservice');
const htmlLanguageService = getLanguageService();
const document = { uri: 'test://test/test.html', languageId: 'html', version: 1, getText: () => '<div></div>' };
const position = { line: 0, character: 5 };
const completions = htmlLanguageService.doComplete(document, position);
console.log(completions);
HTML Hover Information
This feature provides hover information for HTML elements. The code sample demonstrates how to get hover information at a specific position in the document.
const { getLanguageService } = require('vscode-html-languageservice');
const htmlLanguageService = getLanguageService();
const document = { uri: 'test://test/test.html', languageId: 'html', version: 1, getText: () => '<div></div>' };
const position = { line: 0, character: 5 };
const hover = htmlLanguageService.doHover(document, position);
console.log(hover);
Other packages similar to vscode-html-languageservice
vscode-css-languageservice
The vscode-css-languageservice package provides similar functionalities but for CSS. It offers CSS validation, completion, and hover information, making it useful for enhancing CSS editing capabilities in VS Code.
vscode-json-languageservice
The vscode-json-languageservice package provides language services for JSON, including validation, completion, and hover information. It is similar to vscode-html-languageservice but tailored for JSON documents.
vscode-languageserver
The vscode-languageserver package provides a framework for implementing language servers in VS Code. It can be used to create language services for various programming languages, offering a broader scope compared to the HTML-specific services of vscode-html-languageservice.
vscode-html-languageservice
HTML language service extracted from VSCode to be reused, e.g in the Monaco editor.

Why?
The vscode-html-languageservice contains the language smarts behind the HTML editing experience of Visual Studio Code
and the Monaco editor.
-
doComplete / doComplete2 (async) provide completion proposals for a given location.
-
setCompletionParticipants allows participant to provide suggestions for specific tokens.
-
doHover provides hover information at a given location.
-
format formats the code at the given range.
-
findDocumentLinks finds all links in the document.
-
findDocumentSymbols finds all the symbols in the document.
-
getFoldingRanges return folding ranges for the given document.
-
getSelectionRanges return the selection ranges for the given document.
...
For the complete API see htmlLanguageService.ts and htmlLanguageTypes.ts
Installation
npm install --save vscode-html-languageservice
Development
- clone this repo, run yarn
yarn test
to compile and run tests
How can I run and debug the service?
- open the folder in VSCode.
- set breakpoints, e.g. in
htmlCompletion.ts
- run the Unit tests from the run viewlet and wait until a breakpoint is hit:

How can I run and debug the service inside an instance of VSCode?
- run VSCode out of sources setup as described here: https://github.com/Microsoft/vscode/wiki/How-to-Contribute
- link the folder of the
vscode-html-languageservice
repo to vscode/extensions/html-language-features/server
to run VSCode with the latest changes from that folder:
- cd
vscode-html-languageservice
, yarn link
- cd
vscode/extensions/html-language-features/server
, yarn link vscode-html-languageservice
- run VSCode out of source (
vscode/scripts/code.sh|bat
) and open a .html
file - in VSCode window that is open on the
vscode-html-languageservice
sources, run command Debug: Attach to Node process
and pick the code-oss
process with the html-language-features
path
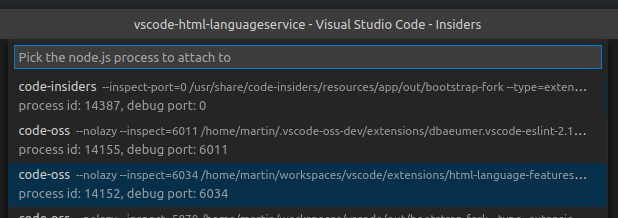
- set breakpoints, e.g. in
htmlCompletion.ts
- in the instance run from sources, invoke code completion in the
.html
file
License
(MIT License)
Copyright 2016-2020, Microsoft
With the exceptions of data/*.json
, which is built upon content from Mozilla Developer Network
and distributed under CC BY-SA 2.5.