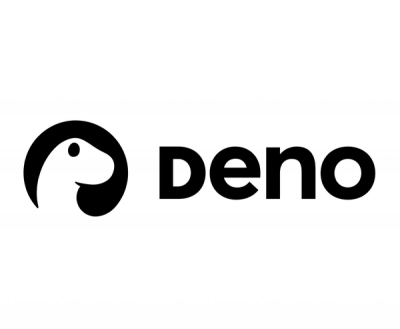
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
vue-chartjs
Advanced tools
vue-chartjs is a wrapper for Chart.js in Vue. It allows you to create various types of charts using the Chart.js library while leveraging the reactive data-binding and component-based architecture of Vue.js.
Line Chart
This code sample demonstrates how to create a simple line chart using vue-chartjs. The `Line` component from vue-chartjs is used to render the chart, and the data and options are passed as props.
```javascript
<template>
<line-chart :chart-data="datacollection" :options="options"></line-chart>
</template>
<script>
import { Line } from 'vue-chartjs'
export default {
components: {
LineChart: Line
},
data () {
return {
datacollection: {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'Data One',
backgroundColor: '#f87979',
data: [40, 39, 10, 40, 39, 80, 40]
}
]
},
options: {
responsive: true,
maintainAspectRatio: false
}
}
}
}
</script>
```
Bar Chart
This code sample demonstrates how to create a bar chart using vue-chartjs. The `Bar` component is used to render the chart, and the data and options are passed as props.
```javascript
<template>
<bar-chart :chart-data="datacollection" :options="options"></bar-chart>
</template>
<script>
import { Bar } from 'vue-chartjs'
export default {
components: {
BarChart: Bar
},
data () {
return {
datacollection: {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'Data One',
backgroundColor: '#f87979',
data: [40, 39, 10, 40, 39, 80, 40]
}
]
},
options: {
responsive: true,
maintainAspectRatio: false
}
}
}
}
</script>
```
Pie Chart
This code sample demonstrates how to create a pie chart using vue-chartjs. The `Pie` component is used to render the chart, and the data and options are passed as props.
```javascript
<template>
<pie-chart :chart-data="datacollection" :options="options"></pie-chart>
</template>
<script>
import { Pie } from 'vue-chartjs'
export default {
components: {
PieChart: Pie
},
data () {
return {
datacollection: {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [
{
label: 'Data One',
backgroundColor: ['#FF6384', '#36A2EB', '#FFCE56', '#4BC0C0', '#9966FF', '#FF9F40'],
data: [12, 19, 3, 5, 2, 3]
}
]
},
options: {
responsive: true,
maintainAspectRatio: false
}
}
}
}
</script>
```
vue-echarts is a wrapper for Apache ECharts, a powerful charting and visualization library. It provides a wide range of chart types and customization options. Compared to vue-chartjs, vue-echarts offers more advanced features and a larger variety of chart types, but it may have a steeper learning curve.
vue-d3 is a library that integrates D3.js with Vue.js. D3.js is a powerful library for creating complex and interactive data visualizations. vue-d3 allows you to use D3.js within Vue components. Compared to vue-chartjs, vue-d3 offers more flexibility and control over the visualizations, but it requires a deeper understanding of D3.js.
vue-apexcharts is a wrapper for ApexCharts, a modern charting library that offers a variety of interactive charts. It is easy to use and integrates well with Vue.js. Compared to vue-chartjs, vue-apexcharts provides more interactive features and a more modern look and feel, but it may not be as widely used or documented.
vue-chartjs is a wrapper for Chart.js in Vue. You can easily create reuseable chart components.
Supports Chart.js v4.
Install this library with peer dependencies:
pnpm add vue-chartjs chart.js
# or
yarn add vue-chartjs chart.js
# or
npm i vue-chartjs chart.js
Then, import and use individual components:
<template>
<Bar :data="data" :options="options" />
</template>
<script lang="ts">
import {
Chart as ChartJS,
Title,
Tooltip,
Legend,
BarElement,
CategoryScale,
LinearScale
} from 'chart.js'
import { Bar } from 'vue-chartjs'
ChartJS.register(CategoryScale, LinearScale, BarElement, Title, Tooltip, Legend)
export default {
name: 'App',
components: {
Bar
},
data() {
return {
data: {
labels: ['January', 'February', 'March'],
datasets: [{ data: [40, 20, 12] }]
},
options: {
responsive: true
}
}
}
}
</script>
Need an API to fetch data? Consider Cube, an open-source API for data apps.
# install dependencies
pnpm install
# build for production with minification
pnpm build
# run unit tests
pnpm test:unit
# run all tests
pnpm test
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)This software is distributed under MIT license.
FAQs
Vue.js wrapper for chart.js for creating beautiful charts.
The npm package vue-chartjs receives a total of 371,951 weekly downloads. As such, vue-chartjs popularity was classified as popular.
We found that vue-chartjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.