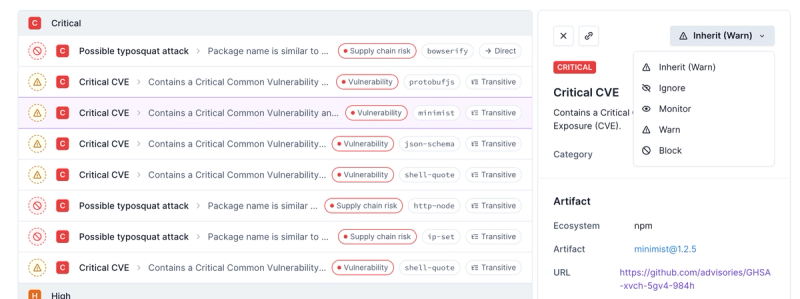
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
vue-hotel-datepicker
Advanced tools
Readme
A responsive date range picker for Vue.js that displays the number of nights selected and allow several useful options like custom check-in/check-out rules, localisation support and more.
https://krystalcampioni.github.io/vue-hotel-datepicker/
npm install vue-hotel-datepicker
pnpm install vue-hotel-datepicker
yarn add vue-hotel-datepicker
import HotelDatePicker from 'vue-hotel-datepicker2'
import 'vue-hotel-datepicker2/dist/vueHotelDatepicker2.css';
export default {
components: {
HotelDatePicker,
},
}
<HotelDatePicker />
Date
null
Allows to stop calendar pagination after the month of that date
Boolean
true
Allows to have half a day, if you have check in at noon and checkout before noon
String
YYYY-MM-DD
The date format string.
Date
or String
new Date()
The start view date. All the dates before this date will be disabled.
Date
null
The initial value of the start date.
Date
or String
or Boolean
false
The end view date. All the dates after this date will be disabled.
Date
null
The initial value of the end date.
Number
0
The first day of the week. Where Sun = 0, Mon = 1, ... Sat = 6.
You need to set the right order in i18n.day-names
too.
Number
1
Minimum nights required to select a range of dates.
Number
0
Maximum nights required to select a range of dates.
Array
[]
An array of strings in this format: YYYY-MM-DD
. All the dates passed to the list will be disabled.
Array
[]
An array of strings in this format: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
. All the days passed to the list will be disabled.
Boolean
false
If true
, allows the checkout on a disabled date.
Boolean
or Function
true
Shows a tooltip with the number of nights when hovering a date.
String
null
If provided, it will override the default tooltip "X nights" with the text provided. You can use HTML in the string.
boolean
false
boolean
false
Shows the year next to the month
boolean
true
boolean
true
If set to true, displays a clear button on the right side of the input if there are dates set
boolean
false
If set to true, disable checkout on the same date has checkin
Object
Default:
i18n: {
night: 'Night',
nights: 'Nights',
'day-names': ['Sun', 'Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat'],
'check-in': 'Check-in',
'check-out': 'Check-Out',
'month-names': ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'],
tooltip: {
halfDayCheckIn: "Available CheckIn",
halfDayCheckOut: "Available CheckOut",
saturdayToSaturday: "Only Saturday to Saturday",
sundayToSunday: "Only Sunday to Sunday",
minimumRequiredPeriod: "A minimum of <br/> %{minNightInPeriod} %{night} is required."
},
week: "week",
weeks: "weeks",
}
Array
[]
If you want to have specific startAt and endAt period with different duration or price or type of period-
Key | Type | Description |
---|---|---|
endAt | String | YYYY-MM-DD |
startAt | String | YYYY-MM-DD |
minimumDuration | Number | Minimum stay (Type: weekly => per_week |
periodType | String | nightly, weekly_by_saturday, weekly_by_sunday |
Example:
periodDates: [
{
startAt: "2020-06-09",
endAt: "2020-07-26",
minimumDuration: 4,
periodType: "nightly"
},
{
startAt: "2020-07-26",
endAt: "2020-09-30",
minimumDuration: 1,
periodType: "weekly_by_saturday"
},
{
startAt: "2020-09-30",
endAt: "2020-11-30",
minimumDuration: 2,
periodType: "weekly_by_sunday",
price: 4000.0
}
],
MinimumDuration
with a periodType weekly-~
equals to a weekBoolean
false
If set to true, displays a price contains on your periodDates
Boolean
false
If set to true, display one month only
Boolean
true
Show or hide a grid around the days
Boolean
false
Display calendar on the right or the left of the input (left by default)
Boolean
false
Display calendar in the page without an input
Array
[]
If you want to show bookings
Key | Type | Description |
---|---|---|
checkInDate | String | YYYY-MM-DD |
checkOutDate | String | YYYY-MM-DD |
style | Object | Style, (see the example) |
Example:
bookings: [
{
event: true,
checkInDate: "2020-08-26",
checkOutDate: "2020-08-29",
style: {
backgroundColor: "#399694"
}
},
{
event: false,
checkInDate: "2020-07-01",
checkOutDate: "2020-07-08",
style: {
backgroundColor: "#9DC1C9"
}
}
],
â ď¸ In order to open/close the datepicker from an external element, such as a button make sure to set closeDatepickerOnClickOutside
to false
Hide datepicker
Show datepicker
Toggle datepicker
Emitted every time when day is clicked
Params:
name | Description |
---|---|
date | new Date() |
formatDate | YYYY-MM-DD |
nextDisabledDate | Date, Number, String |
Emitted every time a new check in date is selected with the new date as payload
Emitted every time a new check out date is selected with the new date as payload
Emitted every time you clicked on clearDate button
Emitted on [beforeMount, clearSelection, checkOut]
Params:
name | Description |
---|---|
checkIncheckOutHalfDay | Object of checkinCheckout date |
Emitted every time a booking is clicked
Params: name | Type | Description --------------------|------------------------- event | MouseEvent | Mouse javascript event date | Date | Clicked Date currentBooking | Object | Clicked Booking
Example of currentBooking:
{
checkInDate: "YYYY-MM-DD",
checkOutDate: "YYYY-MM-DD",
style: {
backgroundColor: "#399694",
}
}
Emitted every time when a checkOut is clicked
name | Type | Description --------------------|------------------------- event | MouseEvent | Mouse javascript event checkIn | Date | checkIn checkIn | Date | checkOut
This component was originally built as a Vue wrapper component for the Hotel Datepicker by @benitolopez. Version 2.0.0 was completely rewritten with Vue, removing the original library, removing some features and introducing others.
FAQs
A responsive date range picker for Vue.js that displays the number of nights selected and allow several useful options like custom check-in/check-out rules, localization support and more
The npm package vue-hotel-datepicker receives a total of 1,481 weekly downloads. As such, vue-hotel-datepicker popularity was classified as popular.
We found that vue-hotel-datepicker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.