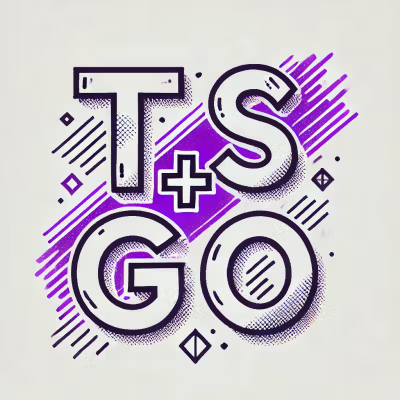
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
webpack-dev-middleware
Advanced tools
webpack-dev-middleware is a package that provides a simple way to serve and live reload webpack bundles for development purposes. It's designed to be used with a Node.js server, such as Express, and it allows developers to serve the webpack-generated files without writing them to disk, providing a faster development experience.
Serving Webpack Bundles
This code sample demonstrates how to set up webpack-dev-middleware with an Express server. It serves the files generated by webpack based on the provided configuration.
const express = require('express');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const config = require('./webpack.config.js');
const compiler = webpack(config);
const app = express();
app.use(webpackDevMiddleware(compiler, {
publicPath: config.output.publicPath
}));
app.listen(3000, function () {
console.log('Example app listening on port 3000!\n');
});
Enabling Hot Module Replacement (HMR)
This code sample shows how to enable Hot Module Replacement (HMR) in conjunction with webpack-dev-middleware. It requires an additional package, webpack-hot-middleware, to work.
const webpackHotMiddleware = require('webpack-hot-middleware');
app.use(webpackDevMiddleware(compiler, {
publicPath: config.output.publicPath
}));
app.use(webpackHotMiddleware(compiler));
BrowserSync is a package that allows for live reloading of web pages as files are edited and saved. It can be integrated with webpack using browser-sync-webpack-plugin. Unlike webpack-dev-middleware, BrowserSync is focused on synchronizing interactions across multiple devices/browsers during testing.
Serve is a static file serving and directory listing package that can be used for quick prototyping and local development. It does not have built-in webpack integration or HMR, but it's a simple alternative for serving static files.
webpack-hot-server-middleware is similar to webpack-dev-middleware but is specifically designed for use with server-side rendering in Node.js applications. It works in tandem with webpack-dev-middleware and webpack-hot-middleware to enable HMR for server-rendered apps.
webpack-serve is a now-deprecated package that was once an alternative to webpack-dev-middleware. It provided a development server that used webpack's watch mode to observe file changes and recompile automatically. It has since been replaced by webpack-dev-server, which offers similar functionality.
An express-style development middleware for use with webpack bundles and allows for serving of the files emitted from webpack. This should be used for development only.
Some of the benefits of using this middleware include:
First thing's first, install the module:
npm install webpack-dev-middleware --save-dev
[!WARNING]
We do not recommend installing this module globally.
const webpack = require("webpack");
const middleware = require("webpack-dev-middleware");
const compiler = webpack({
// webpack options
});
const express = require("express");
const app = express();
app.use(
middleware(compiler, {
// webpack-dev-middleware options
}),
);
app.listen(3000, () => console.log("Example app listening on port 3000!"));
See below for an example of use with fastify.
Name | Type | Default | Description |
---|---|---|---|
methods | Array | [ 'GET', 'HEAD' ] | Allows to pass the list of HTTP request methods accepted by the middleware |
headers | Array|Object|Function | undefined | Allows to pass custom HTTP headers on each request. |
index | boolean|string | index.html | If false (but not undefined ), the server will not respond to requests to the root URL. |
mimeTypes | Object | undefined | Allows to register custom mime types or extension mappings. |
mimeTypeDefault | string | undefined | Allows to register a default mime type when we can't determine the content type. |
etag | boolean| "weak"| "strong" | undefined | Enable or disable etag generation. |
lastModified | boolean | undefined | Enable or disable Last-Modified header. Uses the file system's last modified value. |
cacheControl | boolean|number|string|Object | undefined | Enable or disable setting Cache-Control response header. |
cacheImmutable | boolean\ | undefined | Enable or disable setting Cache-Control: public, max-age=31536000, immutable response header for immutable assets. |
publicPath | string | undefined | The public path that the middleware is bound to. |
stats | boolean|string|Object | stats (from a configuration) | Stats options object or preset name. |
serverSideRender | boolean | undefined | Instructs the module to enable or disable the server-side rendering mode. |
writeToDisk | boolean|Function | false | Instructs the module to write files to the configured location on disk as specified in your webpack configuration. |
outputFileSystem | Object | memfs | Set the default file system which will be used by webpack as primary destination of generated files. |
modifyResponseData | Function | undefined | Allows to set up a callback to change the response data. |
The middleware accepts an options
Object. The following is a property reference for the Object.
Type: Array
Default: [ 'GET', 'HEAD' ]
This property allows a user to pass the list of HTTP request methods accepted by the middleware**.
Type: Array|Object|Function
Default: undefined
This property allows a user to pass custom HTTP headers on each request.
eg. { "X-Custom-Header": "yes" }
or
webpackDevMiddleware(compiler, {
headers: () => {
return {
"Last-Modified": new Date(),
};
},
});
or
webpackDevMiddleware(compiler, {
headers: (req, res, context) => {
res.setHeader("Last-Modified", new Date());
},
});
or
webpackDevMiddleware(compiler, {
headers: [
{
key: "X-custom-header",
value: "foo",
},
{
key: "Y-custom-header",
value: "bar",
},
],
});
or
webpackDevMiddleware(compiler, {
headers: () => [
{
key: "X-custom-header",
value: "foo",
},
{
key: "Y-custom-header",
value: "bar",
},
],
});
Type: Boolean|String
Default: index.html
If false
(but not undefined
), the server will not respond to requests to the root URL.
Type: Object
Default: undefined
This property allows a user to register custom mime types or extension mappings.
eg. mimeTypes: { phtml: 'text/html' }
.
Please see the documentation for mime-types
for more information.
Type: String
Default: undefined
This property allows a user to register a default mime type when we can't determine the content type.
Type: "weak" | "strong"
Default: undefined
Enable or disable etag generation. Boolean value use
Type: Boolean
Default: undefined
Enable or disable Last-Modified
header. Uses the file system's last modified value.
Type: Boolean | Number | String | { maxAge?: number, immutable?: boolean }
Default: undefined
Depending on the setting, the following headers will be generated:
Boolean
- Cache-Control: public, max-age=31536000000
Number
- Cache-Control: public, max-age=YOUR_NUMBER
String
- Cache-Control: YOUR_STRING
{ maxAge?: number, immutable?: boolean }
- Cache-Control: public, max-age=YOUR_MAX_AGE_or_31536000000
, also , immutable
can be added if you set the immutable
option to true
Enable or disable setting Cache-Control
response header.
Type: Boolean
Default: undefined
Enable or disable setting Cache-Control: public, max-age=31536000, immutable
response header for immutable assets (i.e. asset with a hash like image.a4c12bde.jpg
).
Immutable assets are assets that have their hash in the file name therefore they can be cached, because if you change their contents the file name will be changed.
Take preference over the cacheControl
option if the asset was defined as immutable.
Type: String
Default: output.publicPath
(from a configuration)
The public path that the middleware is bound to.
Best Practice: use the same publicPath
defined in your webpack config. For more information about publicPath
, please see the webpack documentation.
Type: Boolean|String|Object
Default: stats
(from a configuration)
Stats options object or preset name.
Type: Boolean
Default: undefined
Instructs the module to enable or disable the server-side rendering mode. Please see Server-Side Rendering for more information.
Type: Boolean|Function
Default: false
If true
, the option will instruct the module to write files to the configured location on disk as specified in your webpack
config file.
Setting writeToDisk: true
won't change the behavior of the webpack-dev-middleware
, and bundle files accessed through the browser will still be served from memory.
This option provides the same capabilities as the WriteFilePlugin
.
This option also accepts a Function
value, which can be used to filter which files are written to disk.
The function follows the same premise as Array#filter
in which a return value of false
will not write the file, and a return value of true
will write the file to disk. eg.
const webpack = require("webpack");
const configuration = {
/* Webpack configuration */
};
const compiler = webpack(configuration);
middleware(compiler, {
writeToDisk: (filePath) => {
return /superman\.css$/.test(filePath);
},
});
Type: Object
Default: memfs
Set the default file system which will be used by webpack as primary destination of generated files. This option isn't affected by the writeToDisk option.
You have to provide .join()
and mkdirp
method to the outputFileSystem
instance manually for compatibility with webpack@4
.
This can be done simply by using path.join
:
const webpack = require("webpack");
const path = require("path");
const myOutputFileSystem = require("my-fs");
const mkdirp = require("mkdirp");
myOutputFileSystem.join = path.join.bind(path); // no need to bind
myOutputFileSystem.mkdirp = mkdirp.bind(mkdirp); // no need to bind
const compiler = webpack({
/* Webpack configuration */
});
middleware(compiler, { outputFileSystem: myOutputFileSystem });
Allows to set up a callback to change the response data.
const webpack = require("webpack");
const configuration = {
/* Webpack configuration */
};
const compiler = webpack(configuration);
middleware(compiler, {
// Note - if you send the `Range` header you will have `ReadStream`
// Also `data` can be `string` or `Buffer`
modifyResponseData: (req, res, data, byteLength) => {
// Your logic
// Don't use `res.end()` or `res.send()` here
return { data, byteLength };
},
});
webpack-dev-middleware
also provides convenience methods that can be use to
interact with the middleware at runtime:
close(callback)
Instructs webpack-dev-middleware
instance to stop watching for file changes.
callback
Type: Function
Required: No
A function executed once the middleware has stopped watching.
const express = require("express");
const webpack = require("webpack");
const compiler = webpack({
/* Webpack configuration */
});
const middleware = require("webpack-dev-middleware");
const instance = middleware(compiler);
const app = new express();
app.use(instance);
setTimeout(() => {
// Says `webpack` to stop watch changes
instance.close();
}, 1000);
invalidate(callback)
Instructs webpack-dev-middleware
instance to recompile the bundle, e.g. after a change to the configuration.
callback
Type: Function
Required: No
A function executed once the middleware has invalidated.
const express = require("express");
const webpack = require("webpack");
const compiler = webpack({
/* Webpack configuration */
});
const middleware = require("webpack-dev-middleware");
const instance = middleware(compiler);
const app = new express();
app.use(instance);
setTimeout(() => {
// After a short delay the configuration is changed and a banner plugin is added to the config
new webpack.BannerPlugin("A new banner").apply(compiler);
// Recompile the bundle with the banner plugin:
instance.invalidate();
}, 1000);
waitUntilValid(callback)
Executes a callback function when the compiler bundle is valid, typically after compilation.
callback
Type: Function
Required: No
A function executed when the bundle becomes valid. If the bundle is valid at the time of calling, the callback is executed immediately.
const express = require("express");
const webpack = require("webpack");
const compiler = webpack({
/* Webpack configuration */
});
const middleware = require("webpack-dev-middleware");
const instance = middleware(compiler);
const app = new express();
app.use(instance);
instance.waitUntilValid(() => {
console.log("Package is in a valid state");
});
getFilenameFromUrl(url)
Get filename from URL.
url
Type: String
Required: Yes
URL for the requested file.
const express = require("express");
const webpack = require("webpack");
const compiler = webpack({
/* Webpack configuration */
});
const middleware = require("webpack-dev-middleware");
const instance = middleware(compiler);
const app = new express();
app.use(instance);
instance.waitUntilValid(() => {
const filename = instance.getFilenameFromUrl("/bundle.js");
console.log(`Filename is ${filename}`);
});
Since output.publicPath
and output.filename
/output.chunkFilename
can be dynamic, it's not possible to know which files are webpack bundles (and they public paths) and which are not, so we can't avoid blocking requests.
But there is a solution to avoid it - mount the middleware to a non-root route, for example:
const webpack = require("webpack");
const middleware = require("webpack-dev-middleware");
const compiler = webpack({
// webpack options
});
const express = require("express");
const app = express();
// Mounting the middleware to the non-root route allows avoids this.
// Note - check your public path, if you want to handle `/dist/`, you need to setup `output.publicPath` to `/` value.
app.use(
"/dist/",
middleware(compiler, {
// webpack-dev-middleware options
}),
);
app.listen(3000, () => console.log("Example app listening on port 3000!"));
Note: this feature is experimental and may be removed or changed completely in the future.
In order to develop an app using server-side rendering, we need access to the
stats
, which is
generated with each build.
With server-side rendering enabled, webpack-dev-middleware
sets the stats
to res.locals.webpack.devMiddleware.stats
and the filesystem to res.locals.webpack.devMiddleware.outputFileSystem
before invoking the next middleware,
allowing a developer to render the page body and manage the response to clients.
Note: Requests for bundle files will still be handled by
webpack-dev-middleware
and all requests will be pending until the build
process is finished with server-side rendering enabled.
Example Implementation:
const express = require("express");
const webpack = require("webpack");
const compiler = webpack({
/* Webpack configuration */
});
const isObject = require("is-object");
const middleware = require("webpack-dev-middleware");
const app = new express();
// This function makes server rendering of asset references consistent with different webpack chunk/entry configurations
function normalizeAssets(assets) {
if (isObject(assets)) {
return Object.values(assets);
}
return Array.isArray(assets) ? assets : [assets];
}
app.use(middleware(compiler, { serverSideRender: true }));
// The following middleware would not be invoked until the latest build is finished.
app.use((req, res) => {
const { devMiddleware } = res.locals.webpack;
const outputFileSystem = devMiddleware.outputFileSystem;
const jsonWebpackStats = devMiddleware.stats.toJson();
const { assetsByChunkName, outputPath } = jsonWebpackStats;
// Then use `assetsByChunkName` for server-side rendering
// For example, if you have only one main chunk:
res.send(`
<html>
<head>
<title>My App</title>
<style>
${normalizeAssets(assetsByChunkName.main)
.filter((path) => path.endsWith(".css"))
.map((path) => outputFileSystem.readFileSync(path.join(outputPath, path)))
.join("\n")}
</style>
</head>
<body>
<div id="root"></div>
${normalizeAssets(assetsByChunkName.main)
.filter((path) => path.endsWith(".js"))
.map((path) => `<script src="${path}"></script>`)
.join("\n")}
</body>
</html>
`);
});
We do our best to keep Issues in the repository focused on bugs, features, and needed modifications to the code for the module. Because of that, we ask users with general support, "how-to", or "why isn't this working" questions to try one of the other support channels that are available.
Your first-stop-shop for support for webpack-dev-server should by the excellent documentation for the module. If you see an opportunity for improvement of those docs, please head over to the webpack.js.org repo and open a pull request.
From there, we encourage users to visit the webpack discussions and
talk to the fine folks there. If your quest for answers comes up dry in chat,
head over to StackOverflow and do a quick search or open a new
question. Remember; It's always much easier to answer questions that include your
webpack.config.js
and relevant files!
If you're twitter-savvy you can tweet #webpack with your question and someone should be able to reach out and lend a hand.
If you have discovered a :bug:, have a feature suggestion, or would like to see a modification, please feel free to create an issue on Github. Note: The issue template isn't optional, so please be sure not to remove it, and please fill it out completely.
Examples of use with other servers will follow here.
const connect = require("connect");
const http = require("http");
const webpack = require("webpack");
const webpackConfig = require("./webpack.config.js");
const devMiddleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
const app = connect();
app.use(devMiddleware(compiler, devMiddlewareOptions));
http.createServer(app).listen(3000);
const http = require("http");
const Router = require("router");
const finalhandler = require("finalhandler");
const webpack = require("webpack");
const webpackConfig = require("./webpack.config.js");
const devMiddleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
const router = Router();
router.use(devMiddleware(compiler, devMiddlewareOptions));
var server = http.createServer((req, res) => {
router(req, res, finalhandler(req, res));
});
server.listen(3000);
const express = require("express");
const webpack = require("webpack");
const webpackConfig = require("./webpack.config.js");
const devMiddleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
const app = express();
app.use(devMiddleware(compiler, devMiddlewareOptions));
app.listen(3000, () => console.log("Example app listening on port 3000!"));
const Koa = require("koa");
const webpack = require("webpack");
const webpackConfig = require("./webpack.simple.config");
const middleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
const app = new Koa();
app.use(middleware.koaWrapper(compiler, devMiddlewareOptions));
app.listen(3000);
const Hapi = require("@hapi/hapi");
const webpack = require("webpack");
const webpackConfig = require("./webpack.config.js");
const devMiddleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {};
(async () => {
const server = Hapi.server({ port: 3000, host: "localhost" });
await server.register({
plugin: devMiddleware.hapiPlugin(),
options: {
// The `compiler` option is required
compiler,
...devMiddlewareOptions,
},
});
await server.start();
console.log("Server running on %s", server.info.uri);
})();
process.on("unhandledRejection", (err) => {
console.log(err);
process.exit(1);
});
Fastify interop will require the use of fastify-express
instead of middie
for providing middleware support. As the authors of fastify-express
recommend, this should only be used as a stopgap while full Fastify support is worked on.
const fastify = require("fastify")();
const webpack = require("webpack");
const webpackConfig = require("./webpack.config.js");
const devMiddleware = require("webpack-dev-middleware");
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
(async () => {
await fastify.register(require("@fastify/express"));
await fastify.use(devMiddleware(compiler, devMiddlewareOptions));
await fastify.listen(3000);
})();
import webpack from "webpack";
import { serve } from "@hono/node-server";
import { Hono } from "hono";
import devMiddleware from "webpack-dev-middleware";
import webpackConfig from "./webpack.config.js";
const compiler = webpack(webpackConfig);
const devMiddlewareOptions = {
/** Your webpack-dev-middleware-options */
};
const app = new Hono();
app.use(devMiddleware.honoWrapper(compiler, devMiddlewareOptions));
serve(app);
Please take a moment to read our contributing guidelines if you haven't yet done so.
FAQs
A development middleware for webpack
The npm package webpack-dev-middleware receives a total of 16,806,740 weekly downloads. As such, webpack-dev-middleware popularity was classified as popular.
We found that webpack-dev-middleware demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.