wix-style-processor
Advanced tools
Comparing version 2.0.12 to 3.0.0
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var lodash_1 = require("lodash"); | ||
exports.default = { | ||
extractStyles: function () { | ||
return lodash_1.map(document.querySelectorAll('style:not([wix-style]):not([data-computed])'), function (style) { return style.textContent.split('\n').join(' '); }).join(' '); | ||
}, | ||
getAllStyleTags: function () { | ||
return document.querySelectorAll('style:not([wix-style])'); | ||
}, | ||
overrideStyles: function (css) { | ||
lodash_1.each(document.querySelectorAll('style[data-computed=true]'), function (item) { return item.parentNode.removeChild(item); }); | ||
var style = document.createElement("style"); | ||
style.setAttribute('data-computed', 'true'); | ||
style.appendChild(document.createTextNode(css)); | ||
if (document.querySelector('style[wix-style]')) { | ||
(document.head || document.getElementsByTagName('head')[0]).insertBefore(style, document.querySelector('style[wix-style]')); | ||
} | ||
else { | ||
(document.head || document.getElementsByTagName('head')[0]).appendChild(style); | ||
} | ||
}, | ||
overrideStyle: function (tag, css) { | ||
tag.originalTemplate = tag.originalTemplate || tag.textContent; | ||
tag.textContent = css; | ||
}, | ||
isCssVarsSupported: function () { | ||
return !!(window.CSS && window.CSS.supports && window.CSS.supports('--fake-var', 0)); | ||
}, | ||
updateCssVars: function (varMap) { | ||
Object.keys(varMap).forEach(function (key) { | ||
document.documentElement.style.setProperty(key, varMap[key]); | ||
}); | ||
} | ||
}; | ||
//# sourceMappingURL=domService.js.map |
@@ -7,2 +7,3 @@ declare var expect: Function; | ||
Wix: any; | ||
CSS: {supports: Function} | ||
styleProcessor?: any; | ||
@@ -9,0 +10,0 @@ changeStyles?: any; |
@@ -12,16 +12,18 @@ "use strict"; | ||
var _this = this; | ||
if (options === void 0) { options = {}; } | ||
if (domServiceOverride === void 0) { domServiceOverride = domService_1.default; } | ||
Object.keys(defaultPlugins_1.defaultPlugins).forEach(function (funcName) { return _this.plugins.addCssFunction(funcName, defaultPlugins_1.defaultPlugins[funcName]); }); | ||
options = setDefaultOptions(options, this.plugins); | ||
var wixService = wixService_1.default(window.Wix); | ||
Object.keys(defaultPlugins_1.defaultPlugins) | ||
.forEach(function (funcName) { return _this.plugins.addCssFunction(funcName, defaultPlugins_1.defaultPlugins[funcName]); }); | ||
var defaultOptions = {}; | ||
defaultOptions.plugins = this.plugins; | ||
defaultOptions.shouldUseCssVars = domService_1.default.isCssVarsSupported() && (wixService.isEditorMode() || wixService.isPreviewMode()); | ||
options = Object.assign({}, defaultOptions, options); | ||
var styleUpdater = styleUpdater_1.default(wixService, domServiceOverride, options); | ||
wixService.listenToStyleParamsChange(function () { return styleUpdater.update(); }); | ||
if (wixService.isEditorMode() || wixService.isPreviewMode()) { | ||
wixService.listenToStyleParamsChange(function () { return styleUpdater.update(true); }); | ||
} | ||
return styleUpdater.update(); | ||
} | ||
}; | ||
function setDefaultOptions(options, plugins) { | ||
options = options || {}; | ||
options.plugins = options.plugins || plugins; | ||
return options; | ||
} | ||
//# sourceMappingURL=index.js.map |
@@ -44,5 +44,5 @@ "use strict"; | ||
} | ||
return function (tpaParams) { return fn.apply(void 0, args.concat([tpaParams])); }; | ||
return function (tpaParams) { return fn.apply(void 0, args.map(function (fn) { return fn(tpaParams); }).concat([tpaParams])); }; | ||
}; | ||
} | ||
//# sourceMappingURL=plugins.js.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var utils_1 = require("./utils"); | ||
var hash_1 = require("./hash"); | ||
var paramsRegex = /,(?![^(]*(?:\)|}))/g; | ||
var customSyntaxRegex = /"\w+\([^"]*\)"/g; | ||
function processor(_a, plugins) { | ||
var declaration = _a.declaration, vars = _a.vars; | ||
var _b = utils_1.splitDeclaration(declaration), key = _b.key, value = _b.value; | ||
if (plugins.declarationReplacers.length > 0) { | ||
plugins.declarationReplacers.forEach(function (plugin) { | ||
var pluginResult = plugin(key, value); | ||
key = pluginResult.key; | ||
value = pluginResult.value; | ||
}); | ||
function processor(_a, _b) { | ||
var part = _a.part, customSyntaxHelper = _a.customSyntaxHelper, tpaParams = _a.tpaParams, cacheMap = _a.cacheMap; | ||
var plugins = _b.plugins, shouldUseCssVars = _b.shouldUseCssVars; | ||
if (plugins.isSupportedFunction(part)) { | ||
var evaluationFunc = executeFunction(part, plugins, customSyntaxHelper); | ||
if (shouldUseCssVars) { | ||
var partHash = "--" + hash_1.hash(part); | ||
cacheMap[partHash] = evaluationFunc; | ||
return "var(" + partHash + ")"; | ||
} | ||
else { | ||
return evaluationFunc(tpaParams); | ||
} | ||
} | ||
var newValue = value.replace(customSyntaxRegex, function (part) { | ||
if (plugins.isSupportedFunction(part)) { | ||
return executeFunction(part, plugins, vars); | ||
} | ||
return part; | ||
}); | ||
return key + ': ' + newValue; | ||
return part; | ||
} | ||
exports.processor = processor; | ||
function executeFunction(value, plugins, vars) { | ||
function executeFunction(value, plugins, customSyntaxHelper) { | ||
var functionSignature; | ||
if (functionSignature = plugins.getFunctionSignature(value)) { | ||
return (_a = plugins.cssFunctions)[functionSignature.funcName].apply(_a, functionSignature.args.split(paramsRegex) | ||
.map(function (v) { return executeFunction(v.trim(), plugins, vars); }))(vars.tpaParams); | ||
.map(function (v) { return executeFunction(v.trim(), plugins, customSyntaxHelper); })); | ||
} | ||
else { | ||
return getVarOrPrimitiveValue(value, plugins, vars); | ||
return getVarOrPrimitiveValue(value, plugins, customSyntaxHelper); | ||
} | ||
var _a; | ||
} | ||
function getVarOrPrimitiveValue(varName, plugins, vars) { | ||
function getVarOrPrimitiveValue(varName, plugins, customSyntaxHelper) { | ||
if (utils_1.isCssVar(varName)) { | ||
varName = vars.getValue(varName); | ||
if (plugins.isSupportedFunction(varName)) { | ||
varName = executeFunction(varName, plugins, vars); | ||
var varValue_1 = customSyntaxHelper.getValue(varName); | ||
var defaultVarValue = void 0; | ||
if (plugins.isSupportedFunction(varValue_1)) { | ||
defaultVarValue = executeFunction(varValue_1, plugins, customSyntaxHelper); | ||
} | ||
else { | ||
defaultVarValue = function () { return varValue_1; }; | ||
} | ||
return getDefaultValueOrValueFromSettings(varName, defaultVarValue); | ||
} | ||
return varName; | ||
return function () { return varName; }; | ||
} | ||
function getDefaultValueOrValueFromSettings(varName, defaultVarValue) { | ||
return function (tpaParams) { | ||
var varNameInSettings = varName.substring(2, varName.length); | ||
if (tpaParams.strings[varNameInSettings] && tpaParams.strings[varNameInSettings].value) { | ||
return tpaParams.strings[varNameInSettings].value; | ||
} | ||
else if (tpaParams.colors[varNameInSettings]) { | ||
return tpaParams.colors[varNameInSettings]; | ||
} | ||
else if (tpaParams.fonts[varNameInSettings]) { | ||
return tpaParams.fonts[varNameInSettings]; | ||
} | ||
else if (tpaParams.numbers[varNameInSettings]) { | ||
return tpaParams.numbers[varNameInSettings]; | ||
} | ||
//not found in settings | ||
return defaultVarValue(tpaParams); | ||
}; | ||
} | ||
//# sourceMappingURL=processor.js.map |
@@ -8,9 +8,11 @@ "use strict"; | ||
var processor_1 = require("./processor"); | ||
var varsResolver_1 = require("./varsResolver"); | ||
exports.default = function (wixService, domService, options) { return ({ | ||
update: function () { | ||
return wixService.getStyleParams().then(function (_a) { | ||
var siteColors = _a[0], siteTextPresets = _a[1], styleParams = _a[2]; | ||
domService.getAllStyleTags().forEach(function (fillVars) { | ||
var css = (fillVars.originalTemplate || fillVars.textContent); | ||
var customSyntaxHelper_1 = require("./customSyntaxHelper"); | ||
var utils_1 = require("./utils"); | ||
exports.default = function (wixService, domService, options) { | ||
var cacheMap = {}; | ||
return { | ||
update: function (isRerender) { | ||
if (isRerender === void 0) { isRerender = false; } | ||
return wixService.getStyleParams().then(function (_a) { | ||
var siteColors = _a[0], siteTextPresets = _a[1], styleParams = _a[2]; | ||
var isStringHack = function (fontParam) { return fontParam.fontStyleParam === false; }; | ||
@@ -24,36 +26,64 @@ var isValidFontParam = function (fontParam) { return fontParam.family !== undefined; }; | ||
var strings = lodash_1.pickBy(styleParams.fonts, isStringHack); | ||
var stylis = new Stylis({ semicolon: false, compress: false, preserve: true }); | ||
var vars = new varsResolver_1.VarsResolver({ | ||
colors: colors, | ||
fonts: fonts, | ||
numbers: numbers, | ||
strings: strings, | ||
}); | ||
extractVars(css, vars.extractVar.bind(vars), stylis); | ||
stylis.use(function (context, declaration) { | ||
if (context === 1) { | ||
return processor_1.processor({ | ||
declaration: declaration, | ||
vars: vars | ||
}, options.plugins); | ||
} | ||
}); | ||
var newCss = stylis('', css); | ||
domService.overrideStyle(fillVars, newCss); | ||
var tpaParams = { colors: colors, fonts: fonts, numbers: numbers, strings: strings }; | ||
if (!isRerender || !options.shouldUseCssVars) { | ||
domService.getAllStyleTags().forEach(function (tagStyle) { | ||
var css = (tagStyle.originalTemplate || tagStyle.textContent); | ||
var stylis = new Stylis({ semicolon: false, compress: false, preserve: true }); | ||
applyDeclarationReplacers(options.plugins, stylis); | ||
applyCssFunctionsExtraction({ tpaParams: tpaParams, cacheMap: cacheMap, options: options }, stylis); | ||
var newCss = stylis('', css); | ||
domService.overrideStyle(tagStyle, newCss); | ||
}); | ||
} | ||
if (options.shouldUseCssVars) { | ||
var varMap = Object.keys(cacheMap).reduce(function (varMap, key) { | ||
varMap[key] = cacheMap[key](tpaParams); | ||
return varMap; | ||
}, {}); | ||
domService.updateCssVars(varMap); | ||
} | ||
}).catch(function (err) { | ||
console.error('failed updating styles', err); | ||
throw err; | ||
}); | ||
}).catch(function (err) { | ||
console.error('failed updating styles', err); | ||
throw err; | ||
} | ||
}; | ||
}; | ||
function escapeRegExp(str) { | ||
return str.replace(/[\-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, '\\$&'); | ||
} | ||
function applyDeclarationReplacers(plugins, stylis) { | ||
plugins.declarationReplacers | ||
.forEach(function (replacer) { | ||
stylis.use(function (context, declaration) { | ||
if (context == 1) { | ||
var _a = utils_1.splitDeclaration(declaration), key = _a.key, value = _a.value; | ||
var pluginResult = replacer(key, value); | ||
return pluginResult.key + ": " + pluginResult.value; | ||
} | ||
}); | ||
} | ||
}); }; | ||
function extractVars(css, extractVarsFn, stylis) { | ||
stylis.use(function (context, decleration) { | ||
}); | ||
} | ||
function applyCssFunctionsExtraction(_a, stylis) { | ||
var tpaParams = _a.tpaParams, cacheMap = _a.cacheMap, options = _a.options; | ||
var customSyntaxHelper = new customSyntaxHelper_1.CustomSyntaxHelper(); | ||
stylis.use(function (context, content) { | ||
if (context === 1) { | ||
extractVarsFn(decleration); | ||
/* for each declaration */ | ||
var _a = utils_1.splitDeclaration(content), key = _a.key, value = _a.value; | ||
customSyntaxHelper.extractVar(key, value); | ||
customSyntaxHelper.extractCustomSyntax(key, value); | ||
return key + ": " + value; | ||
} | ||
if (context === -2) { | ||
/* post-process */ | ||
return customSyntaxHelper.customSyntaxStrs.reduce(function (content, part) { | ||
var newValue = processor_1.processor({ | ||
part: part, customSyntaxHelper: customSyntaxHelper, tpaParams: tpaParams, cacheMap: cacheMap | ||
}, options); | ||
return content.replace(new RegExp(escapeRegExp(part), 'g'), newValue); | ||
}, content); | ||
} | ||
}); | ||
stylis('', css); | ||
stylis.use(null); | ||
} | ||
//# sourceMappingURL=styleUpdater.js.map |
@@ -16,2 +16,8 @@ "use strict"; | ||
Wix.addEventListener(Wix.Events.SETTINGS_UPDATED, cb); | ||
}, | ||
isEditorMode: function () { | ||
return Wix.Utils.getViewMode() === 'editor'; | ||
}, | ||
isPreviewMode: function () { | ||
return Wix.Utils.getViewMode() === 'preview'; | ||
} | ||
@@ -18,0 +24,0 @@ }); }; |
@@ -6,3 +6,4 @@ "use strict"; | ||
var _this = this; | ||
this.queue = []; | ||
this.viewMode = 'standalone'; | ||
this.callbackQ = []; | ||
this.Styles = { | ||
@@ -19,2 +20,5 @@ getSiteColors: function (cb) { | ||
}; | ||
this.Utils = { | ||
getViewMode: function () { return _this.viewMode; } | ||
}; | ||
this.Events = { | ||
@@ -32,2 +36,11 @@ STYLE_PARAMS_CHANGE: 'style_params_change' | ||
_this.styleParams = styleParams; | ||
}, | ||
viewMode: function (mode) { | ||
_this.viewMode = mode; | ||
return _this; | ||
}, | ||
siteColor: function (ref, value) { | ||
_this.siteColors.filter(function (color) { return color.reference === ref; }) | ||
.map(function (c) { return c.value = value; }); | ||
return _this; | ||
} | ||
@@ -37,3 +50,3 @@ }; | ||
updateStyleParams: function () { | ||
var cb = _this.queue.shift(); | ||
var cb = _this.callbackQ.shift(); | ||
if (cb) { | ||
@@ -46,3 +59,3 @@ return cb(); | ||
WixMock.prototype.addEventListener = function (eventName, cb) { | ||
this.queue.push(cb); | ||
this.callbackQ.push(cb); | ||
}; | ||
@@ -49,0 +62,0 @@ return WixMock; |
@@ -7,2 +7,3 @@ "use strict"; | ||
var index_1 = require("../../src/index"); | ||
window.Wix.Utils.getViewMode = function () { return 'editor'; }; | ||
if (window.name !== 'E2E') { | ||
@@ -9,0 +10,0 @@ index_1.default.init({}); |
@@ -5,3 +5,3 @@ { | ||
"description": "An alternative Wix Styles TPA processor", | ||
"version": "2.0.12", | ||
"version": "3.0.0", | ||
"author": { | ||
@@ -8,0 +8,0 @@ "name": "Eran Shabi", |
@@ -88,3 +88,3 @@ # wix-style-processor | ||
#### Declaration Replacer plugins | ||
### 2. Declaration Replacer plugins | ||
These plugins allow you to replace the entire key / value of the CSS declaration. | ||
@@ -128,2 +128,16 @@ Since they're invoked upon each and every declaration, there's no need to name them. | ||
# Enhanced Editor mode | ||
This mode take leverage of native **css vars** to speed up the rendering of editor changes. | ||
This feature will be enabled only on browsers that [supports it](http://caniuse.com/#search=css-variables) and in `Editor / Preview` mode. | ||
#### Benchmarks | ||
Without css-vars usage: (the first line is a timing of first render) | ||
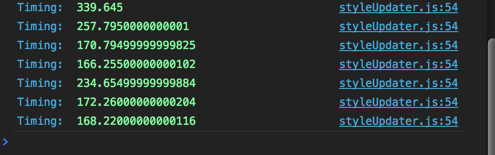 | ||
With css-vars usage: (the first line is a timing of first render) | ||
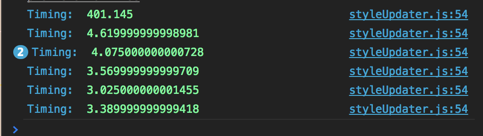 | ||
# Important | ||
@@ -130,0 +144,0 @@ This module only parses inline CSS. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
4204311
46
28461
145