Comparing version 5.0.0 to 6.0.0
"use strict"; | ||
function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.default = void 0; | ||
var _os = _interopRequireDefault(require("os")); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
@@ -11,3 +18,3 @@ | ||
function _possibleConstructorReturn(self, call) { if (call && (_typeof(call) === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
function _possibleConstructorReturn(self, call) { if (call && (typeof call === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
@@ -20,5 +27,5 @@ function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } | ||
function isNativeReflectConstruct() { if (typeof Reflect === "undefined" || !Reflect.construct) return false; if (Reflect.construct.sham) return false; if (typeof Proxy === "function") return true; try { Date.prototype.toString.call(Reflect.construct(Date, [], function () {})); return true; } catch (e) { return false; } } | ||
function _construct(Parent, args, Class) { if (_isNativeReflectConstruct()) { _construct = Reflect.construct; } else { _construct = function _construct(Parent, args, Class) { var a = [null]; a.push.apply(a, args); var Constructor = Function.bind.apply(Parent, a); var instance = new Constructor(); if (Class) _setPrototypeOf(instance, Class.prototype); return instance; }; } return _construct.apply(null, arguments); } | ||
function _construct(Parent, args, Class) { if (isNativeReflectConstruct()) { _construct = Reflect.construct; } else { _construct = function _construct(Parent, args, Class) { var a = [null]; a.push.apply(a, args); var Constructor = Function.bind.apply(Parent, a); var instance = new Constructor(); if (Class) _setPrototypeOf(instance, Class.prototype); return instance; }; } return _construct.apply(null, arguments); } | ||
function _isNativeReflectConstruct() { if (typeof Reflect === "undefined" || !Reflect.construct) return false; if (Reflect.construct.sham) return false; if (typeof Proxy === "function") return true; try { Boolean.prototype.valueOf.call(Reflect.construct(Boolean, [], function () {})); return true; } catch (e) { return false; } } | ||
@@ -31,17 +38,21 @@ function _isNativeFunction(fn) { return Function.toString.call(fn).indexOf("[native code]") !== -1; } | ||
var os = require('os'); // Create an Error able to contain some params and better stack traces | ||
var YError = | ||
/*#__PURE__*/ | ||
function (_Error) { | ||
/** | ||
* An YError class able to contain some params and | ||
* print better stack traces | ||
* @extends Error | ||
*/ | ||
let YError = /*#__PURE__*/function (_Error) { | ||
_inherits(YError, _Error); | ||
function YError(wrappedErrors, errorCode) { | ||
/** | ||
* Creates a new YError with an error code | ||
* and some params as debug values. | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
*/ | ||
function YError(wrappedErrors, errorCode, ...params) { | ||
var _this; | ||
for (var _len = arguments.length, params = new Array(_len > 2 ? _len - 2 : 0), _key = 2; _key < _len; _key++) { | ||
params[_key - 2] = arguments[_key]; | ||
} | ||
_classCallCheck(this, YError); | ||
@@ -75,3 +86,3 @@ | ||
return (this.wrappedErrors.length ? // eslint-disable-next-line | ||
this.wrappedErrors[this.wrappedErrors.length - 1].stack + os.EOL : '') + this.constructor.name + ': ' + this.code + ' (' + this.params.join(', ') + ')'; | ||
this.wrappedErrors[this.wrappedErrors.length - 1].stack + _os.default.EOL : '') + this.constructor.name + ': ' + this.code + ' (' + this.params.join(', ') + ')'; | ||
} | ||
@@ -81,11 +92,23 @@ }]); | ||
return YError; | ||
}(_wrapNativeSuper(Error)); // Wrap a classic error | ||
}(_wrapNativeSuper(Error)); | ||
/** | ||
* Wraps any error and output a YError with an error | ||
* code and some params as debug values. | ||
* @param {Error} err | ||
* The error to wrap | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.wrap = function yerrorWrap(err, errorCode) { | ||
var yError = null; | ||
YError.wrap = function yerrorWrap(err, errorCode, ...params) { | ||
let yError = null; | ||
var wrappedErrorIsACode = _looksLikeAYErrorCode(err.message); | ||
const wrappedErrorIsACode = _looksLikeAYErrorCode(err.message); | ||
var wrappedErrors = (err.wrappedErrors || []).concat(err); | ||
const wrappedErrors = (err.wrappedErrors || []).concat(err); | ||
@@ -100,6 +123,2 @@ if (!errorCode) { | ||
for (var _len2 = arguments.length, params = new Array(_len2 > 2 ? _len2 - 2 : 0), _key2 = 2; _key2 < _len2; _key2++) { | ||
params[_key2 - 2] = arguments[_key2]; | ||
} | ||
if (err.message && !wrappedErrorIsACode) { | ||
@@ -109,7 +128,20 @@ params.push(err.message); | ||
yError = _construct(YError, [wrappedErrors, errorCode].concat(params)); | ||
yError = new YError(wrappedErrors, errorCode, ...params); | ||
return yError; | ||
}; | ||
/** | ||
* Return a YError as is or wraps any other error and output | ||
* a YError with a code and some params as debug values. | ||
* @param {Error} err | ||
* The error to cast | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.cast = function yerrorCast(err) { | ||
YError.cast = function yerrorCast(err, ...params) { | ||
if (_looksLikeAYError(err)) { | ||
@@ -119,10 +151,20 @@ return err; | ||
for (var _len3 = arguments.length, params = new Array(_len3 > 1 ? _len3 - 1 : 0), _key3 = 1; _key3 < _len3; _key3++) { | ||
params[_key3 - 1] = arguments[_key3]; | ||
} | ||
return YError.wrap.apply(YError, [err].concat(params)); | ||
}; | ||
/** | ||
* Same than `YError.wrap()` but preserves the code | ||
* and the debug values of the error if it is | ||
* already an instance of the YError constructor. | ||
* @param {Error} err | ||
* The error to bump | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.bump = function yerrorBump(err) { | ||
YError.bump = function yerrorBump(err, ...params) { | ||
if (_looksLikeAYError(err)) { | ||
@@ -132,6 +174,2 @@ return YError.wrap.apply(YError, [err, err.code].concat(err.params)); | ||
for (var _len4 = arguments.length, params = new Array(_len4 > 1 ? _len4 - 1 : 0), _key4 = 1; _key4 < _len4; _key4++) { | ||
params[_key4 - 1] = arguments[_key4]; | ||
} | ||
return YError.wrap.apply(YError, [err].concat(params)); | ||
@@ -150,2 +188,4 @@ }; // In order to keep compatibility through major versions | ||
module.exports = YError; | ||
var _default = YError; | ||
exports.default = _default; | ||
//# sourceMappingURL=index.js.map |
"use strict"; | ||
var _assert = _interopRequireDefault(require("assert")); | ||
var _ = _interopRequireDefault(require(".")); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
/* eslint max-nested-callbacks:[0], no-magic-numbers:[0] */ | ||
var assert = require('assert'); | ||
describe('YError', () => { | ||
describe('.__constructor', () => { | ||
it('Should work', () => { | ||
var err = new _.default('E_ERROR', 'arg1', 'arg2'); | ||
(0, _assert.default)(err instanceof Error); | ||
var YError = require('./index.js'); | ||
_assert.default.equal(err.name, err.toString()); | ||
describe('YError', function () { | ||
describe('.__constructor', function () { | ||
it('Should work', function () { | ||
var err = new YError('E_ERROR', 'arg1', 'arg2'); | ||
assert(err instanceof Error); | ||
assert.equal(err.name, err.toString()); | ||
assert.equal(err.code, 'E_ERROR'); | ||
assert.deepEqual(err.params, ['arg1', 'arg2']); | ||
assert.equal(err.toString(), 'YError: E_ERROR (arg1, arg2)'); | ||
_assert.default.equal(err.code, 'E_ERROR'); | ||
_assert.default.deepEqual(err.params, ['arg1', 'arg2']); | ||
_assert.default.equal(err.toString(), 'YError: E_ERROR (arg1, arg2)'); | ||
}); | ||
it('Should work without code', function () { | ||
var err = new YError(); | ||
assert.equal(err.code, 'E_UNEXPECTED'); | ||
assert.deepEqual(err.params, []); | ||
assert.equal(err.toString(), 'YError: E_UNEXPECTED ()'); | ||
assert.equal(err.name, err.toString()); | ||
it('Should work without code', () => { | ||
var err = new _.default(); | ||
_assert.default.equal(err.code, 'E_UNEXPECTED'); | ||
_assert.default.deepEqual(err.params, []); | ||
_assert.default.equal(err.toString(), 'YError: E_UNEXPECTED ()'); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should work without new', function () { | ||
var err = new YError('E_ERROR', 'arg1', 'arg2'); | ||
assert.equal(err.code, 'E_ERROR'); | ||
assert(err instanceof YError); | ||
assert.deepEqual(err.params, ['arg1', 'arg2']); | ||
assert.equal(err.toString(), 'YError: E_ERROR (arg1, arg2)'); | ||
assert.equal(err.name, err.toString()); | ||
it('Should work without new', () => { | ||
var err = new _.default('E_ERROR', 'arg1', 'arg2'); | ||
_assert.default.equal(err.code, 'E_ERROR'); | ||
(0, _assert.default)(err instanceof _.default); | ||
_assert.default.deepEqual(err.params, ['arg1', 'arg2']); | ||
_assert.default.equal(err.toString(), 'YError: E_ERROR (arg1, arg2)'); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
}); | ||
describe('.wrap()', function () { | ||
it('Should work with standard errors and a message', function () { | ||
describe('.wrap()', () => { | ||
it('Should work with standard errors and a message', () => { | ||
// eslint-disable-line | ||
var err = YError.wrap(new Error('This is an error!')); | ||
assert.equal(err.code, 'E_UNEXPECTED'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, ['This is an error!']); | ||
var err = _.default.wrap(new Error('This is an error!')); | ||
_assert.default.equal(err.code, 'E_UNEXPECTED'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, ['This is an error!']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains cast error.'); | ||
assert.equal(err.name, err.toString()); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains cast error.'); | ||
_assert.default.equal(err.name, err.toString()); | ||
} | ||
}); | ||
it('Should work with standard errors and an error code', function () { | ||
var err = YError.wrap(new Error('E_ERROR')); | ||
assert.equal(err.code, 'E_ERROR'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, []); | ||
it('Should work with standard errors and an error code', () => { | ||
var err = _.default.wrap(new Error('E_ERROR')); | ||
_assert.default.equal(err.code, 'E_ERROR'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, []); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: E_ERROR'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR ()'), 'Stack contains cast error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: E_ERROR'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR ()'), 'Stack contains cast error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should work with standard errors, an error code and params', function () { | ||
var err = YError.wrap(new Error('E_ERROR'), 'E_ERROR_2', 'arg1', 'arg2'); | ||
assert.equal(err.code, 'E_ERROR_2'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, ['arg1', 'arg2']); | ||
it('Should work with standard errors, an error code and params', () => { | ||
var err = _.default.wrap(new Error('E_ERROR'), 'E_ERROR_2', 'arg1', 'arg2'); | ||
_assert.default.equal(err.code, 'E_ERROR_2'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, ['arg1', 'arg2']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: E_ERROR'), 'Stack contains first error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR_2 (arg1, arg2)'), 'Stack contains second error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: E_ERROR'), 'Stack contains first error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR_2 (arg1, arg2)'), 'Stack contains second error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should work with several wrapped errors', function () { | ||
var err = YError.wrap(YError.wrap(new Error('E_ERROR_1'), 'E_ERROR_2', 'arg2.1', 'arg2.2'), 'E_ERROR_3', 'arg3.1', 'arg3.2'); | ||
assert.equal(err.code, 'E_ERROR_3'); | ||
assert.equal(err.wrappedErrors.length, 2); | ||
assert.deepEqual(err.params, ['arg3.1', 'arg3.2']); | ||
it('Should work with several wrapped errors', () => { | ||
var err = _.default.wrap(_.default.wrap(new Error('E_ERROR_1'), 'E_ERROR_2', 'arg2.1', 'arg2.2'), 'E_ERROR_3', 'arg3.1', 'arg3.2'); | ||
_assert.default.equal(err.code, 'E_ERROR_3'); | ||
_assert.default.equal(err.wrappedErrors.length, 2); | ||
_assert.default.deepEqual(err.params, ['arg3.1', 'arg3.2']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: E_ERROR_1'), 'Stack contains first error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR_2 (arg2.1, arg2.2)'), 'Stack contains second error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR_3 (arg3.1, arg3.2)'), 'Stack contains second error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: E_ERROR_1'), 'Stack contains first error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR_2 (arg2.1, arg2.2)'), 'Stack contains second error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR_3 (arg3.1, arg3.2)'), 'Stack contains second error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
}); | ||
describe('.cast()', function () { | ||
it('Should work with standard errors and a message', function () { | ||
var err = YError.cast(new Error('This is an error!')); | ||
assert.equal(err.code, 'E_UNEXPECTED'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, ['This is an error!']); | ||
describe('.cast()', () => { | ||
it('Should work with standard errors and a message', () => { | ||
var err = _.default.cast(new Error('This is an error!')); | ||
_assert.default.equal(err.code, 'E_UNEXPECTED'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, ['This is an error!']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains cast error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains cast error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should let YError instances pass through', function () { | ||
var err = YError.cast(new YError('E_ERROR', 'arg1', 'arg2')); | ||
assert.equal(err.code, 'E_ERROR'); | ||
assert.deepEqual(err.params, ['arg1', 'arg2']); | ||
it('Should let YError instances pass through', () => { | ||
var err = _.default.cast(new _.default('E_ERROR', 'arg1', 'arg2')); | ||
_assert.default.equal(err.code, 'E_ERROR'); | ||
_assert.default.deepEqual(err.params, ['arg1', 'arg2']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR (arg1, arg2)'), 'Stack contains cast error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR (arg1, arg2)'), 'Stack contains cast error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
}); | ||
describe('.bump()', function () { | ||
it('Should work with standard errors and a message', function () { | ||
var err = YError.bump(new Error('This is an error!')); | ||
assert.equal(err.code, 'E_UNEXPECTED'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, ['This is an error!']); | ||
describe('.bump()', () => { | ||
it('Should work with standard errors and a message', () => { | ||
var err = _.default.bump(new Error('This is an error!')); | ||
_assert.default.equal(err.code, 'E_UNEXPECTED'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, ['This is an error!']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains bumped error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: This is an error!'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_UNEXPECTED (This is an error!)'), 'Stack contains bumped error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should work with YError like errors', function () { | ||
it('Should work with YError like errors', () => { | ||
var baseErr = new Error('E_A_NEW_ERROR'); | ||
baseErr.code = 'E_A_NEW_ERROR'; | ||
baseErr.params = ['baseParam1', 'baseParam2']; | ||
var err = YError.bump(baseErr); | ||
assert.equal(err.code, 'E_A_NEW_ERROR'); | ||
assert.equal(err.wrappedErrors.length, 1); | ||
assert.deepEqual(err.params, ['baseParam1', 'baseParam2']); | ||
let err = _.default.bump(baseErr); | ||
_assert.default.equal(err.code, 'E_A_NEW_ERROR'); | ||
_assert.default.equal(err.wrappedErrors.length, 1); | ||
_assert.default.deepEqual(err.params, ['baseParam1', 'baseParam2']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('Error: E_A_NEW_ERROR'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_A_NEW_ERROR (baseParam1, baseParam2)'), 'Stack contains bumped error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('Error: E_A_NEW_ERROR'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_A_NEW_ERROR (baseParam1, baseParam2)'), 'Stack contains bumped error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
it('Should work with Y errors and a message', function () { | ||
var err = YError.bump(new YError('E_ERROR', 'arg1.1', 'arg1.2'), 'E_ERROR_2', 'arg2.1', 'arg2.2'); | ||
assert.equal(err.code, 'E_ERROR'); | ||
assert.deepEqual(err.params, ['arg1.1', 'arg1.2']); | ||
it('Should work with Y errors and a message', () => { | ||
var err = _.default.bump(new _.default('E_ERROR', 'arg1.1', 'arg1.2'), 'E_ERROR_2', 'arg2.1', 'arg2.2'); | ||
_assert.default.equal(err.code, 'E_ERROR'); | ||
_assert.default.deepEqual(err.params, ['arg1.1', 'arg1.2']); | ||
if (Error.captureStackTrace) { | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR (arg1.1, arg1.2)'), 'Stack contains original error.'); | ||
assert(-1 !== err.stack.indexOf('YError: E_ERROR (arg1.1, arg1.2)'), 'Stack contains bumped error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR (arg1.1, arg1.2)'), 'Stack contains original error.'); | ||
(0, _assert.default)(-1 !== err.stack.indexOf('YError: E_ERROR (arg1.1, arg1.2)'), 'Stack contains bumped error.'); | ||
} | ||
assert.equal(err.name, err.toString()); | ||
_assert.default.equal(err.name, err.toString()); | ||
}); | ||
}); | ||
}); | ||
}); | ||
//# sourceMappingURL=index.mocha.js.map |
216
package.json
{ | ||
"name": "yerror", | ||
"version": "5.0.0", | ||
"version": "6.0.0", | ||
"description": "It helps to know why you got an error.", | ||
"main": "src/index.js", | ||
"types": "src/index.d.ts", | ||
"browser": "dist/index.js", | ||
"main": "dist/index", | ||
"module": "dist/index.mjs", | ||
"types": "dist/index.d.ts", | ||
"browser": "dist/index", | ||
"metapak": { | ||
"configs": [ | ||
"main", | ||
"readme", | ||
"eslint", | ||
"babel", | ||
"codeclimate", | ||
"mocha", | ||
"travis", | ||
"karma", | ||
"jsdocs" | ||
], | ||
"data": { | ||
"testsFiles": "src/*.mocha.js", | ||
"files": "src/*.js", | ||
"ignore": [ | ||
"dist" | ||
], | ||
"bundleFiles": [ | ||
"dist", | ||
"src" | ||
] | ||
} | ||
}, | ||
"scripts": { | ||
"compile": "babel --extensions '.js' src --out-dir=dist", | ||
"build": "rm -rf dist && npm run compile && npm run test:build", | ||
"cli": "env NPM_RUN_CLI=1", | ||
"changelog": "conventional-changelog -p angular -i CHANGELOG.md -s && git add CHANGELOG.md", | ||
"cli": "env NODE_ENV=${NODE_ENV:-cli}", | ||
"compile": "rimraf -f 'dist' && npm run compile:cjs && npm run compile:mjs", | ||
"compile:cjs": "babel --env-name=cjs --out-dir=dist --source-maps=true src", | ||
"compile:mjs": "babel --env-name=mjs --out-file-extension=.mjs --out-dir=dist --source-maps=true src", | ||
"cover": "nyc npm test && nyc report --reporter=html --reporter=text", | ||
"coveralls": "nyc npm test && nyc report --reporter=text-lcov | coveralls && rm -rf ./coverage", | ||
"cz": "env NODE_ENV=${NODE_ENV:-cli} git cz", | ||
"doc": "echo \"# API\" > API.md; jsdoc2md src/*.js >> API.md && git add API.md", | ||
"karma": "karma start karma.conf.js", | ||
"lint": "eslint src/*.js", | ||
"metapak": "metapak", | ||
"mocha": "mocha --require '@babel/register' src/*.mocha.js", | ||
"precz": "npm t && npm run lint && npm run metapak -- -s && npm run compile && npm run doc", | ||
"prettier": "prettier --write src/*.js", | ||
"preversion": "npm run lint && npm build && npm t", | ||
"test": "mocha src/*.mocha.js && karma start karma.conf.js", | ||
"test:build": "mocha dist/*.mocha.js" | ||
"preversion": "npm t && npm run lint && npm run metapak -- -s && npm run compile && npm run doc", | ||
"test": "npm run mocha && npm run karma", | ||
"test:build": "mocha dist/*.mocha.js", | ||
"version": "npm run changelog" | ||
}, | ||
"engines": { | ||
"node": ">=8.12.0" | ||
"node": ">=12.19.0" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git://github.com/SimpliField/yerror.git" | ||
"url": "git://github.com/nfroidure/yerror.git" | ||
}, | ||
@@ -39,47 +74,70 @@ "keywords": [ | ||
"bugs": { | ||
"url": "https://github.com/SimpliField/yerror/issues" | ||
"url": "https://github.com/nfroidure/yerror/issues" | ||
}, | ||
"devDependencies": { | ||
"@babel/cli": "^7.7.4", | ||
"@babel/core": "^7.7.4", | ||
"@babel/register": "^7.7.4", | ||
"@babel/preset-env": "^7.7.4", | ||
"@babel/cli": "^7.13.14", | ||
"@babel/core": "^7.13.15", | ||
"@babel/eslint-parser": "^7.13.14", | ||
"@babel/plugin-proposal-class-properties": "^7.7.4", | ||
"@babel/plugin-proposal-object-rest-spread": "^7.7.4", | ||
"@babel/plugin-proposal-object-rest-spread": "^7.13.8", | ||
"@babel/plugin-transform-classes": "^7.7.4", | ||
"babel-eslint": "^10.0.3", | ||
"@babel/preset-env": "^7.13.15", | ||
"@babel/register": "^7.13.14", | ||
"babel-plugin-transform-builtin-extend": "^1.1.2", | ||
"browserify": "^16.5.0", | ||
"coveralls": "^3.0.9", | ||
"eslint": "^6.7.2", | ||
"eslint-plugin-prettier": "^3.1.1", | ||
"karma": "^4.4.1", | ||
"commitizen": "^4.2.3", | ||
"conventional-changelog-cli": "^2.1.1", | ||
"coveralls": "^3.1.0", | ||
"cz-conventional-changelog": "^3.3.0", | ||
"eslint": "^7.23.0", | ||
"eslint-plugin-prettier": "^3.3.1", | ||
"jsdoc-to-markdown": "^7.0.1", | ||
"karma": "^6.3.2", | ||
"karma-browserify": "^6.1.0", | ||
"karma-chrome-launcher": "^3.1.0", | ||
"karma-firefox-launcher": "^1.2.0", | ||
"karma-mocha": "^1.3.0", | ||
"karma-firefox-launcher": "^2.1.0", | ||
"karma-mocha": "^2.0.1", | ||
"karma-sauce-launcher": "^2.0.2", | ||
"mocha": "^6.2.2", | ||
"nyc": "^14.1.1", | ||
"prettier": "^1.19.1" | ||
"metapak": "^4.0.0", | ||
"metapak-nfroidure": "11.0.6", | ||
"mocha": "^8.3.2", | ||
"nyc": "^15.1.0", | ||
"prettier": "^2.2.1" | ||
}, | ||
"dependencies": {}, | ||
"babel": { | ||
"presets": [ | ||
"@babel/env" | ||
], | ||
"plugins": [ | ||
[ | ||
"@babel/plugin-transform-classes", | ||
{} | ||
], | ||
[ | ||
"babel-plugin-transform-builtin-extend", | ||
{ | ||
"globals": [ | ||
"Error", | ||
"Array" | ||
] | ||
} | ||
] | ||
"contributors": [], | ||
"files": [ | ||
"dist", | ||
"src", | ||
"LICENSE", | ||
"README.md", | ||
"CHANGELOG.md" | ||
], | ||
"config": { | ||
"commitizen": { | ||
"path": "./node_modules/cz-conventional-changelog" | ||
} | ||
}, | ||
"greenkeeper": { | ||
"ignore": [ | ||
"commitizen", | ||
"cz-conventional-changelog", | ||
"conventional-changelog-cli", | ||
"eslint", | ||
"eslint-config-prettier", | ||
"prettier", | ||
"@babel/cli", | ||
"@babel/core", | ||
"@babel/register", | ||
"@babel/preset-env", | ||
"@babel/plugin-proposal-object-rest-spread", | ||
"babel-eslint", | ||
"mocha", | ||
"coveralls", | ||
"nyc", | ||
"karma", | ||
"karma-chrome-launcher", | ||
"karma-firefox-launcher", | ||
"karma-mocha", | ||
"jsdoc-to-markdown" | ||
] | ||
@@ -93,3 +151,3 @@ }, | ||
"ecmaVersion": 2018, | ||
"sourceType": "script", | ||
"sourceType": "module", | ||
"modules": true | ||
@@ -116,3 +174,69 @@ }, | ||
"proseWrap": "always" | ||
}, | ||
"babel": { | ||
"plugins": [ | ||
[ | ||
"@babel/plugin-proposal-object-rest-spread" | ||
], | ||
[ | ||
"@babel/plugin-transform-classes", | ||
{} | ||
], | ||
[ | ||
"babel-plugin-transform-builtin-extend", | ||
{ | ||
"globals": [ | ||
"Error", | ||
"Array" | ||
] | ||
} | ||
] | ||
], | ||
"presets": [ | ||
[ | ||
"@babel/env", | ||
{ | ||
"targets": { | ||
"node": "12.19.0" | ||
} | ||
} | ||
] | ||
], | ||
"env": { | ||
"cjs": { | ||
"presets": [ | ||
[ | ||
"@babel/env", | ||
{ | ||
"targets": { | ||
"node": "10" | ||
}, | ||
"modules": "commonjs" | ||
} | ||
] | ||
], | ||
"comments": true | ||
}, | ||
"mjs": { | ||
"presets": [ | ||
[ | ||
"@babel/env", | ||
{ | ||
"targets": { | ||
"node": "12" | ||
}, | ||
"modules": false | ||
} | ||
] | ||
], | ||
"comments": false | ||
} | ||
}, | ||
"sourceMaps": true | ||
}, | ||
"nyc": { | ||
"exclude": [ | ||
"src/*.mocha.js" | ||
] | ||
} | ||
} |
112
README.md
@@ -1,7 +0,22 @@ | ||
# YError | ||
> Better errors for your NodeJS code. | ||
[//]: # ( ) | ||
[//]: # (This file is automatically generated by a `metapak`) | ||
[//]: # (module. Do not change it except between the) | ||
[//]: # (`content:start/end` flags, your changes would) | ||
[//]: # (be overridden.) | ||
[//]: # ( ) | ||
# yerror | ||
> It helps to know why you got an error. | ||
[](https://github.com/nfroidure/yerror/blob/master/LICENSE) | ||
[](https://travis-ci.com/github/git://github.com/nfroidure/yerror.git) | ||
[](https://coveralls.io/github/git://github.com/nfroidure/yerror.git?branch=master) | ||
[](https://npmjs.org/package/yerror) | ||
[](https://david-dm.org/nfroidure/yerror) | ||
[](https://david-dm.org/nfroidure/yerror#info=devDependencies) | ||
[](https://packagequality.com/#?package=yerror) | ||
[](https://codeclimate.com/github/git://github.com/nfroidure/yerror.git) | ||
[](https://npmjs.org/package/yerror) [](https://travis-ci.org/nfroidure/yerror) [](https://david-dm.org/nfroidure/yerror) [](https://david-dm.org/nfroidure/yerror#info=devDependencies) [](https://coveralls.io/r/nfroidure/yerror?branch=master) [](https://codeclimate.com/github/nfroidure/yerror) | ||
[//]: # (::contents:start) | ||
## Usage | ||
@@ -12,3 +27,3 @@ | ||
```js | ||
var YError = require('yerror'); | ||
import YError from 'yerror'; | ||
``` | ||
@@ -32,7 +47,6 @@ | ||
// (...) | ||
``` | ||
You don't have to use constant like error messages, we use this convention | ||
mainly for i18n reasons. | ||
mainly for i18n reasons. | ||
@@ -66,32 +80,86 @@ Also, you could want to wrap errors and keep a valuable stack trace: | ||
// (...) | ||
``` | ||
``` | ||
[//]: # (::contents:end) | ||
## API | ||
# API | ||
<a name="YError"></a> | ||
### YError(msg:String, ...args:Mixed):Error | ||
## YError ⇐ <code>Error</code> | ||
An YError class able to contain some params and | ||
print better stack traces | ||
Creates a new YError with `msg` as a message and `args` as debug values. | ||
**Kind**: global class | ||
**Extends**: <code>Error</code> | ||
### YError.wrap(err:Error, msg:String, ...args:Mixed):Error | ||
* [YError](#YError) ⇐ <code>Error</code> | ||
* [new YError([errorCode], [...params])](#new_YError_new) | ||
* [.wrap(err, [errorCode], [...params])](#YError.wrap) ⇒ [<code>YError</code>](#YError) | ||
* [.cast(err, [errorCode], [...params])](#YError.cast) ⇒ [<code>YError</code>](#YError) | ||
* [.bump(err, [errorCode], [...params])](#YError.bump) ⇒ [<code>YError</code>](#YError) | ||
Wraps any error and output a YError with `msg` as its message and `args` as | ||
debug values. | ||
<a name="new_YError_new"></a> | ||
### YError.cast(err:Error, msg:String, ...args:Mixed):Error | ||
### new YError([errorCode], [...params]) | ||
Creates a new YError with an error code | ||
and some params as debug values. | ||
Return YError as is or wraps any other error and output a YError with `msg` as | ||
its message and `args` as debug values. | ||
### YError.bump(err:Error, fallbackMsg:String, ...fallbackArgs:Mixed):Error | ||
| Param | Type | Default | Description | | ||
| --- | --- | --- | --- | | ||
| [errorCode] | <code>string</code> | <code>"'E_UNEXPECTED'"</code> | The error code corresponding to the actual error | | ||
| [...params] | <code>any</code> | | Some additional debugging values | | ||
Same than `YError.wrap()` but preserves the message and the debug values of the | ||
YError errors. | ||
<a name="YError.wrap"></a> | ||
## Stats | ||
### YError.wrap(err, [errorCode], [...params]) ⇒ [<code>YError</code>](#YError) | ||
Wraps any error and output a YError with an error | ||
code and some params as debug values. | ||
[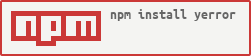](https://nodei.co/npm/yerror/) | ||
[](https://nodei.co/npm/yerror/) | ||
**Kind**: static method of [<code>YError</code>](#YError) | ||
**Returns**: [<code>YError</code>](#YError) - The wrapped error | ||
| Param | Type | Default | Description | | ||
| --- | --- | --- | --- | | ||
| err | <code>Error</code> | | The error to wrap | | ||
| [errorCode] | <code>string</code> | <code>"'E_UNEXPECTED'"</code> | The error code corresponding to the actual error | | ||
| [...params] | <code>any</code> | | Some additional debugging values | | ||
<a name="YError.cast"></a> | ||
### YError.cast(err, [errorCode], [...params]) ⇒ [<code>YError</code>](#YError) | ||
Return a YError as is or wraps any other error and output | ||
a YError with a code and some params as debug values. | ||
**Kind**: static method of [<code>YError</code>](#YError) | ||
**Returns**: [<code>YError</code>](#YError) - The wrapped error | ||
| Param | Type | Default | Description | | ||
| --- | --- | --- | --- | | ||
| err | <code>Error</code> | | The error to cast | | ||
| [errorCode] | <code>string</code> | <code>"'E_UNEXPECTED'"</code> | The error code corresponding to the actual error | | ||
| [...params] | <code>any</code> | | Some additional debugging values | | ||
<a name="YError.bump"></a> | ||
### YError.bump(err, [errorCode], [...params]) ⇒ [<code>YError</code>](#YError) | ||
Same than `YError.wrap()` but preserves the code | ||
and the debug values of the error if it is | ||
already an instance of the YError constructor. | ||
**Kind**: static method of [<code>YError</code>](#YError) | ||
**Returns**: [<code>YError</code>](#YError) - The wrapped error | ||
| Param | Type | Default | Description | | ||
| --- | --- | --- | --- | | ||
| err | <code>Error</code> | | The error to bump | | ||
| [errorCode] | <code>string</code> | <code>"'E_UNEXPECTED'"</code> | The error code corresponding to the actual error | | ||
| [...params] | <code>any</code> | | Some additional debugging values | | ||
# Authors | ||
- [Nicolas Froidure (formerly at SimpliField)](http://insertafter.com/en/index.html) | ||
# License | ||
[MIT](https://github.com/nfroidure/yerror/blob/master/LICENSE) |
@@ -1,5 +0,17 @@ | ||
const os = require('os'); | ||
import os from 'os'; | ||
// Create an Error able to contain some params and better stack traces | ||
/** | ||
* An YError class able to contain some params and | ||
* print better stack traces | ||
* @extends Error | ||
*/ | ||
class YError extends Error { | ||
/** | ||
* Creates a new YError with an error code | ||
* and some params as debug values. | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
*/ | ||
constructor(wrappedErrors, errorCode, ...params) { | ||
@@ -45,3 +57,14 @@ // Detecting if wrappedErrors are passed | ||
// Wrap a classic error | ||
/** | ||
* Wraps any error and output a YError with an error | ||
* code and some params as debug values. | ||
* @param {Error} err | ||
* The error to wrap | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.wrap = function yerrorWrap(err, errorCode, ...params) { | ||
@@ -66,2 +89,14 @@ let yError = null; | ||
/** | ||
* Return a YError as is or wraps any other error and output | ||
* a YError with a code and some params as debug values. | ||
* @param {Error} err | ||
* The error to cast | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.cast = function yerrorCast(err, ...params) { | ||
@@ -74,2 +109,15 @@ if (_looksLikeAYError(err)) { | ||
/** | ||
* Same than `YError.wrap()` but preserves the code | ||
* and the debug values of the error if it is | ||
* already an instance of the YError constructor. | ||
* @param {Error} err | ||
* The error to bump | ||
* @param {string} [errorCode = 'E_UNEXPECTED'] | ||
* The error code corresponding to the actual error | ||
* @param {...any} [params] | ||
* Some additional debugging values | ||
* @return {YError} | ||
* The wrapped error | ||
*/ | ||
YError.bump = function yerrorBump(err, ...params) { | ||
@@ -101,2 +149,2 @@ if (_looksLikeAYError(err)) { | ||
module.exports = YError; | ||
export default YError; |
/* eslint max-nested-callbacks:[0], no-magic-numbers:[0] */ | ||
var assert = require('assert'); | ||
var YError = require('./index.js'); | ||
import assert from 'assert'; | ||
import YError from '.'; | ||
@@ -5,0 +5,0 @@ describe('YError', () => { |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Shell access
Supply chain riskThis module accesses the system shell. Accessing the system shell increases the risk of executing arbitrary code.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 3 instances in 1 package
858
163
0
98426
28
15