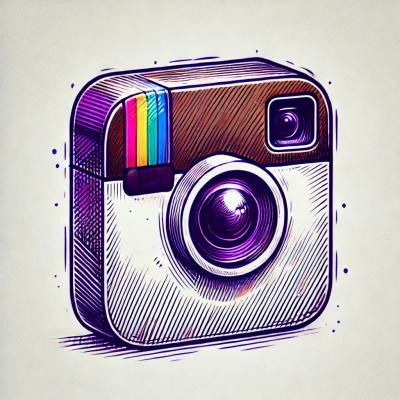
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
:Author: The Blosc development team :Contact: blosc@blosc.org :Github: https://github.com/Blosc/python-blosc2 :Actions: |actions| :PyPi: |version| :NumFOCUS: |numfocus| :Code of Conduct: |Contributor Covenant|
.. |version| image:: https://img.shields.io/pypi/v/blosc2.svg :target: https://pypi.python.org/pypi/blosc2 .. |Contributor Covenant| image:: https://img.shields.io/badge/Contributor%20Covenant-v2.0%20adopted-ff69b4.svg :target: https://github.com/Blosc/community/blob/master/code_of_conduct.md .. |numfocus| image:: https://img.shields.io/badge/powered%20by-NumFOCUS-orange.svg?style=flat&colorA=E1523D&colorB=007D8A :target: https://numfocus.org .. |actions| image:: https://github.com/Blosc/python-blosc2/actions/workflows/build.yml/badge.svg :target: https://github.com/Blosc/python-blosc2/actions/workflows/build.yml
Python-Blosc2 is a high-performance compressed ndarray library with a flexible
compute engine, using C-Blosc2 <https://www.blosc.org/c-blosc2/c-blosc2.html>
_
as its compression backend. It allows complex calculations on compressed data,
whether stored in memory, on disk, or over the network (e.g., via
Caterva2 <https://github.com/ironArray/Caterva2>
). It uses the
C-Blosc2 simple and open format <https://github.com/Blosc/c-blosc2/blob/main/README_FORMAT.rst>
for storing
compressed data.
More info: https://www.blosc.org/python-blosc2/getting_started/overview.html
Binary packages are available for major OSes (Win, Mac, Linux) and platforms.
Install from PyPi using pip
:
.. code-block:: console
pip install blosc2 --upgrade
Conda users can install from conda-forge:
.. code-block:: console
conda install -c conda-forge python-blosc2
The documentation is available here:
https://blosc.org/python-blosc2/python-blosc2.html
You can find examples at:
https://github.com/Blosc/python-blosc2/tree/main/examples
A tutorial from PyData Global 2024 is available at:
https://github.com/Blosc/Python-Blosc2-3.0-tutorial
It contains Jupyter notebooks explaining the main features of Python-Blosc2.
This software is licensed under a 3-Clause BSD license. A copy of the
python-blosc2 license can be found in
LICENSE.txt <https://github.com/Blosc/python-blosc2/tree/main/LICENSE.txt>
_.
Discussion about this package is welcome at:
https://github.com/Blosc/python-blosc2/discussions
Stay informed about the latest developments by following us in
Mastodon <https://fosstodon.org/@Blosc2>
,
Bluesky <https://bsky.app/profile/blosc.org>
or
LinkedIn <https://www.linkedin.com/company/88381936/admin/dashboard/>
_.
Blosc2 is supported by the NumFOCUS foundation <https://numfocus.org>
, the
LEAPS-INNOV project <https://www.leaps-innov.eu>
and ironArray SLU <https://ironarray.io>
_, among many other donors.
This allowed the following people have contributed in an important way
to the core development of the Blosc2 library:
In addition, other people have participated to the project in different aspects:
You can cite our work on the various libraries under the Blosc umbrella as follows:
.. code-block:: console
@ONLINE{blosc, author = {{Blosc Development Team}}, title = "{A fast, compressed and persistent data store library}", year = {2009-2025}, note = {https://blosc.org} }
If you find Blosc useful and want to support its development, please consider
making a donation via the NumFOCUS <https://numfocus.org/donate-to-blosc>
_
organization, which is a non-profit that supports many open-source projects.
Thank you!
Compress Better, Compute Bigger
FAQs
A fast & compressed ndarray library with a flexible compute engine.
We found that blosc2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.