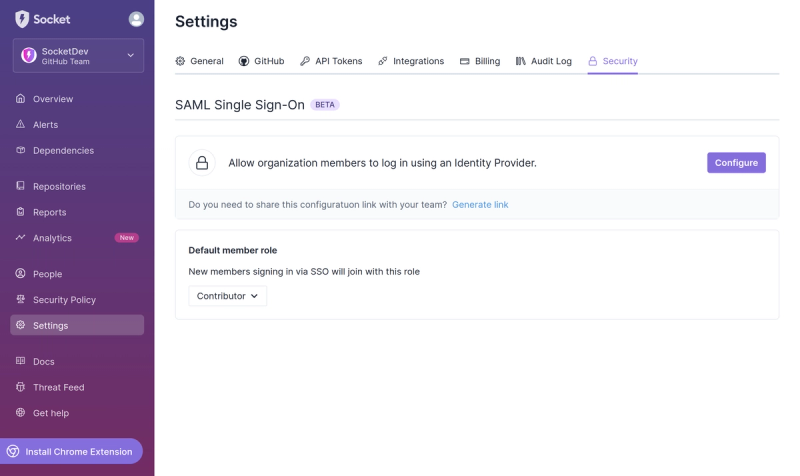
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Readme
Wrapper for the Cuttly URL shortener API.
pip install cuttpy
or python -m pip install cuttpy
Make sure to generate an API key by signing up at cutt.ly and by going to the edit account page, https://cutt.ly/edit.
This README is the documentation :smile:
Basic shortener
from cuttpy import Cuttpy
# define shortener
shortener = Cuttpy("YOUR API KEY")
# shorten URL
response = shortener.shorten("https://www.google.com")
# print shortened url
print(response.shortened_url)
Shortener with basic error handling
from cuttpy import Cuttpy
# define shortener
shortener = Cuttpy("YOUR API KEY")
# shorten URL
response = shortener.shorten("https://www.google.com")
try:
# print shortened url
print(response.shortened_url)
# handle AttributeError because the attribute shortened_url does not return if there was an issue shortening the URL
except AttributeError:
print("An error occurred.")
Shortener with advanced error handling
from cuttpy import Cuttpy
# define shortener
shortener = Cuttpy("YOUR API KEY")
# shorten URL
response = shortener.shorten("https://www.google.com")
# check response code and act accordingly
# you can learn what codes there are later in the README
if response.code == 0:
# a response code of 0 means there was a serverside error.
# it is a good idea to also print the http code in this case.
# print the builtin description for the error
print(f"{response.description}\n{response.http}")
elif not response.code == 7:
# print the builtin description for the error
print(response.description)
elif response.code == 7:
# a response code of 7 means there was no error.
# print shortened url
print(response.shortened_url)
Cuttpy()
The only class worth your time in this library.
Methods:
shorten(url)
- Returns a CuttpyResponse()
object with everything you need.
CuttpyResponse()
The return type of method Cuttpy().shorten()
with various attributes.
Attributes:
Attributes that always return
These attributes always return even if the API fails.
Name | Description |
---|---|
http | The HTTP status code returned by the API. |
code | The code that the wrapper returns. View what they mean below. |
description | A hardcoded description for each wrapper code. View what they mean below. |
Wrapper Codes and descriptions
0 - Unknown serverside error
1 - URL has already been shortened
2 - Entered URL is not a URL
3 - Preferred URL name is already taken
4 - Invalid API key.
5 - URL did not pass the validation. Includes invalid characters
6 - URL provided is from a blocked domain
7 - URL has been shortened successfully
Attributes that return only if the URL was shortened.
Title speaks for itself.
Name | Description |
---|---|
original_url | The original URL which was shortened by the API. |
shortened_url | The shortened version of the original URL. |
This API wrapper uses a system of error codes. View what they mean in the attributes section of the CuttpyResponse()
class.
FAQs
Wrapper for the cutt.ly URL shortener API.
We found that cuttpy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.