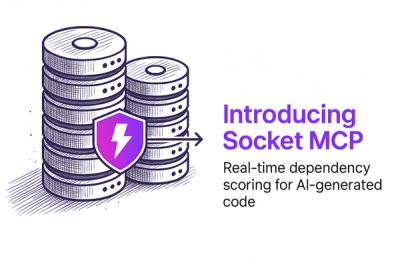
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
CV video player is a Python-based customizable video player that helps computer vision practitioners develop, analyze, and debug their video-related algorithms and models.
The video player is made with simplicity in mind to allow users to easily add or remove functionality. It is only based OpenCV with no additional GUI frameworks, and It's interactivity is based on keyboard presses (no buttons).
The player is designed as a callback system. When initialized the player receives a
list of callbacks, each with a edit_frame
method.
On runtime the callbacks will run in order as specified in the input list. Each callback can also optionally add
keyboard shortcuts to change visualization settings in real time.
pip install cvvideoplayer
from cvvideoplayer import create_video_player
from cvvideoplayer.frame_editors import FrameInfoOverlay, KeyMapOverlay, FitFrameToScreen
VIDEO_OR_FRAME_FOLDER_PATH = "<add local path here>"
def run_player():
video_player = create_video_player(
video_source=VIDEO_OR_FRAME_FOLDER_PATH,
frame_edit_callbacks=[
FitFrameToScreen(),
FrameInfoOverlay(),
KeyMapOverlay(),
],
record=True,
)
video_player.run()
if __name__ == "__main__":
run_player()
In this example, we initiate a very basic video player that will play "example_video.mp4" with added basic frame edit callbacks:
FitFrameToScreen
: Resizes the frame to fit the screen sizeFrameInfoOverlay
: Prints the current frame number and original frame resolution in the top left cornerKeyMapOverlay
: prints all optional shortcuts registered by all callbacksCheck out the ./demos
folder which shows the use of other cool frame edit callback
such as OpticalFlow
and DetectionCsvPlotter
FAQs
moduler multi purpose video player for debugging algorithms in python
We found that cvvideoplayer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.