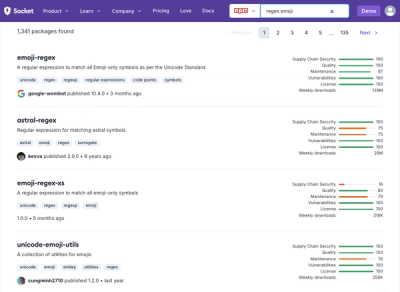
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
A python library that provides a http server mock that can be used for testing code that should interact with an http server
httpservermock
provides a HTTP server mock that can be used to test code that needs to interact with an HTTP server.
pip install httpservermock
# or
poetry add --dev httpservermock
Example usage:
from urllib.error import HTTPError
from urllib.request import urlopen
import pytest
from httpservermock import MethodName, MockHTTPResponse, ServedBaseHTTPServerMock
def test_example() -> None:
with ServedBaseHTTPServerMock() as httpmock:
httpmock.responses[MethodName.GET].append(
MockHTTPResponse(404, "Not Found", b"gone away", {})
)
httpmock.responses[MethodName.GET].append(
MockHTTPResponse(200, "OK", b"here it is", {})
)
# send a request to get the first response
with pytest.raises(HTTPError) as raised:
urlopen(f"{httpmock.url}/bad/path")
assert raised.value.code == 404
# get and validate request that the mock received
req = httpmock.requests[MethodName.GET].pop(0)
assert req.path == "/bad/path"
# send a request to get the second response
resp = urlopen(f"{httpmock.url}/")
assert resp.status == 200
assert resp.read() == b"here it is"
httpmock.responses[MethodName.GET].append(
MockHTTPResponse(404, "Not Found", b"gone away", {})
)
httpmock.responses[MethodName.GET].append(
MockHTTPResponse(200, "OK", b"here it is", {})
)
FAQs
A python library that provides a http server mock that can be used for testing code that should interact with an http server
We found that httpservermock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.