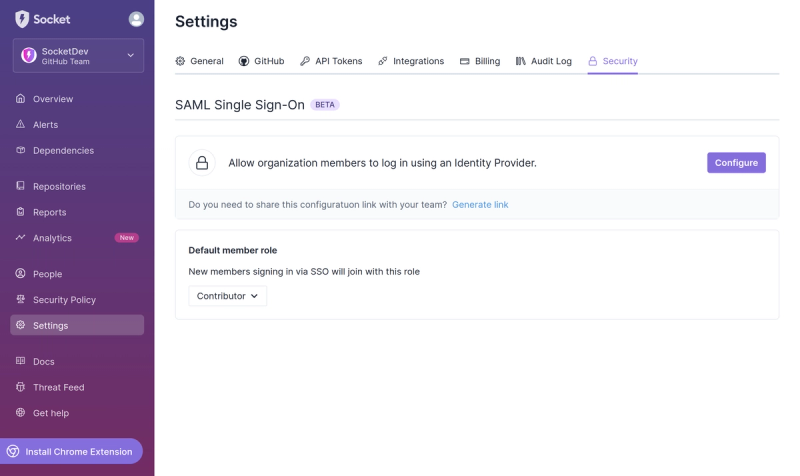
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Readme
Jsoup is a python library that helps to parse and build HTML/XML structures using JSON format.
Use the package manager pip to install jsoup.
pip install jsoup
from jsoup import JsonTreeBuilder
from bs4 import BeautifulSoup
json = {
"body": {
"h1": {"attrs":{"class":"heading1"}, "text":"Hello World"},
"p": ["this ", "is ", "a ","test 1<2 && 2>1", {"comment":["this is a comment"]}],
"comment": "this is also a comment",
"br": None,
"form" : {
"attrs": {
"method": "post"
},
"input": {"attrs":{
"type": "text",
"name": "username"
}}
}
}
}
soup = BeautifulSoup(json, builder=JsonTreeBuilder)
print(soup.prettify())
<body>
<h1 class="heading1">
Hello World
</h1>
<p>
this
</p>
<p>
is
</p>
<p>
a
</p>
<p>
test 1<2 && 2>1
</p>
<p>
<!--this is a comment-->
</p>
<!--this is also a comment-->
<br/>
<form method="post">
<input name="username" type="text"/>
</form>
</body>
We welcome contributions to jsoup
. To get started, follow these steps:
python -m unittest discover -v
.We appreciate all contributions and thank all the contributors!
FAQs
Convert JSON to BeautifulSoup object
We found that jsoup demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.