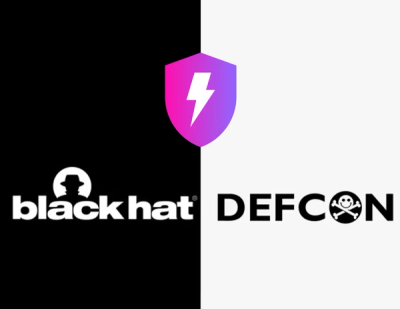
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Take full control of your keyboard with this small Python library. Hook global events, register hotkeys, simulate key presses and much more.
ctrl+shift+m, ctrl+space
) with controllable timeout.Ctrl+ç
).pip install mouse
).Install the PyPI package:
pip install keyboard
or clone the repository (no installation required, source files are sufficient):
git clone https://github.com/boppreh/keyboard
or download and extract the zip into your project folder.
Then check the API docs below to see what features are available.
Use as library:
import keyboard
keyboard.press_and_release('shift+s, space')
keyboard.write('The quick brown fox jumps over the lazy dog.')
keyboard.add_hotkey('ctrl+shift+a', print, args=('triggered', 'hotkey'))
# Press PAGE UP then PAGE DOWN to type "foobar".
keyboard.add_hotkey('page up, page down', lambda: keyboard.write('foobar'))
# Blocks until you press esc.
keyboard.wait('esc')
# Record events until 'esc' is pressed.
recorded = keyboard.record(until='esc')
# Then replay back at three times the speed.
keyboard.play(recorded, speed_factor=3)
# Type @@ then press space to replace with abbreviation.
keyboard.add_abbreviation('@@', 'my.long.email@example.com')
# Block forever, like `while True`.
keyboard.wait()
Use as standalone module:
# Save JSON events to a file until interrupted:
python -m keyboard > events.txt
cat events.txt
# {"event_type": "down", "scan_code": 25, "name": "p", "time": 1622447562.2994788, "is_keypad": false}
# {"event_type": "up", "scan_code": 25, "name": "p", "time": 1622447562.431007, "is_keypad": false}
# ...
# Replay events
python -m keyboard < events.txt
event.device == None
). #21/dev/input/input*
) but this requires root.keyboard
will be unable to report events.keyboard
via SSH, the server will not detect your key events.import keyboard
keyboard.add_hotkey('space', lambda: print('space was pressed!'))
# If the program finishes, the hotkey is not in effect anymore.
# Don't do this! This will use 100% of your CPU.
#while True: pass
# Use this instead
keyboard.wait()
# or this
import time
while True:
time.sleep(1000000)
import keyboard
# Don't do this! This will use 100% of your CPU until you press the key.
#
#while not keyboard.is_pressed('space'):
# continue
#print('space was pressed, continuing...')
# Do this instead
keyboard.wait('space')
print('space was pressed, continuing...')
import keyboard
# Don't do this!
#
#while True:
# if keyboard.is_pressed('space'):
# print('space was pressed!')
#
# This will use 100% of your CPU and print the message many times.
# Do this instead
while True:
keyboard.wait('space')
print('space was pressed! Waiting on it again...')
# or this
keyboard.add_hotkey('space', lambda: print('space was pressed!'))
keyboard.wait()
import keyboard
# Don't do this! This will call `print('space')` immediately then fail when the key is actually pressed.
#keyboard.add_hotkey('space', print('space was pressed'))
# Do this instead
keyboard.add_hotkey('space', lambda: print('space was pressed'))
# or this
def on_space():
print('space was pressed')
keyboard.add_hotkey('space', on_space)
# or this
while True:
# Wait for the next event.
event = keyboard.read_event()
if event.event_type == keyboard.KEY_DOWN and event.name == 'space':
print('space was pressed')
# Don't do this! The `keyboard` module is meant for global events, even when your program is not in focus.
#import keyboard
#print('Press any key to continue...')
#keyboard.get_event()
# Do this instead
input('Press enter to continue...')
# Or one of the suggestions from here
# https://stackoverflow.com/questions/983354/how-to-make-a-script-wait-for-a-pressed-key
FAQs
Hook and simulate keyboard events on Windows and Linux
We found that keyboarded demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.