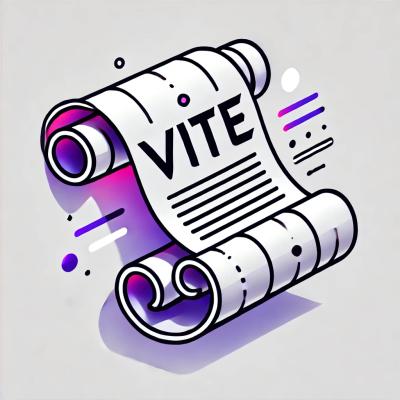
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
You can install mangodm
via pip
.
pip install mangodm
Firstly need to connect to MongoDB.
from mangodm import connect_to_mongo
MONGODB_CONNECTION_URL = ""
DATABASE_NAME = ""
async def main():
await connect_to_mongo(MONGODB_CONNECTION_URL, DATABASE_NAME)
Don't forgot to close connection.
from mangodm import connect_to_mongo, close_connection
MONGODB_CONNECTION_URL = ""
DATABASE_NAME = ""
def befor_down():
close_connection()
async def main():
await connect_to_mongo(MONGODB_CONNECTION_URL, DATABASE_NAME)
Inherit Document
class and describe its fields to defining new collection.
from mangodm import Document
class User(Document):
name: str
age: int
Before start to use collection you need to register it.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection() # IMPORTANT
Also you can add special configuration to collection. To do this add subclass Config
.
Config Parameters
from mangodm import Document
class User(Document):
name: str
password: str
age: int
points: int
class Config:
collection_name = "users"
excludeFields = ["points"]
excludeFieldsResponse: ["password"]
User.register_collection()
For create new document just create new object of collection class and call create()
method from it.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
mike = User(name="Mike", age=23)
await mike.create()
After creating you can get document id
. ID is str
type.
async def main():
mike = User(name="Mike", age=23)
await mike.create()
print(mike.id)
To get one document use method - get
. Parameters for this method is filter for finding document. Parameter convert to JSON and put to MongoDB method find_one
.
Method return class object or none
if querying document doesn't exist.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
mike = await User.get(name="Mike")
if mike:
print(mike.id)
anna = await User.get(id="6561a3851492b7e9d9a9533e")
if anna:
print(anna.age)
To get all documets which suitable for finding filter use method - find
. This method has same paramrtrs how method get
. find
method return list of class objects, list can be empty.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
users = await User.find() # get all documents if no parametrs
for user in users:
print(user.id)
To update editing use method update
.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
mike = await User.get(name="Mike")
if mike:
print(mike.id)
mike.age = 24
await mike.update()
To delete document use method delete
.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
mike = await User.get(name="Mike")
if mike:
await mike.delete()
You can convert your document to JSON. For this use method to_response()
.
from mangodm import Document
class User(Document):
name: str
age: int
User.register_collection()
async def main():
mike = await User.get(name="Mike")
if mike:
print(mike.to_response())
FAQs
mangODM - simple async ODM for mongodb.
We found that mangodm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.