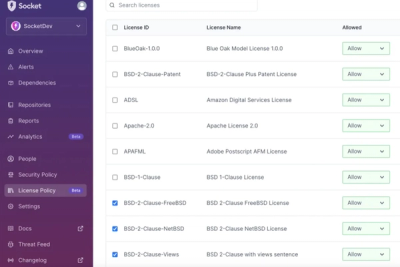
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
This is an extremely simple command-line utility for obfuscating and deobfuscating data.
--binary
override is available.The program is a single file, so you can just download/run it manually: python mystipy.py
For the sake of convenience (and to be added to your $PATH
), it is also available on PyPI.
pip install --upgrade mystipy
Obfuscate a file:
mystipy input_file output_file
Deobfuscate a file:
mystipy input_file output_file --reverse
-p, --prompt
: Prompt mode. If provided, you will be prompted for any arguments you don't explicitly set.-r, --reverse
: Deobfuscate the input file.-k, --key
: XOR key for encryption/deencryption. Must be a valid byte string. (Default: "mystipy")-s, --salt
: Number of bytes to use in salt. (Default: 16)--binary
: Disable exadecimal encoding/decoding. The data will be (or is) compressed binary data.Obfuscate a file with a custom key and default settings:
mystipy myfile.txt obfuscated.myst -k "hunter2"
Deobfuscate a binary encoded file:
mystipy obfuscated.myst deobfuscated.txt -r --binary
Use prompt mode for interactive input:
mystipy -p
Use it in your own program:
import mystipy
# Define your key and data
key = "hunter2".encode()
data = b"I would tell you a joke about UDP, but I'm not sure if you'd get it."
# Obfuscate the data
obfuscated_data = mystipy.obfuscate(data, key)
print(f"Obfuscated data: {obfuscated_data}")
# Deobfuscate the data
deobfuscated_data = mystipy.deobfuscate(obfuscated_data, key)
print(f"Deobfuscated data: {deobfuscated_data.decode()}")
The script includes function aliases to remove ambiguity if you're using it in your own code:
mystipy
for obfuscate
demystipy
for deobfuscate
import mystipy
print(mystipy.mystipy == mystipy.obfuscate) # True
print(mystipy.demystipy == mystipy.deobfuscate) # True
So if you'd prefer to use the aliases, you'd import the obfuscation/deobfuscation functions like this:
from mystipy import mystipy, demystipy
FAQs
A simple Python data obfuscation utility.
We found that mystipy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.