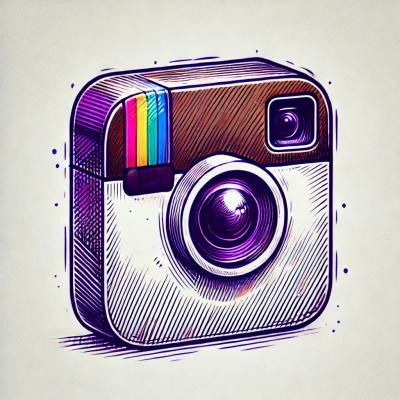
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
A Python library for interacting with OpenAlgo's trading APIs with high-performance technical indicators
A Python library for algorithmic trading using OpenAlgo's REST APIs. This library provides a comprehensive interface for order management, market data, account operations, and strategy automation.
pip install openalgo
from openalgo import api
# Initialize the client
client = api(
api_key="your_api_key",
host="http://127.0.0.1:5000" # or your OpenAlgo server URL
)
OpenAlgo's Strategy Management Module allows you to automate your trading strategies using webhooks. This enables seamless integration with any platform or custom system that can send HTTP requests. The Strategy class provides a simple interface to send signals that trigger orders based on your strategy configuration in OpenAlgo.
from openalgo import Strategy
import requests
# Initialize strategy client
client = Strategy(
host_url="http://127.0.0.1:5000", # Your OpenAlgo server URL
webhook_id="your-webhook-id" # Get this from OpenAlgo strategy section
)
try:
# Long entry (BOTH mode with position size)
response = client.strategyorder("RELIANCE", "BUY", 1)
print(f"Long entry successful: {response}")
# Short entry
response = client.strategyorder("ZOMATO", "SELL", 1)
print(f"Short entry successful: {response}")
# Close positions
response = client.strategyorder("RELIANCE", "SELL", 0) # Close long
response = client.strategyorder("ZOMATO", "BUY", 0) # Close short
except requests.exceptions.RequestException as e:
print(f"Error sending order: {e}")
Strategy Modes:
The Strategy Management Module can be integrated with:
Get funds and margin details of the trading account.
result = client.funds()
# Returns:
{
"data": {
"availablecash": "18083.01",
"collateral": "0.00",
"m2mrealized": "0.00",
"m2munrealized": "0.00",
"utiliseddebits": "0.00"
},
"status": "success"
}
Get orderbook details with statistics.
result = client.orderbook()
# Returns order details and statistics including:
# - Total buy/sell orders
# - Total completed/open/rejected orders
# - Individual order details with status
Get execution details of trades.
result = client.tradebook()
# Returns list of executed trades with:
# - Symbol, action, quantity
# - Average price, trade value
# - Timestamp, order ID
Get current positions across all segments.
result = client.positionbook()
# Returns list of positions with:
# - Symbol, exchange, product
# - Quantity, average price
Get stock holdings with P&L details.
result = client.holdings()
# Returns:
# - List of holdings with quantity and P&L
# - Statistics including total holding value
# - Total investment value and P&L
Place a regular order.
result = client.placeorder(
symbol="RELIANCE",
exchange="NSE",
action="BUY",
quantity=1,
price_type="MARKET",
product="MIS"
)
Place an order with position sizing.
result = client.placesmartorder(
symbol="RELIANCE",
exchange="NSE",
action="BUY",
quantity=1,
position_size=100,
price_type="MARKET",
product="MIS"
)
Place multiple orders simultaneously.
orders = [
{
"symbol": "RELIANCE",
"exchange": "NSE",
"action": "BUY",
"quantity": 1,
"pricetype": "MARKET",
"product": "MIS"
},
{
"symbol": "INFY",
"exchange": "NSE",
"action": "SELL",
"quantity": 1,
"pricetype": "MARKET",
"product": "MIS"
}
]
result = client.basketorder(orders=orders)
Split a large order into smaller ones.
result = client.splitorder(
symbol="YESBANK",
exchange="NSE",
action="SELL",
quantity=105,
splitsize=20,
price_type="MARKET",
product="MIS"
)
Check status of a specific order.
result = client.orderstatus(
order_id="24120900146469",
strategy="Test Strategy"
)
Get current open position for a symbol.
result = client.openposition(
symbol="YESBANK",
exchange="NSE",
product="CNC"
)
Modify an existing order.
result = client.modifyorder(
order_id="24120900146469",
symbol="RELIANCE",
action="BUY",
exchange="NSE",
quantity=2,
price="2100",
product="MIS",
price_type="LIMIT"
)
Cancel a specific order.
result = client.cancelorder(
order_id="24120900146469"
)
Cancel all open orders.
result = client.cancelallorder()
Close all open positions.
result = client.closeposition()
The WebSocket Feed API provides real-time market data through WebSocket connections. The API supports three types of market data:
Get real-time LTP updates for multiple instruments:
from openalgo import api
import time
# Initialize the client with explicit WebSocket URL
client = api(
api_key="your_api_key",
host="http://127.0.0.1:5000", # REST API host
ws_url="ws://127.0.0.1:8765" # WebSocket server URL (can be different from REST API)
)
# Define instruments to subscribe to
instruments = [
{"exchange": "MCX", "symbol": "GOLDPETAL30MAY25FUT"},
{"exchange": "MCX", "symbol": "GOLD05JUN25FUT"}
]
# Callback function for data updates
def on_data_received(data):
print("LTP Update:")
print(data)
# Connect and subscribe
client.connect()
client.subscribe_ltp(instruments, on_data_received=on_data_received)
# Poll LTP data
print(client.get_ltp())
# Returns nested format:
# {"ltp": {"MCX": {"GOLDPETAL30MAY25FUT": {"timestamp": 1747761583959, "ltp": 9529.0}}}}
# Cleanup
client.unsubscribe_ltp(instruments)
client.disconnect()
Get real-time quote updates with OHLC data:
from openalgo import api
# Initialize the client
client = api(
api_key="your_api_key",
host="http://127.0.0.1:5000",
ws_url="ws://127.0.0.1:8765"
)
# Define instruments
instruments = [
{"exchange": "MCX", "symbol": "GOLDPETAL30MAY25FUT"}
]
# Connect and subscribe
client.connect()
client.subscribe_quote(instruments)
# Poll quote data
print(client.get_quotes())
# Returns nested format:
# {"quote": {"MCX": {"GOLDPETAL30MAY25FUT": {
# "timestamp": 1747767126517,
# "open": 9430.0,
# "high": 9544.0,
# "low": 9390.0,
# "close": 9437.0,
# "ltp": 9535.0
# }}}}
# Cleanup
client.unsubscribe_quote(instruments)
client.disconnect()
Get real-time market depth (order book) data:
from openalgo import api
# Initialize the client
client = api(
api_key="your_api_key",
host="http://127.0.0.1:5000",
ws_url="ws://127.0.0.1:8765"
)
# Define instruments
instruments = [
{"exchange": "MCX", "symbol": "GOLDPETAL30MAY25FUT"}
]
# Connect and subscribe
client.connect()
client.subscribe_depth(instruments)
# Poll depth data
print(client.get_depth())
# Returns nested format with order book:
# {"depth": {"MCX": {"GOLDPETAL30MAY25FUT": {
# "timestamp": 1747767126517,
# "ltp": 9535.0,
# "buyBook": {"1": {"price": "9533.0", "qty": "53332", "orders": "0"}, ...},
# "sellBook": {"1": {"price": "9535.0", "qty": "53332", "orders": "0"}, ...}
# }}}}
# Cleanup
client.unsubscribe_depth(instruments)
client.disconnect()
Get real-time quotes for a symbol using REST API.
result = client.quotes(
symbol="RELIANCE",
exchange="NSE"
)
# Returns bid/ask, LTP, volume and other quote data
Get market depth (order book) data.
result = client.depth(
symbol="RELIANCE",
exchange="NSE"
)
# Returns market depth with top 5 bids/asks
Get historical price data.
result = client.history(
symbol="RELIANCE",
exchange="NSE",
interval="5m", # Use intervals() to get supported intervals
start_date="2024-01-01",
end_date="2024-01-31"
)
# Returns pandas DataFrame with OHLC data
Get supported time intervals for historical data.
result = client.intervals()
# Returns:
{
"status": "success",
"data": {
"seconds": ["1s"],
"minutes": ["1m", "2m", "3m", "5m", "10m", "15m", "30m", "60m"],
"hours": [],
"days": ["D"],
"weeks": [],
"months": []
}
}
Note: The legacy
interval()
method is still available but will be deprecated in future versions.
Get details for a specific trading symbol.
result = client.symbol(
symbol="NIFTY24APR25FUT",
exchange="NFO"
)
# Returns:
{
"status": "success",
"data": {
"brexchange": "NFO",
"brsymbol": "NIFTY24APR25FUT",
"exchange": "NFO",
"expiry": "24-APR-25",
"id": 39521,
"instrumenttype": "FUTIDX",
"lotsize": 75,
"name": "NIFTY",
"strike": -0.01,
"symbol": "NIFTY24APR25FUT",
"tick_size": 0.05,
"token": "54452"
}
}
Check the examples directory for detailed usage:
Update version in openalgo/__init__.py
Build the distribution:
python -m pip install --upgrade build
python -m build
python -m pip install --upgrade twine
python -m twine upload dist/*
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A Python library for interacting with OpenAlgo's trading APIs with high-performance technical indicators
We found that openalgo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.