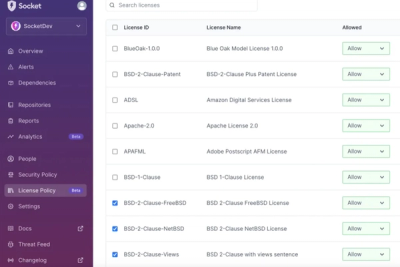
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
The PSystem library allows you to create P Systems and evolve them, enabling complex simulations of these computational models.
To create a PSystem object:
ps = PSystem(H, V, base_struct, m_objects, m_plasmids, m_rules, p_rules, i0)
Parameter | Type | Description | Default |
---|---|---|---|
H | dict | Plasmids' alphabet and its rules. | None |
V | list | System's alphabet. | [ ] |
base_struct | str | Initial system's structure. | "[1]1" |
m_objects | dict | Membrane's objects. | { 0 : '' } |
m_plasmids | dict | Membranes' plasmids. | None |
m_rules | dict | Membrane's rules. | { 0 : { } } |
p_rules | dict | Rules priority in each membrane. | { 0: [ ] } |
i0 | int | Output membrane. | 1 |
ps.steps(n, verbose=False): Evolve the system n steps. If verbose is True, prints the system's structure at each step. Returns the system dictionary after applying n steps.
ps.while_evolve(verbose=False): Evolve the system until all possible iterations are finished. If verbose is True, prints the system's structure at each step. Returns the system dictionary after applying all iterations.
ps.evolve(feasible_rules, verbose=False): Evolve the system by choosing a random membrane from feasible_rules list whose items are a tuple of membrane's id and their rules to apply. If verbose is True, prints the membrane where the rules are being applied, the rules applied, and the number of times each rule has been applied.
ps.get_feasible_rules(): Get feasible rules from all the membranes in the current state.
ps.get_memb_feasible_rules(memb_id): Get a combination of rules that can be applied all at once in the membrane with id memb_id. ps.accessible_plasmids(memb_id): Get the plasmids that could go into the membrane with id memb_id.
ps.print_system(): Print the system's structure.
ps.to_dict(): Returns the system structure in a dictionary.
memb = Membrane(V, id, parent, objects, plasmids, rules, p_rules)
Parameter | Type | Description | Default |
---|---|---|---|
V | list | Membrane's alphabet (same as system's) | |
id | int | Membrane's id | |
parent | int | Parent Membrane's id. | None |
objects | str | Membrane's objects. | '' |
plasmids | list | Membrane's plasmids. | [ ] |
rules | dict | Membrane's rules. | {} |
p_rules | dict | Rules priority in membrane. | [ ] |
A P System generating n², n >= 1
from p_system_simulate import *
alphabet = ['a','b','x','c','f']
struct = '[1[2[3]3[4]4]2]1'
m_objects = {
3:'af',
}
r_2 = {
1:('x','b'),
2:('b','bc4'),
3:('ff','f'),
4:('f','a.')
}
r_3 = {
1:('a','ax'),
2:('a','x.'),
3:('f','ff')
}
m_rules = {
2:r_2,
3:r_3,
}
p_rules = {
2:[(3,4)],
}
i0 = 4
ps = PSystem(V=alphabet, base_struct=struct, m_objects=m_objects, m_rules=m_rules, p_rules=p_rules, i0=i0)
print(ps.while_evolve(verbose=True))
[1 '' [2 '' [3 'fa' ]3[4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 3 | n_times: 1 -> rule '1': ('a', 'ax')
membrane: 3 | n_times: 1 -> rule '3': ('f', 'ff')
[1 '' [2 '' [3 'ffxa' ]3[4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 3 | n_times: 1 -> rule '1': ('a', 'ax')
membrane: 3 | n_times: 2 -> rule '3': ('f', 'ff')
[1 '' [2 '' [3 'ffffxxa' ]3[4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 3 | n_times: 1 -> rule '1': ('a', 'ax')
membrane: 3 | n_times: 4 -> rule '3': ('f', 'ff')
[1 '' [2 '' [3 'ffffffffxxxa' ]3[4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 3 | n_times: 1 -> rule '2': ('a', 'x.')
membrane: 3 | n_times: 8 -> rule '3': ('f', 'ff')
[1 '' [2 'ffffffffffffffffxxxx' [4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '1': ('x', 'b')
membrane: 2 | n_times: 8 -> rule '3': ('ff', 'f')
[1 '' [2 'ffffffffbbbb' [4 '' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '2': ('b', 'bc4')
membrane: 2 | n_times: 4 -> rule '3': ('ff', 'f')
[1 '' [2 'ffffbbbb' [4 'cccc' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '2': ('b', 'bc4')
membrane: 2 | n_times: 2 -> rule '3': ('ff', 'f')
[1 '' [2 'ffbbbb' [4 'cccccccc' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '2': ('b', 'bc4')
membrane: 2 | n_times: 1 -> rule '3': ('ff', 'f')
[1 '' [2 'fbbbb' [4 'cccccccccccc' ]4]2]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '2': ('b', 'bc4')
membrane: 2 | n_times: 1 -> rule '4': ('f', 'a.')
[1 'abbbb' [4 'cccccccccccccccc' ]4]1
============================================================================================
{'environment': {'childs': {1: {'childs': {4: {'objects': {'c': 16}}},
'objects': {'a': 1, 'b': 4}}},
'objects': {}}}
A P system that checks if a number n is divisible by another number k.
In this case k = 3 divides n = 15 .
from p_system_simulate import *
n = 15
k = 3
alphabet = ['a','c','x','d']
struct = '[1[2]2[3]3]1'
m_objects = {
2:'a'*n+'c'*k+'d',
3:'a'
}
r_1 = {
1:('dcx','a3')
}
r_2 = {
1:('ac','x'),
2:('ax','c'),
3:('d','d.')
}
m_rules = {
1:r_1,
2:r_2,
}
p_rules = {
2 : [(1,3),(2,3)],
}
i0 = 3
ps = PSystem(V=alphabet, base_struct=struct, m_objects=m_objects, m_rules=m_rules, p_rules=p_rules, i0=i0)
print(ps.while_evolve(verbose=True))
[1 '' [2 'cccdaaaaaaaaaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '1': ('ac', 'x')
[1 '' [2 'xxxdaaaaaaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '2': ('ax', 'c')
[1 '' [2 'cccdaaaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '1': ('ac', 'x')
[1 '' [2 'xxxdaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '2': ('ax', 'c')
[1 '' [2 'cccdaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '1': ('ac', 'x')
[1 '' [2 'xxxd' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 1 -> rule '3': ('d', 'd.')
[1 'xxxd' [3 'a' ]3]1
============================================================================================
{'environment': {'childs': {1: {'childs': {3: {'objects': {'a': 1}}},
'objects': {'d': 1, 'x': 3}}},
'objects': {}}}
In this other case k = 4 not divides n = 15.
[1 '' [2 'ccccdaaaaaaaaaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '1': ('ac', 'x')
[1 '' [2 'xxxxdaaaaaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '2': ('ax', 'c')
[1 '' [2 'ccccdaaaaaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule '1': ('ac', 'x')
[1 '' [2 'xxxxdaaa' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 3 -> rule '2': ('ax', 'c')
[1 '' [2 'cccxd' ]2[3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 1 -> rule '3': ('d', 'd.')
[1 'cccxd' [3 'a' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('dcx', 'a3')
[1 'cc' [3 'aa' ]3]1
============================================================================================
{'environment': {'childs': {1: {'childs': {3: {'objects': {'a': 2}}},
'objects': {'c': 2}}},
'objects': {}}}
A P System that makes an arithmetic substraction operation between m and n.
from p_system_simulate import *
n = 4
m = 10
alphabet = ['a','b','c','p','q']
plasmids = {
"P_1":{"P_1_1":('a','a0')},
"P_2":{"P_2_1":('ab','c0')}
}
struct = '[1[2]2[3]3]1'
m_objects = {
1:'pq',
2:'a'*n,
3:'b'*m
}
m_plasmids = {
0: set(['P_1','P_2'])
}
r_0 = {
1:("P_1[p]1","p[P_1]1"),
2:("P_2[q]1","q[P_2]1"),
}
r_1 = {
1:("P_1[]2","[P_1]2"),
2:("P_2[]3","[P_2]3"),
3:("a","a3"),
}
m_rules = {
0:r_0,
1:r_1,
}
i0 = 3
ps = PSystem(H=plasmids, V=alphabet, base_struct=struct, m_objects=m_objects, m_plasmids=m_plasmids, m_rules=m_rules, i0=i0)
print(ps.while_evolve(verbose=True))
'P_1P_2' '' [1 '' 'pq' [2 '' 'aaaa' ]2 [3 '' 'bbbbbbbbbb' ]3]1
--------------------------------------------------------------------------------------------
enviroment | n_times: 1 -> rule '1': ('P_1[p]1', 'p[P_1]1')
enviroment | n_times: 1 -> rule '2': ('P_2[q]1', 'q[P_2]1')
'' 'pq' [1 'P_1P_2' '' [2 '' 'aaaa' ]2 [3 '' 'bbbbbbbbbb' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('P_1[]2', '[P_1]2')
membrane: 1 | n_times: 1 -> rule '2': ('P_2[]3', '[P_2]3')
'' 'pq' [1 '' '' [2 'P_1' 'aaaa' ]2 [3 'P_2' 'bbbbbbbbbb' ]3]1
--------------------------------------------------------------------------------------------
membrane: 2 | n_times: 4 -> rule 'P_1_1': ('a', 'a0')
'' 'pq' [1 '' 'aaaa' [2 'P_1' '' ]2 [3 'P_2' 'bbbbbbbbbb' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 4 -> rule '3': ('a', 'a3')
'' 'pq' [1 '' '' [2 'P_1' '' ]2 [3 'P_2' 'aaaabbbbbbbbbb' ]3]1
--------------------------------------------------------------------------------------------
membrane: 3 | n_times: 4 -> rule 'P_2_1': ('ab', 'c0')
'' 'pq' [1 '' 'cccc' [2 'P_1' '' ]2 [3 'P_2' 'bbbbbb' ]3]1
============================================================================================
{'environment': {'childs': {1: {'childs': {2: {'objects': {},
'plasmids': {'P_1'}},
3: {'objects': {'b': 6},
'plasmids': {'P_2'}}},
'objects': {'c': 4}}},
'objects': {'p': 1, 'q': 1}}}
A P System that makes product operation between m and n.
from p_system_simulate import *
n = 4
m = 5
alphabet = ['a','b','p','x','q','r','t','s']
plasmids = {
"P_1":{"P_1_1":('ba','b')},
"P_2":{"P_2_1":('a',"ab0")},
}
struct = '[1[2]2[3]3]1'
m_objects = {
1:'p',
2:'b' + 'a'*n,
3:'b' + 'a'*m,
}
m_plasmids = {
0: set(['P_1','P_2']),
}
r_0 = {
1:("P_1[p]1","[P_1,x]1"),
2:("P_2[x]1","[P_2,q]1"),
}
r_1 = {
1:("P_1,q[a]2","r[P_1,a]2"),
2:("r[P_1]2","P_1,s[]2"),
3:("P_2,s[]3","t[P_2]3"),
4:("t[P_2]3","P_2,q[]3"),
}
m_rules = {
0:r_0,
1:r_1,
}
i0 = 1
ps = PSystem(H=plasmids, V=alphabet, base_struct=struct, m_objects=m_objects, m_plasmids=m_plasmids, m_rules=m_rules, i0=i0)
print(ps.while_evolve(verbose=True))
'P_1P_2' '' [1 '' 'p' [2 '' 'aaaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
enviroment | n_times: 1 -> rule '1': ('P_1[p]1', '[P_1,x]1')
'P_2' '' [1 'P_1' 'x' [2 '' 'aaaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
enviroment | n_times: 1 -> rule '2': ('P_2[x]1', '[P_2,q]1')
'' '' [1 'P_1P_2' 'q' [2 '' 'aaaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('P_1,q[a]2', 'r[P_1,a]2')
'' '' [1 'P_2' 'r' [2 'P_1' 'aaaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '2': ('r[P_1]2', 'P_1,s[]2')
membrane: 2 | n_times: 1 -> rule 'P_1_1': ('ba', 'b')
'' '' [1 'P_1P_2' 's' [2 '' 'aaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '3': ('P_2,s[]3', 't[P_2]3')
'' '' [1 'P_1' 't' [2 '' 'aaab' ]2 [3 'P_2' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '4': ('t[P_2]3', 'P_2,q[]3')
membrane: 3 | n_times: 5 -> rule 'P_2_1': ('a', 'ab0')
'' '' [1 'P_1P_2' 'bbbbbq' [2 '' 'aaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('P_1,q[a]2', 'r[P_1,a]2')
'' '' [1 'P_2' 'bbbbbr' [2 'P_1' 'aaab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '2': ('r[P_1]2', 'P_1,s[]2')
membrane: 2 | n_times: 1 -> rule 'P_1_1': ('ba', 'b')
'' '' [1 'P_1P_2' 'bbbbbs' [2 '' 'aab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '3': ('P_2,s[]3', 't[P_2]3')
'' '' [1 'P_1' 'bbbbbt' [2 '' 'aab' ]2 [3 'P_2' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '4': ('t[P_2]3', 'P_2,q[]3')
membrane: 3 | n_times: 5 -> rule 'P_2_1': ('a', 'ab0')
'' '' [1 'P_1P_2' 'bbbbbbbbbbq' [2 '' 'aab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('P_1,q[a]2', 'r[P_1,a]2')
'' '' [1 'P_2' 'bbbbbbbbbbr' [2 'P_1' 'aab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '2': ('r[P_1]2', 'P_1,s[]2')
membrane: 2 | n_times: 1 -> rule 'P_1_1': ('ba', 'b')
'' '' [1 'P_1P_2' 'bbbbbbbbbbs' [2 '' 'ab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '3': ('P_2,s[]3', 't[P_2]3')
'' '' [1 'P_1' 'bbbbbbbbbbt' [2 '' 'ab' ]2 [3 'P_2' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '4': ('t[P_2]3', 'P_2,q[]3')
membrane: 3 | n_times: 5 -> rule 'P_2_1': ('a', 'ab0')
'' '' [1 'P_1P_2' 'bbbbbbbbbbbbbbbq' [2 '' 'ab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '1': ('P_1,q[a]2', 'r[P_1,a]2')
'' '' [1 'P_2' 'bbbbbbbbbbbbbbbr' [2 'P_1' 'ab' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '2': ('r[P_1]2', 'P_1,s[]2')
membrane: 2 | n_times: 1 -> rule 'P_1_1': ('ba', 'b')
'' '' [1 'P_1P_2' 'bbbbbbbbbbbbbbbs' [2 '' 'b' ]2 [3 '' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '3': ('P_2,s[]3', 't[P_2]3')
'' '' [1 'P_1' 'bbbbbbbbbbbbbbbt' [2 '' 'b' ]2 [3 'P_2' 'aaaaab' ]3]1
--------------------------------------------------------------------------------------------
membrane: 1 | n_times: 1 -> rule '4': ('t[P_2]3', 'P_2,q[]3')
membrane: 3 | n_times: 5 -> rule 'P_2_1': ('a', 'ab0')
'' '' [1 'P_1P_2' 'bbbbbbbbbbbbbbbbbbbbq' [2 '' 'b' ]2 [3 '' 'aaaaab' ]3]1
============================================================================================
{'environment': {'childs': {1: {'childs': {2: {'objects': {'b': 1}},
3: {'objects': {'a': 5, 'b': 1}}},
'objects': {'b': 20, 'q': 1},
'plasmids': {'P_2', 'P_1'}}},
'objects': {}}}
Using as example a P system deciding whether k divides n, which was used as example of use before:
Object | Parameter | In code | In traditional notation |
---|---|---|---|
PSystem All membs | alphabet | ['a','c','x','d'] | { a, c, x, d } |
PSystem | struct | '[1[2]2[3]3]1' | [1 [2 ]2 [3 ]3 ]1 |
memb1 | objects | '' | λ |
memb2 | objects | 'a'*n+'c'*k+'d' | anckd |
memb3 | objects | 'a' | a |
memb1 | rules | { 1: ( 'dcx', 'a3' )} | dcx → (a, in3) |
memb2 | rules | { 1: ( 'ac', 'x' ), 2: ( 'ax', 'c' ), 3: ( 'd', 'd.' ) } | r1: ac → x, r2: ax → c, r3: d → dδ |
memb3 | rules | { } | Ø |
memb1 | p_rules | [ ] | Ø |
memb2 | p_rules | [ ( 1, 3 ), ( 2, 3 ) ] | { r1 > r3, r2 > r3 } |
memb3 | p_rules | [ ] | Ø |
PSystem | m_rules | { 1 : memb1.rules, 2 : memb2.rules, 3 : memb3.rules } | R1, R2, R3 |
PSystem | p_rules | { 1 : memb1.p_rules, 2 : memb2.p_rules, 3 : memb3.p_rules } | ρ1, ρ2, ρ3 |
Description | In code | In traditional notation |
---|---|---|
Add an object to a membrane | Using 2 to enter to memb2 ( 'a', 'ab2' ) | Using in2 to enter to memb2 a → a ( b, in2 ) |
An object will exit the membrane | Using 0 to exit the membrane ( 'a', 'a0' ) | Using out to exit the membrane a → ( a, out ) |
Remove a membrane (dissolve) | Using '.' to dissolve ( 'b', '.' ) | Using δ to dissolve b → δ |
Priority | ||
Description | In code | In traditional notation |
rule1 more priority than rule2 | ( 1, 2 ) | r1 > r2 |
When using plasmids in rules, they must be listed before the objects. For example, ("P_1a", "P_1a0") indicates that if the plasmid 'P_1' and an object 'a' are present in the membrane, the plasmid 'P_1' will be retained, and the object 'a' will be removed. Interactions between plasmids across different membranes are not handled in the same way as objects. However, it is possible to interact with objects in the same way as with plasmids.
This format allows for operations involving plasmids across different membranes and also supports checking across multiple membranes. To achieve this, the structure of the membranes is defined, along with the objects/plasmids within each membrane.
The structure is defined by specifying the elements in the membrane that the rule applies to, followed by opening square brackets to represent a child membrane. Inside the brackets, list the elements of the child membrane, then close the square bracket and indicate the child membrane ID.
For example:
The best way to understand this is through an example, such as the Mathematical Product P System mentioned earlier.
Object | Parameter | In code | In traditional notation |
---|---|---|---|
PSystem All membs | alphabet | ['a','b','p','x','q','r','t','s'] | { a, b, p, x, q, r, t, s } |
PSystem | plasmids & its rules | { "P_1" : { "P_1_1" : ( 'ba', 'b' ) }, "P_2" : { "P_2_1" : ( 'a', "ab0" ) } } | P_1: { ba → b } P_2: { a → a, ( b, out ) } |
PSystem | struct | '[1[2]2[3]3]1' | [1 [2 ]2 [3 ]3 ]1 |
enviroment | plasmids | [ 'P_1', 'P_2' ] | P_1, P_2 |
memb1 | objects | 'p' | p |
memb2 | objects | b + 'a'*n | b an |
memb3 | objects | b + 'a'*m | b am |
enviroment | rules | { 1 : ( "P_1[p]1", "[P_1x]1" ), 2 : ( "P_2[x]1", "[P_2q]1" ) } | P_1 [1 p ]1 → [1 P_1x ]1 P_2 [1 x ]1 → [1 P_2q ]1 |
memb1 | rules | { 1 : ( "P_1q[a]2", "r[P_1a]2" ), 2 : ( "r[P_1]2", "P_1s[]2" ), 3 : ( "P_2s[]3", "t[P_2]3" ), 4 : ( "t[P_2]3", "P_2q[]3" ) } | P_1q [2 a ]2 → r [2 P_1a ]2 r [2 P_1 ]2 → P_1s [2 ]2 P_2s [3 ]3 → t [3 P_2 ]3 t [3P_2 ]3 → P_2q [3 ]3 |
memb2 | rules | { } | Ø |
memb3 | rules | { } | Ø |
PSystem | m_rules | { 0 : enviroment.rules, 1 : memb1.rules, 2 : memb2.rules, 3 : memb3.rules } | R1, R2, R3 |
FAQs
Library to simulate P Systems
We found that p-system-simulate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.