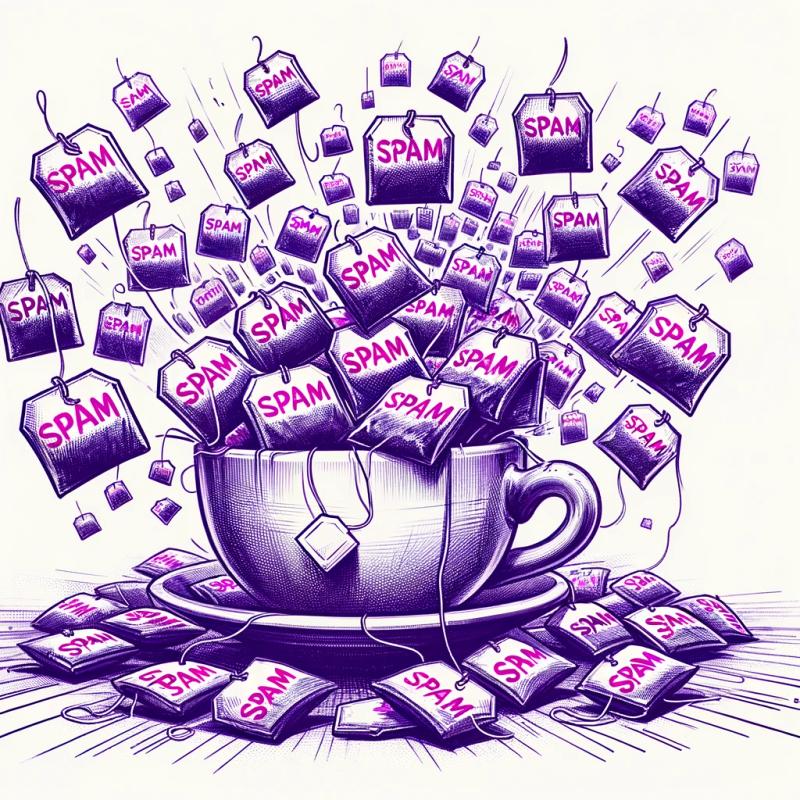
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
A python-based wrapper for the Financial Models Prep pro API (fmpcloud.io) for financial data of public companies
Readme
pyfmpcloud is a Python wrapper library for the Financial Model Prep pro API (fmpcloud.io)
Use the package manager pip to install pyfmpcloud
pip install pyfmpcloud --upgrade --no-cache-dir
Alternatively, download and extract the *.tar.gz folder, and run the following command from the root directory in your shell:
make setup
pyfmpcloud contains a settings
module and five other modules that are the core of the
pyfmpcloud API wrapper.
The following sections show the five main modules for pyfmpcloud and their usage with examples. Note that the modules are structured in the same way as the API documentation that can be found at fmpcloud.io API documentation. The names of the functions within the modules also follow the naming convention on this documentation. This helps with staying consistent. The five modules and their functions are as follows:
from pyfmpcloud import settings
General helper functions to access and update settings for the library environment. These are:
set_apikey
: Please set your API Key in this file using the settings.set_apikey([YOUR KEY]). The key can be retrieved from fmpcloud.io by signing up. Default is the 'demo' key. Example:
settings.set_apikey('stringcontainingyourapikey')
get_apikey
: Gets the currently set API key. Default is demo
settings.get_apikey()
get_urlroot
: Gets the root API url for fmpcloud.io
settings.get_urlroot()
get_urlrootfmp
: Gets the root API url for fmpcloud's sister API financialmodelprep.com. Good to have but is not currently used in the wrapper.
settings.get_urlrootfmp()
from pyfmpcloud import company_valuation as cv
Here, you have functions that wrap around the APIs listed in fmpcloud.io (see fmpcloud.io API documentation) under Company Valuation. These are:
rss_feed
: This function returns updates for all filings of public companies, including their filing type (10-Q, 13-F, etc.), their CIK number, and the date and time of the filing.
cv.rss_feed()
balance_sheet
: This function returns the balance sheet information of the specified ticker. Inputs are: ticker
, period
('annual' or 'quarter') and ftype
('full' or 'growth')
Examples:
cv.balance_sheet(ticker = 'AAPL', period = 'annual', ftype = 'full')
cv.balance_sheet(ticker = 'AAPL', period = 'annual', ftype = 'growth')
cv.balance_sheet(ticker = 'AAPL', period = 'quarter', ftype = 'full')
cv.balance_sheet(ticker = 'AAPL', period = 'quarter', ftype = 'growth')
income_statement
: This function returns the income statement of the specified ticker. Inputs arguments are: ticker
, period
('annual' or 'quarter') and ftype
('full', 'growth')
Examples:
cv.balance_sheet(ticker = 'AAPL', period = 'annual', ftype = 'full')
cv.balance_sheet(ticker = 'AAPL', period = 'annual', ftype = 'growth')
cv.balance_sheet(ticker = 'AAPL', period = 'quarter', ftype = 'full')
cv.balance_sheet(ticker = 'AAPL', period = 'quarter', ftype = 'growth')
cash_flow_statement
: This function returns the cash-flow statement of the specified ticker. Inputs are Inputs are: ticker
, period
('annual' or 'quarter') and ftype
('full' or 'growth')
Examples:
cv.cash_flow_statement(ticker = 'AAPL', period = 'annual', ftype = 'full')
cv.cash_flow_statement(ticker = 'AAPL', period = 'annual', ftype = 'growth')
cv.cash_flow_statement(ticker = 'AAPL', period = 'quarter', ftype = 'full')
cv.cash_flow_statement(ticker = 'AAPL', period = 'quarter', ftype = 'growth')
financial_ratios
: This function returns the financial ratios of the specified ticker. ttm
can provide these ratios for the trailing twelve months. Inputs arguments are: ticker
, period
('annual' or 'quarter') and ttm
(True
or False
)
Examples:
cv.financial_ratios(ticker = 'AAPL', period = 'annual', ttm = True)
cv.financial_ratios(ticker = 'AAPL', period = 'annual', ttm = False)
cv.financial_ratios(ticker = 'AAPL', period = 'quarter', ttm = True)
cv.financial_ratios(ticker = 'AAPL', period = 'quarter', ttm = False)
key_metrics
: This function returns the key metrics of the specified ticker. Inputs are: ticker
and period
('annual' or 'quarter')
Examples:
cv.key_metrics(ticker = 'AAPL', period = 'annual')
cv.key_metrics(ticker = 'AAPL', period = 'quarter')
enterprise_value
: This function returns the enterprise value of the specified ticker. Inputs are: ticker
and period
('annual' or 'quarter')
Examples:
cv.enterprise_value(ticker = 'AAPL', period = 'annual')
cv.enterprise_value(ticker = 'AAPL', period = 'quarter')
financial_statements_growth
: This function returns the financial statements growth/evolution over time of the specified ticker. Inputs are: ticker
and period
('annual' or 'quarter')
Examples:
cv.financial_statements_growth(ticker = 'AAPL', period = 'annual')
cv.financial_statements_growth(ticker = 'AAPL', period = 'quarter')
dcf
: This function returns the discounted cashflow value of the specified ticker, over time. Inputs are: ticker
and history
('today', 'daily', 'annual' or 'quarter')
Examples:
cv.dcf(ticker = 'AAPL', history = 'today')
cv.dcf(ticker = 'AAPL', history = 'daily')
cv.dcf(ticker = 'AAPL', history = 'annual')
cv.dcf(ticker = 'AAPL', history = 'quarter')
market_capitalization
: This function returns the market capitalization of the specified ticker, over time. Inputs are: ticker
and history
('today', 'daily')
Examples:
cv.market_capitalization(ticker = 'AAPL', history = 'today')
cv.market_capitalization(ticker = 'AAPL', history = 'daily')
rating
: This function returns the ratings of the specified ticker, over time. Inputs are: ticker
and history
('today', 'daily')
Examples:
cv.rating(ticker = 'AAPL', history = 'today')
cv.rating(ticker = 'AAPL', history = 'daily')
stock_screener
: A function to screen stocks based on market capitalization, beta, dividend payouts, trading volume and sector. Also allows you to limit the number of rows returned from the request. The following are the arguments to apply the filter.
mcgt
: float
input for market cap greater than.
mclt
: float
input for market cap less than
bgt
: float
input for beta greater than
blt
: float
input for beta less than
divgt
: float
input for dividend greater than
divlt
: float
input for dividend less than
volgt
: float
input for volume greater than
vollt
: float
input for volume less than
sector
: string
input for sector.
limit
: int
input for limit of returned rows from the API request
Examples:
cv.stock_screener(mcgt = 1000000, mclt = 5000000, bgt = 1.2, blt = 2.1)
cv.stock_screener(mcgt = 1000000, mclt = 5000000, volgt = 500000, blt = 2.1, divgt = 1)
from pyfmpcloud import stock_time_series as sts
These contain functions from fmpcloud.io 's API for Stock Time Series (see fmpcloud.io API documentation) under Stock Time Series. these are:
real_time_quote
: Real-Time Quotes for specified tickers. Input is ticker
Example:
sts.real_time_quote('AAPL')
ticker_search
: Partial matching of tickers based on provided string element. Inputs are match
, limit
and exchange
Examples:
sts.ticker_search(match = 'AA', limit = 100, exchange = 'Nasdaq')
historical_stock_data
: Historical stock data for specified tickers based on specified period
('1 min', '5min', '15min', '30min' and '1hour', or daily change type dailytype
('line', 'change'). period
and dailytype
cannot be used together. If dailytype
is used, you can specify start
and end
dates for your request. Dates are a simple string (not a datetime object) in the format yyyy-mm-dd. Alternatively, you can also specify a request for daily prices over the last number of days using last
Examples:
sts.historical_stock_data('AAPL', period = '1hour')
sts.historical_stock_data('AAPL', dailytype = 'line', start = '2019-04-15', end = '2020-04-15')
sts.historical_stock_data('AAPL', dailytype = 'change', start = '2019-04-15', end = '2020-04-15')
sts.historical_stock_data('AAPL', dailytype = 'change', last = 30)
batch_request_eod_prices
: batch request for EOD prices for a single date (if no date is specified, it will return the EOD prices for the last market close). Only accepts an array of strings, even for a single ticker
request. If tickers are provided, a date must be provided. If no ticker is provided, it will return the EOD prices for all tickers. date
is a simple string (not a datetime object) in the format yyyy-mm-dd
Examples:
sts.batch_request_eod_prices()
sts.batch_request_eod_prices(['AAPL'], date='2020-04-15')
sts.batch_request_eod_prices(date='2020-04-15')
sts.batch_request_eod_prices(['AAPL', 'FB', 'MSFT'], date='2020-04-15')
symbol_list
: List of all available tickers.
Example:
sts.symbol_list()
company_profile
: Company profile for the specified ticker
Example:
sts.company_profile('AAPL')
available_markets_and_tickers
: List of available stocks on the specified market (e.g. Nasdaq), and prices of the tickers on the specified market. Inputs are markettype
('ETF','Commodities','Euronext','NYSE','AMEX','TSX','Mutual Funds','Index' or 'Nasdaq') and a boolean marketprices` (
True or False
) to indicate if prices of the tickers for the specified markettypes
are sought.
Examples:
sts.available_markets_and_tickers(markettype = 'Nasdaq')
sts.available_markets_and_tickers(markettype = 'Nasdaq', marketprices = True)
stock_market_performances
: Overview of the market performance across specified performance type, such as by sector, or by largest gainers. Input is performancetype
('active','gainers','losers','sector','sector historical', 'market hours')
Examples:
sts.stock_market_performances(performancetype = 'active')
sts.stock_market_performances(performancetype = 'market hours')
from pyfmpcloud import forex as fx
These contain functions from fmpcloud.io 's API for Forex (see fmpcloud.io API documentation). these are:
forex_realtime_quote
: Real-time quotes for specified fx tickers. You can request the list of forex, their prices, or both through the fxtype
('list', 'price', 'both') argument.
Examples:
fx.forex_realtime_quote(fxtype = 'list')
fx.forex_realtime_quote(fxtype = 'price')
fx.forex_realtime_quote(fxtype = 'both')
forex_historical_data
: Historical fx data for specified tickers based on specified period
('1 min', '5min', '15min', '30min' and '1hour', or daily change type dailytype
('line', 'change'). period
and dailytype
cannot be used together. If dailytype
is used, you can specify start
and end
dates for your request. Dates are a simple string (not a datetime object) in the format yyyy-mm-dd. Alternatively, you can also specify a request for daily fx prices over the last number of days using last
Examples:
fx.forex_historical_data('EURUSD', period = '1hour')
fx.forex_historical_data('EURUSD', dailytype = 'line', start = '2019-04-15', end = '2020-04-15')
fx.forex_historical_data('EURUSD', dailytype = 'change', start = '2019-04-15', end = '2020-04-15')
fx.forex_historical_data('EURUSD', dailytype = 'change', last = 30)
from pyfmpcloud import crypto as cp
These contain functions from fmpcloud.io 's API for Crypto (see fmpcloud.io API documentation). These are:
crypto_realtime_quote
: Real-time quotes for specified crypto tickers. You can request the list of crypto or their prices through the cryptotype
('list', 'price') argument.
Examples:
cp.crypto_realtime_quote(cryptotype = 'list')
cp.crypto_realtime_quote(cryptotype = 'price')
crypto_historical_data
: Historical crypto data for specified tickers based on specified period
('1 min', '5min', '15min', '30min' and '1hour', or daily change type dailytype
('line', 'change'). period
and dailytype
cannot be used together. If dailytype
is used, you can specify start
and end
dates for your request. Dates are a simple string (not a datetime object) in the format yyyy-mm-dd. Alternatively, you can also specify a request for daily fx prices over the last number of days using last
Examples:
cp.crypto_historical_data('BTCUSD', period = '1hour')
cp.crypto_historical_data('BTCUSD', dailytype = 'line', start = '2019-04-15', end = '2020-04-15')
cp.crypto_historical_data('BTCUSD', dailytype = 'change', start = '2019-04-15', end = '2020-04-15')
cp.crypto_historical_data('BTCUSD', dailytype = 'change', last = 30)
from pyfmpcloud import form_13f as ff
These contain functions from fmpcloud.io 's API for Form 13f (see fmpcloud.io API documentation). These are:
form_list
: List of all 13F forms available on the API. See: https://fmpcloud.io/documentation#thirteenFormList
Examples:
ff.form_list()
form_nametocik
: Converts specified company
name to the corresponding CIK number. Allows partial matching. See: https://fmpcloud.io/documentation#thirteenFormName
Examples:
ff.form_nametocik('Berskshire')
form_ciktoname
: Converts the specified cik
number to corresponding company name. See: https://fmpcloud.io/documentation#thirteenFormCik
Examples:
ff.form_ciktoname('1214816')
form
: Returns all associated 13F forms for the specified cik
number, for specified year
including issuer name, SEC link, etc. See: https://fmpcloud.io/documentation#thirteenForm
Examples:
ff.form('49205', '2020')
cusip_mapper
: Returns company/security name for specified CUSIP number. https://fmpcloud.io/documentation#cusipMapper
Examples:
ff.cusip_mapper('000360206')
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.
FAQs
A python-based wrapper for the Financial Models Prep pro API (fmpcloud.io) for financial data of public companies
We found that pyfmpcloud demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.