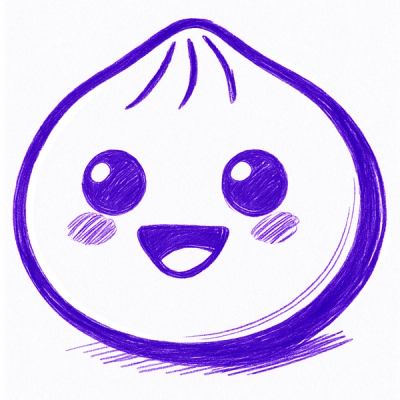
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
A simple interface for terminal based progress updates
Begin by creating an instance with the starting and ending value
import os
files = os.listdir('.')
bar = ProgressBar(0, len(files))
for file in files:
bar.iterbegin('Processing file: {}'.format(file))
process(file)
bar.iterdone()
bar.done()
Manually call ProgressBar.iterbegin([message])
before an iteration starts
and call ProgressBar.iterend()
when it completes or ProgressBar.iterfail()
if it fails
When all items complete use ProgressBar.done()
to print a summary
ProgressBar instances can also be used as context managers
use a with statement on every iteration
import os
files = os.listdir('.')
bar = ProgressBar(0, len(files), supress_exc=True)
for file in files:
with bar:
process(file)
bar.done()
Using the supress_exc=True
argument will report statistic for failures
from raised exceptions but not raise the exception itself
This is not necessary to use bar as a Context Manager but you must use it as a Context Manager to get the exception suppressing behavior
FAQs
A terminal progress printing utility
We found that pyprogress-elunico demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.