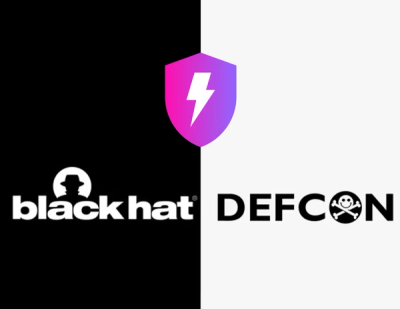
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
A Python package that seamlessly transfers a requests.Session
to a Selenium WebDriver, maintaining session cookies and proxy settings. Ideal for web scraping and automated browsing with pre-established session states.
requests.Session
to a Selenium WebDriver.requests.Session
.pip install reqdriver
Import the RequestsDriver
class from the reqdriver
package:
from reqdriver import RequestsDriver
import requests
Transfer cookies from a requests.Session
:
session = requests.Session()
session.cookies.set('test_cookie', '12345', domain='example.com')
req_driver = RequestsDriver(session)
req_driver.set_cookies('http://example.com')
driver = req_driver.get_driver()
driver.get('http://example.com')
Set up a WebDriver with the same proxy settings as your requests.Session
:
session = requests.Session()
session.proxies = {
'http': 'http://192.168.3.2:8080'
}
req_driver = RequestsDriver(session)
driver = req_driver.get_driver()
driver.get('http://example.com')
Pass your custom Chrome WebDriver options:
from selenium.webdriver.chrome.options import Options
custom_options = Options()
custom_options.add_argument('--headless')
session = requests.Session()
req_driver = RequestsDriver(session, custom_options=custom_options)
driver = req_driver.get_driver()
driver.get('http://example.com')
Pass your already created WebDriver instance:
from selenium import webdriver
existing_driver = webdriver.Chrome(executable_path='path_to_chromedriver')
session = requests.Session()
req_driver = RequestsDriver(session, driver=existing_driver)
req_driver.set_cookies('http://example.com')
existing_driver.get('http://example.com')
Contributions are welcome! Feel free to open issues or submit pull requests.
Distributed under the MIT License. See LICENSE
for more information.
FAQs
Effortlessly transfer sessions from Python requests to Selenium WebDriver.
We found that reqdriver demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.