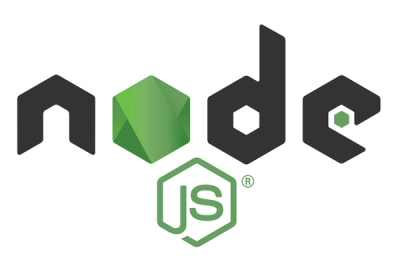
Security News
Node.js Homepage Adds Paid Support Link, Prompting Contributor Pushback
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Assertion library (skeleton) inspired from `assertpy` but without batteries
Assertion library (skeleton) inspired from assertpy but without batteries! also allows to convert all the assertions to warnings, just like delayed asserts.
assertpy
is beautiful library but for my needs it's too much, i really loved the API of
assertpy
so i created new module because of following reasons
please check tests/test_*.py
for use cases.
assert_warn
to be dynamic, i.e. given certain condition all assertions are converted to
warning.
ASSERT_ERROR_AS_WARNING
variable which can do that!assert_that
API to make is call based with assert_that(.., as_warn=True)
SimpleAssertions
class, and just inherit it to add new methodassert_that
in assertpy
creates new instance of class and loads all the extensions on every
call, and i wanted to avoid that!
SimpleAssertion
class, you can just make it part of your assertion classNote: earlier i used
assert_that
keyword fromassertpy
but i felt like it's copying from other library , so i changedassert_that
tocheck
, while reading this gives same amount of experience but saved 6 chars!
pip install simple-assertions
Please check tests
files for more usage here is only basic usage
Just import the check
function, and away you go...
from simple_assertions import check
def test_something():
check(4 + 10).is_equal_to(14)
check(1).is_instance_of(int)
check(3, "lucky_num", as_warn=True).is_equal_to(4)
from simple_assertions import SimpleAssertions
class YourTestClass:
def __init__(self):
self.check = SimpleAssertions().check
def test_something(self):
self.check(4 + 10).is_equal_to(14)
self.check(1).is_instance_of(int)
self.check(3, "lucky_num", as_warn=True).is_equal_to(4)
from simple_assertions import SimpleAssertions
from typing import Union
class YourAssertions(SimpleAssertions):
def __init__(self, as_warn=False, logger=None):
super().__init__(as_warn, logger)
def is_greater_than(self, other: Union[int, float]):
if self.val_to_chk.val < other:
self.raise_err(self.compare_err_msg(other, "to be greater than"))
return self
class YourTestClass:
def __init__(self):
self.check = YourAssertions().check
def test_something(self):
self.check(4 + 10).is_greater_than(10).is_equal_to(14)
self.check(1).is_instance_of(int)
self.check(3, "lucky_num", as_warn=True).is_equal_to(4)
Though only checked with unittests
but it should work fine with pytest or Nose.
FAQs
Assertion library (skeleton) inspired from `assertpy` but without batteries
We found that simple-assertions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.
Research
Security News
The Socket Research Team investigates a malicious Python typosquat of a popular password library that forces Windows shutdowns when input is incorrect.