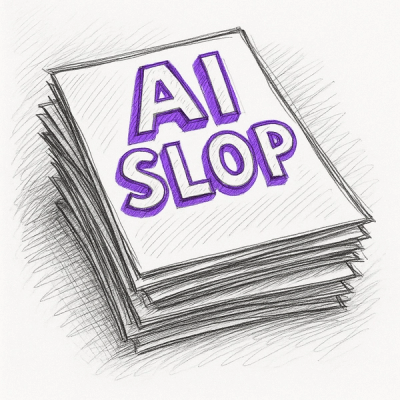
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
simple-query-builder
Advanced tools
This is a small easy-to-use component for working with a database. It provides some public methods to compose SQL queries and manipulate data. Each SQL query is prepared and safe.
This is a small easy-to-use module for working with a database. It provides some public methods to compose SQL queries and manipulate data. Each SQL query is prepared and safe. QueryBuilder fetches data to dictionary by default. At present time the component supports SQLite (file or memory).
Bug reports and/or pull requests are welcome
The module is available as open source under the terms of the MIT license
Install the current version with PyPI:
pip install simple-query-builder
Or from Github:
pip install https://github.com/co0lc0der/simple-query-builder-python/archive/main.zip
QueryBuilder
with Database()
from simple_query_builder import *
qb = QueryBuilder(DataBase(), 'my_db.db')
# or DB in memory
qb = QueryBuilder(DataBase(), ':memory:')
results = qb.select('users').all()
Result query
SELECT * FROM `users`;
results = qb.select('users').where([['id', '>', 1], 'and', ['group_id', '=', 2]]).all()
Result query
SELECT * FROM `users` WHERE (`id` > 1) AND (`group_id` = 2);
qb.update('users', {
'username': 'John Doe',
'status': 'new status'
})\
.where([['id', '=', 7]])\
.limit()\
.go()
Result query
UPDATE `users` SET `username` = 'John Doe', `status` = 'new status'
WHERE `id` = 7 LIMIT 1;
More examples you can find in documentation
I'm going to add the next features into future versions
BETWEEN
WHERE EXISTS
CREATE TABLE
, move qb.drop()
and qb.truncate()
into it)WITH
FAQs
This is a small easy-to-use component for working with a database. It provides some public methods to compose SQL queries and manipulate data. Each SQL query is prepared and safe.
We found that simple-query-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.