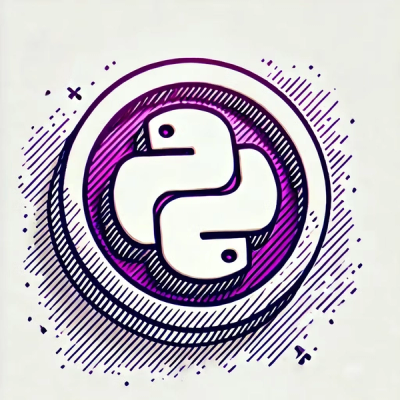
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Travel Time Python SDK helps users find locations by journey
time rather than using ‘as the crow flies’ distance.
Time-based searching gives users more opportunities for personalisation and delivers a more relevant search.
Install Travel Time Python SDK in a virtualenv
using pip
. virtualenv
is a tool to create isolated Python
environments.
virtualenv
allows to install Travel Time Python SDK without needing system install permissions, and without clashing
with the installed system dependencies.
pip3 install virtualenv
virtualenv <your-env>
source <your-env>/bin/activate
<your-env>/bin/pip install traveltimepy
pip install virtualenv
virtualenv <your-env>
<your-env>\Scripts\activate
<your-env>\Scripts\pip.exe install traveltimepy
In order to authenticate with Travel Time API, you will have to supply the Application Id and Api Key.
from traveltimepy import TravelTimeSdk
sdk = TravelTimeSdk(app_id="YOUR_APP_ID", api_key="YOUR_APP_KEY")
Given origin coordinates, find shapes of zones reachable within corresponding travel time.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
print(results)
asyncio.run(main())
import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_geojson_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
print(results)
asyncio.run(main())
import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
response = await sdk.time_map_wkt_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
response.pretty_print() # for a custom formatted response
print(response) # default Python print
asyncio.run(main())
import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
response = await sdk.time_map_wkt_no_holes_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
response.pretty_print() # for a custom formatted response
print(response) # default Python print
asyncio.run(main())
A very fast version of time_map()
. However, the request parameters are much more limited.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.requests.time_map_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_fast_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
transportation=Transportation(type="driving+ferry"),
travel_time=900
)
print(results)
asyncio.run(main())
import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.requests.time_map_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_fast_geojson_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
transportation=Transportation(type="driving+ferry"),
travel_time=900
)
print(results)
asyncio.run(main())
import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.requests.time_map_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_fast_wkt_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
transportation=Transportation(type="driving+ferry"),
travel_time=900
)
print(results)
asyncio.run(main())
import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.requests.time_map_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_fast_wkt_no_holes_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
transportation=Transportation(type="driving+ferry"),
travel_time=900
)
print(results)
asyncio.run(main())
Calculate the travel times to all H3 cells within a travel time catchment area. Return the max, min, and mean travel time for each cell.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, H3Centroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.h3_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
H3Centroid(h3_centroid="87195da49ffffff"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=8,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
A very fast version of H3. However, the request parameters are more limited.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, H3Centroid
from traveltimepy.dto.requests.time_filter_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.h3_fast_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
H3Centroid(h3_centroid="87195da49ffffff"),
],
properties=[CellProperty.MIN],
resolution = 8,
transportation=Transportation(type="driving+ferry"),
travel_time=900,
)
print(results)
asyncio.run(main())
Calculate the travel times to all geohash cells within a travel time catchment area. Return the max, min, and mean travel time for each cell.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, GeohashCentroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geohash_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
GeohashCentroid(geohash_centroid="gcpvj3"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=6,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
A very fast version of Geohash. However, the request parameters are more limited.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, GeohashCentroid
from traveltimepy.dto.requests.time_filter_fast import Transportation
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geohash_fast_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
GeohashCentroid(geohash_centroid="gcpvj3"),
],
properties=[CellProperty.MIN],
resolution = 6,
transportation=Transportation(type="driving+ferry"),
travel_time=900,
)
print(results)
asyncio.run(main())
Given origin coordinates, find intersections of specified shapes or cells.
Currently these requests support Intersections in this SDK:
Intersection requests take the same params as their regular (arrival_search
/ departure_search
) counterparts. Coordinates list size cannot be more than 10.
Intersection requests return the same responses as their regular (arrival_search
/ departure_search
) counterparts.
Time Map:
import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_intersection_async( # `sdk.intersection_async` will work too
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
print(results)
asyncio.run(main())
H3:
import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, H3Centroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.h3_intersection_async_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
H3Centroid(h3_centroid="87195da49ffffff"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=8,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
Geohash:
import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, GeohashCentroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geohash_intersection_async_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
GeohashCentroid(geohash_centroid="gcpvj3"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=6,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
Given origin coordinates, find unions of specified shapes or cells.
Currently these requests support Intersections in this SDK:
Union requests take the same params as their regular (arrival_search
/ departure_search
) counterparts. Coordinates list size cannot be more than 10.
Union requests return the same responses as their regular (arrival_search
/ departure_search
) counterparts.
Time Map:
import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.time_map_union_async( # `sdk.union_async` will work too
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
print(results)
asyncio.run(main())
H3:
import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, H3Centroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.h3_union_async_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
H3Centroid(h3_centroid="87195da49ffffff"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=8,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
Geohash:
import asyncio
from datetime import datetime
from traveltimepy import Coordinates, TravelTimeSdk
from traveltimepy.dto.common import CellProperty, GeohashCentroid
from traveltimepy.dto.transportation import Driving
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geohash_union_async_async(
coordinates=[
Coordinates(lat=51.507609, lng=-0.128315),
GeohashCentroid(geohash_centroid="gcpvj3"),
],
arrival_time=datetime.now(),
transportation=Driving(),
travel_time=900,
resolution=6,
properties=[CellProperty.MIN],
)
print(results)
asyncio.run(main())
Given origin coordinates, find shapes of zones reachable within corresponding travel distance.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Driving, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.distance_map_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315), Coordinates(lat=51.517609, lng=-0.138315)],
arrival_time=datetime.now(),
transportation=Driving()
)
print(results)
asyncio.run(main())
Given origin and destination points filter out points that cannot be reached within specified time limit. Find out travel times, distances and costs between an origin and up to 2,000 destination points.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Location, Coordinates, PublicTransport, Property, FullRange, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
locations = [
Location(id="London center", coords=Coordinates(lat=51.508930, lng=-0.131387)),
Location(id="Hyde Park", coords=Coordinates(lat=51.508824, lng=-0.167093)),
Location(id="ZSL London Zoo", coords=Coordinates(lat=51.536067, lng=-0.153596))
]
results = await sdk.time_filter_async(
locations=locations,
search_ids={
"London center": ["Hyde Park", "ZSL London Zoo"],
"ZSL London Zoo": ["Hyde Park", "London center"],
},
departure_time=datetime.now(),
travel_time=3600,
transportation=PublicTransport(type="bus"),
properties=[Property.TRAVEL_TIME],
range=FullRange(enabled=True, max_results=3, width=600)
)
print(results)
asyncio.run(main())
A very fast version of time_filter()
. However, the request parameters are much more limited.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import Location, Coordinates, Transportation, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
locations = [
Location(id="London center", coords=Coordinates(lat=51.508930, lng=-0.131387)),
Location(id="Hyde Park", coords=Coordinates(lat=51.508824, lng=-0.167093)),
Location(id="ZSL London Zoo", coords=Coordinates(lat=51.536067, lng=-0.153596))
]
results = await sdk.time_filter_fast_async(
locations=locations,
search_ids={
"London center": ["Hyde Park", "ZSL London Zoo"],
"ZSL London Zoo": ["Hyde Park", "London center"],
},
transportation=Transportation(type="public_transport"),
one_to_many=False
)
print(results)
asyncio.run(main())
A fast version of time filter communicating using protocol buffers.
The request parameters are much more limited and only travel time is returned. In addition, the results are only approximately correct (95% of the results are guaranteed to be within 5% of the routes returned by regular time filter). This inflexibility comes with a benefit of faster response times (Over 5x faster compared to regular time filter) and larger limits on the amount of destination points.
import asyncio
from traveltimepy import ProtoCountry, Coordinates, ProtoTransportation, TravelTimeSdk, PropertyProto
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
travel_times = await sdk.time_filter_proto_async(
origin=Coordinates(lat=51.425709, lng=-0.122061),
destinations=[
Coordinates(lat=51.348605, lng=-0.314783),
Coordinates(lat=51.337205, lng=-0.315793)
],
transportation=ProtoTransportation.DRIVING_FERRY,
travel_time=7200,
country=ProtoCountry.UNITED_KINGDOM,
properties=[PropertyProto.DISTANCE],
)
print(travel_times)
asyncio.run(main())
Returns routing information between source and destinations.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Location, Coordinates, PublicTransport, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
locations = [
Location(id="London center", coords=Coordinates(lat=51.508930, lng=-0.131387)),
Location(id="Hyde Park", coords=Coordinates(lat=51.508824, lng=-0.167093)),
Location(id="ZSL London Zoo", coords=Coordinates(lat=51.536067, lng=-0.153596))
]
results = await sdk.routes_async(
locations=locations,
search_ids={
"London center": ["Hyde Park", "ZSL London Zoo"],
"ZSL London Zoo": ["Hyde Park", "London center"],
},
transportation=PublicTransport(),
departure_time=datetime.now()
)
print(results)
asyncio.run(main())
Match a query string to geographic coordinates.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geocoding_async(query="Parliament square", limit=30)
print(results.features)
asyncio.run(main())
Match a latitude, longitude pair to an address.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.geocoding_reverse_async(lat=51.507281, lng=-0.132120)
print(results.features)
asyncio.run(main())
Find reachable postcodes from origin (or to destination) and get statistics about such postcodes. Currently only supports United Kingdom.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Coordinates, PublicTransport, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.postcodes_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315)],
departure_time=datetime.now(),
transportation=PublicTransport()
)
print(results)
asyncio.run(main())
Find districts that have a certain coverage from origin (or to destination) and get statistics about postcodes within such districts. Currently only supports United Kingdom.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Coordinates, PublicTransport, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.postcodes_districts_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315)],
departure_time=datetime.now(),
transportation=PublicTransport()
)
print(results)
asyncio.run(main())
Find sectors that have a certain coverage from origin (or to destination) and get statistics about postcodes within such sectors. Currently only supports United Kingdom.
f"/v4/{v4_endpoint_path}"
.import asyncio
from datetime import datetime
from traveltimepy import Coordinates, PublicTransport, TravelTimeSdk, ZonesProperty
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.postcodes_sectors_async(
coordinates=[Coordinates(lat=51.507609, lng=-0.128315)],
departure_time=datetime.now(),
transportation=PublicTransport(),
properties=[ZonesProperty.TRAVEL_TIME_REACHABLE, ZonesProperty.TRAVEL_TIME_ALL]
)
print(results)
asyncio.run(main())
Returns information about currently supported countries.
It is useful when you have an application that can do searches in any country that we support, you can use Supported Locations to get the map name for a certain point and then use this endpoint to check what features are available for that map. That way you could show fares for routes in the maps that support it.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
results = await sdk.map_info_async()
print(results)
asyncio.run(main())
Find out what points are supported by our api. The returned map name for a point can be used to determine what features are supported.
f"/v4/{v4_endpoint_path}"
.import asyncio
from traveltimepy import Location, Coordinates, TravelTimeSdk
async def main():
sdk = TravelTimeSdk("YOUR_APP_ID", "YOUR_APP_KEY")
locations = [
Location(id="Kaunas", coords=Coordinates(lat=54.900008, lng=23.957734)),
Location(id="London", coords=Coordinates(lat=51.506756, lng=-0.12805)),
Location(id="Bangkok", coords=Coordinates(lat=13.761866, lng=100.544818)),
Location(id="Lisbon", coords=Coordinates(lat=38.721869, lng=-9.138549)),
]
results = await sdk.supported_locations_async(locations)
print(results.locations)
print(results.unsupported_locations)
asyncio.run(main())
In transportation.py you can find all implemented transportation types, their sub-parameters and their default values.
These examples don't apply to proto / fast endpoints. For more examples you can always refer to Unit Tests
from traveltimepy import Driving, DrivingTrafficModel
transportation=Driving()
transportation=Driving(disable_border_crossing = True)
transportation=Driving(traffic_model = DrivingTrafficModel.OPTIMISTIC)
from traveltimepy import Walking
transportation=Walking()
from traveltimepy import Cycling
transportation=Cycling()
from traveltimepy import Ferry, DrivingTrafficModel
transportation=Ferry()
transportation=Ferry(type="cycling+ferry")
transportation=Ferry(type="driving+ferry")
transportation=Ferry(type="cycling+ferry", boarding_time = 300)
transportation=Ferry(type="driving+ferry", traffic_model=DrivingTrafficModel.OPTIMISTIC)
from traveltimepy import DrivingTrain, MaxChanges, DrivingTrafficModel
transportation=DrivingTrain()
transportation=DrivingTrain(
pt_change_delay = 300,
driving_time_to_station=1800,
parking_time=800,
walking_time=500,
max_changes=MaxChanges(enabled=True, limit=3),
traffic_model=DrivingTrafficModel.OPTIMISTIC
)
from traveltimepy import PublicTransport, MaxChanges
transportation=PublicTransport() # type="public_transport" - any public transport
transportation=PublicTransport(type="train")
transportation=PublicTransport(type="bus")
transportation=PublicTransport(type="coach")
transportation=PublicTransport(
pt_change_delay = 300,
walking_time=500,
max_changes=MaxChanges(enabled=True, limit=3)
)
from traveltimepy import CyclingPublicTransport, MaxChanges
transportation=CyclingPublicTransport()
transportation=CyclingPublicTransport(
walking_time=500,
pt_change_delay = 300,
cycling_time_to_station=300,
parking_time=800,
boarding_time=300,
max_changes=MaxChanges(enabled=True, limit=3)
)
When picking transportation mode for proto requests take note that some of the transportation modes support extra configuration parameters.
Examples:
Select transportation mode, no extra parameters
from traveltimepy.dto.requests.time_filter_proto import ProtoTransportation
transportation=ProtoTransportation.PUBLIC_TRANSPORT
transportation=ProtoTransportation.DRIVING
transportation=ProtoTransportation.DRIVING_AND_PUBLIC_TRANSPORT
transportation=ProtoTransportation.DRIVING_FERRY
transportation=ProtoTransportation.WALKING
transportation=ProtoTransportation.CYCLING
transportation=ProtoTransportation.CYCLING_FERRY
transportation=ProtoTransportation.WALKING_FERRY
This mode uses ProtoTransportation.PUBLIC_TRANSPORT
transportion mode and allows to set these parameters:
walking_time_to_station
- limits the possible duration of walking paths.
This limit is of low precedence and will not override the global travel time limit
Optional. Must be <= 1800.from traveltimepy.dto.requests.time_filter_proto import PublicTransportWithDetails
transportation=PublicTransportWithDetails()
transportation=PublicTransportWithDetails(walking_time_to_station=900)
This mode uses ProtoTransportation.DRIVING_AND_PUBLIC_TRANSPORT
transportion mode and allows to set these parameters:
walking_time_to_station
- limits the possible duration of walking paths.
This limit is of low precedence and will not override the global travel time limit.
Optional. Must be <= 1800.driving_time_to_station
- limits the possible duration of driving paths.
This limit is of low precedence and will not override the global travel time limit
Optional. Must be <= 1800.parking_time
- constant penalty to apply to simulate the difficulty of finding a parking spot.
Optional. Cannot be greater than the global travel time limit.from traveltimepy.dto.requests.time_filter_proto import DrivingAndPublicTransportWithDetails
transportation=DrivingAndPublicTransportWithDetails()
transportation=DrivingAndPublicTransportWithDetails(walking_time_to_station=900, driving_time_to_station=1800, parking_time=300)
level_of_detail
can be used to specify how detailed the isochrone result should be.
For a more detailed description of how to use this parameter, you can refer to our API Docs
from traveltimepy import LevelOfDetail
# scale_type "simple"
level_of_detail=LevelOfDetail(scale_type="simple", level="lowest")
# scale_type "simple_numeric"
level_of_detail=LevelOfDetail(scale_type="simple_numeric", level=0)
# scale_type "coarse_grid"
level_of_detail=LevelOfDetail(scale_type="coarse_grid", square_size=600)
snapping
Adjusts the process of looking up the nearest roads from the departure / arrival points.
For a more detailed description of how to use this parameter, you can refer to our API Docs
from traveltimepy.dto.common import Snapping, SnappingAcceptRoads, SnappingPenalty
snapping=Snapping(
penalty=SnappingPenalty.ENABLED, # default
accept_roads=SnappingAcceptRoads.BOTH_DRIVABLE_AND_WALKABLE # default
)
snapping=Snapping(
penalty=SnappingPenalty.DISABLED,
accept_roads=SnappingAcceptRoads.ANY_DRIVABLE
)
Cell properties are the properties
param used in H3 and geohash requests. Specifies proprties to be returned for each cell. Possible values: min, max, mean.
from traveltimepy.dto.common import CellProperty
# all properties
properties=[CellProperty.MIN, CellProperty.MAX, CellProperty.MEAN]
# some properties
properties=[CellProperty.MIN]
polygons_filter
specifies polygon filter of a single shape.
For a more detailed description of how to use this parameter, you can refer to our API Docs
from traveltimepy.dto.common import PolygonsFilter
polygons_filter = PolygonsFilter(limit=1)
polygons_filter = PolygonsFilter(limit=5)
render_mode
specifies how the shape should be rendered.
For a more detailed description of how to use this parameter, you can refer to our API Docs
from traveltimepy.dto.common import RenderMode
render_mode = RenderMode.APPROXIMATE_TIME_FILTER # default
render_mode = RenderMode.ROAD_BUFFERING
FAQs
Python Interface to Travel Time.
We found that traveltimepy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.