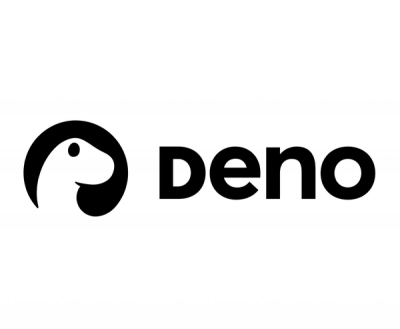
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
git.fractalqb.de/fractalqb/daq
Access nested properties in Go data structures.
Go code for working with dynamic data structures can sometimes become somewhat verbose. E.g. like this:
var data any
json.Unmarshal([]byte(`{
"glossary": {
"title": "example glossary",
"GlossDiv": {
"title": "S",
"GlossList": {
"GlossEntry": {
"ID": "SGML",
"GlossTerm": "Standard Generalized Markup Language"
}
}
}
}
}`), &data)
title := "-no title-"
jObj, ok := data.(map[string]any)
if !ok {
fmt.Println(title)
return
}
if data = jObj["glossary"]; data == nil {
fmt.Println(title)
return
}
if jObj, ok = data.(map[string]any); !ok {
fmt.Println(title)
return
}
if data = jObj["title"]; data == nil {
fmt.Println(title)
return
}
title, _ = data.(string)
fmt.Println(title)
// Output:
// example glossary
Package daq helps for example to make it much more concise:
var data any
json.Unmarshal([]byte(`{
"glossary": {
"title": "example glossary",
"GlossDiv": {
"title": "S",
"GlossList": {
"GlossEntry": {
"ID": "SGML",
"GlossTerm": "Standard Generalized Markup Language"
}
}
}
}
}`), &data)
title := StringOr("-no title-")(Get(data, "glossary", "title"))
fmt.Println(title)
// Output:
// example glossary
In addition, daq can do even more, e.g. evaluate static data structures with the same API, iterate and … please consult the reference.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.