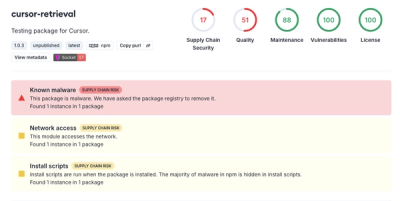
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
github.com/H0llyW00dzZ/FiberValidator
This is a custom validator middleware for the Fiber web framework. It provides a flexible and extensible way to define and apply validation rules to incoming request bodies. The middleware allows for easy validation and sanitization of data, enforcement of specific field requirements, and ensures the integrity of the application's input.
The middleware currently supports the following features:
More features and validation capabilities will be added in the future to enhance the middleware's functionality and cater to a wider range of validation scenarios.
To use this middleware in a Fiber project, Go must be installed and set up.
go get github.com/H0llyW00dzZ/FiberValidator
import "github.com/H0llyW00dzZ/FiberValidator"
To use the validator middleware in a Fiber application, create a new instance of the middleware with the desired configuration and register it with the application.
package main
import (
"github.com/gofiber/fiber/v2"
"github.com/H0llyW00dzZ/FiberValidator"
)
func main() {
app := fiber.New()
app.Use(validator.New(validator.Config{
Rules: []validator.Restrictor{
validator.RestrictUnicode{
Fields: []string{"name", "email"},
},
},
}))
// Register routes and start the server
// ...
app.Listen(":3000")
}
In the example above, a new instance of the validator middleware is created with a configuration that restricts the use of Unicode characters in the "name" and "email" fields of the request body.
The validator middleware accepts a validator.Config
struct for configuration. The available options are:
Rules
: A slice of validator.Restrictor
implementations that define the validation rules to be applied.Next
: An optional function that determines whether to skip the validation middleware for a given request. If the function returns true
, the middleware will be skipped.ErrorHandler
: An optional custom error handler function that handles the error response. If not provided, the default error handler will be used.To define custom validation rules, implement the validator.Restrictor
interface:
type Restrictor interface {
Restrict(c *fiber.Ctx) error
}
The Restrict
method takes a Fiber context and returns an error if the validation fails.
Here's an example implementation that restricts the use of Unicode characters in specified fields:
type RestrictUnicode struct {
Fields []string
}
func (r RestrictUnicode) Restrict(c *fiber.Ctx) error {
// Parse the request body based on the Content-Type header
// ...
// Check each configured field for Unicode characters
// ...
return nil
}
The validator middleware provides a default error handler that formats the error response based on the content type of the request. It supports JSON, XML, and plain text formats.
{"error": "Error message"}
.<xmlError><error>Error message</error></xmlError>
.You can customize the error handling behavior by providing a custom error handler function in the ErrorHandler
field of the validator.Config
struct. The custom error handler should have the following signature:
func(c *fiber.Ctx, err error) error
Example custom error handler:
func customErrorHandler(c *fiber.Ctx, err error) error {
if e, ok := err.(*validator.Error); ok {
return c.Status(fiber.StatusUnprocessableEntity).JSON(fiber.Map{
"custom_error": e.Message,
})
}
return err
}
In this example, the custom error handler checks if the error is of type *validator.Error
and returns a JSON response with a custom error format.
Contributions are welcome! If there are any issues or suggestions for improvements, please open an issue or submit a pull request.
This project is licensed under the BSD License. See the LICENSE file for details.
goos: windows
goarch: amd64
pkg: github.com/H0llyW00dzZ/FiberValidator
cpu: AMD Ryzen 9 3900X 12-Core Processor
BenchmarkValidatorWithSonicJSON/Valid_JSON_request-24 45967 24768 ns/op 16464 B/op 86 allocs/op
BenchmarkValidatorWithStandardJSON/Valid_JSON_request-24 43248 27835 ns/op 16624 B/op 112 allocs/op
BenchmarkValidatorWithDefaultXML/Valid_XML_request-24 28101 42913 ns/op 23223 B/op 212 allocs/op
BenchmarkValidatorWithCustomXML/Valid_XML_request-24 28191 43596 ns/op 23248 B/op 212 allocs/op
goos: windows
goarch: amd64
pkg: github.com/H0llyW00dzZ/FiberValidator
cpu: AMD Ryzen 9 3900X 12-Core Processor
BenchmarkValidatorWithSonicJSONSeafood/Valid_JSON_request-24 46785 24696 ns/op 16447 B/op 86 allocs/op
BenchmarkValidatorWithStandardJSONSeafood/Valid_JSON_request-24 42541 28542 ns/op 16672 B/op 112 allocs/op
BenchmarkValidatorWithDefaultXMLSeafood/Valid_XML_request-24 26637 44806 ns/op 23450 B/op 213 allocs/op
BenchmarkValidatorWithCustomXMLSeafood/Valid_XML_request-24 26622 45684 ns/op 23458 B/op 213 allocs/op
BenchmarkValidatorWithSonicJSON/Valid_JSON_request-24 50625 24377 ns/op 16410 B/op 86 allocs/op
BenchmarkValidatorWithStandardJSON/Valid_JSON_request-24 42150 27954 ns/op 16626 B/op 112 allocs/op
BenchmarkValidatorWithDefaultXML/Valid_XML_request-24 27764 43721 ns/op 23244 B/op 212 allocs/op
BenchmarkValidatorWithCustomXML/Valid_XML_request-24 27417 43951 ns/op 23256 B/op 212 allocs/op
goos: windows
goarch: amd64
pkg: github.com/H0llyW00dzZ/FiberValidator
cpu: AMD Ryzen 9 3900X 12-Core Processor
BenchmarkRestrictStringLengthLongDescriptionSonicJSON/Valid_JSON_long_description-24 55225 23263 ns/op 19344 B/op 58 allocs/op
BenchmarkRestrictStringLengthLongDescriptionStandardJSON/Valid_JSON_long_description-24 48288 24452 ns/op 19234 B/op 65 allocs/op
BenchmarkRestrictStringLengthLongDescriptionDefaultXML/Valid_XML_long_description-24 30114 39411 ns/op 25018 B/op 111 allocs/op
BenchmarkRestrictStringLengthLongDescriptionCustomXML/Valid_XML_long_description-24 30322 40180 ns/op 25025 B/op 111 allocs/op
BenchmarkValidatorWithSonicJSONSeafood/Valid_JSON_request-24 51158 23802 ns/op 16421 B/op 86 allocs/op
BenchmarkValidatorWithStandardJSONSeafood/Valid_JSON_request-24 43411 27269 ns/op 16652 B/op 112 allocs/op
BenchmarkValidatorWithDefaultXMLSeafood/Valid_XML_request-24 26984 45736 ns/op 23451 B/op 213 allocs/op
BenchmarkValidatorWithCustomXMLSeafood/Valid_XML_request-24 26899 45503 ns/op 23443 B/op 213 allocs/op
BenchmarkValidatorWithSonicJSON/Valid_JSON_request-24 50936 24697 ns/op 16417 B/op 86 allocs/op
BenchmarkValidatorWithStandardJSON/Valid_JSON_request-24 43172 28678 ns/op 16627 B/op 112 allocs/op
BenchmarkValidatorWithDefaultXML/Valid_XML_request-24 27283 43998 ns/op 23262 B/op 212 allocs/op
BenchmarkValidatorWithCustomXML/Valid_XML_request-24 27990 43546 ns/op 23264 B/op 212 allocs/op
goos: windows
goarch: amd64
pkg: github.com/H0llyW00dzZ/FiberValidator
cpu: AMD Ryzen 9 3900X 12-Core Processor
BenchmarkRestrictStringLengthLongDescriptionSonicJSON/Valid_JSON_long_description-24 51478 21306 ns/op 19331 B/op 58 allocs/op
BenchmarkRestrictStringLengthLongDescriptionStandardJSON/Valid_JSON_long_description-24 47730 25044 ns/op 19240 B/op 65 allocs/op
BenchmarkRestrictStringLengthLongDescriptionDefaultXML/Valid_XML_long_description-24 30450 39450 ns/op 25025 B/op 111 allocs/op
BenchmarkRestrictStringLengthLongDescriptionCustomXML/Valid_XML_long_description-24 29910 40017 ns/op 25025 B/op 111 allocs/op
BenchmarkValidatorWithSonicJSONSeafood/Valid_JSON_request-24 60594 20457 ns/op 14826 B/op 68 allocs/op
BenchmarkValidatorWithStandardJSONSeafood/Valid_JSON_request-24 50836 23481 ns/op 15081 B/op 94 allocs/op
BenchmarkValidatorWithDefaultXMLSeafood/Valid_XML_request-24 30085 40004 ns/op 21815 B/op 195 allocs/op
BenchmarkValidatorWithCustomXMLSeafood/Valid_XML_request-24 29608 40108 ns/op 21819 B/op 195 allocs/op
BenchmarkValidatorWithSonicJSON/Valid_JSON_request-24 61047 20826 ns/op 14801 B/op 68 allocs/op
BenchmarkValidatorWithStandardJSON/Valid_JSON_request-24 51811 23220 ns/op 15045 B/op 94 allocs/op
BenchmarkValidatorWithDefaultXML/Valid_XML_request-24 31850 38777 ns/op 21632 B/op 194 allocs/op
BenchmarkValidatorWithCustomXML/Valid_XML_request-24 30577 39133 ns/op 21633 B/op 194 allocs/op
[!NOTE] Based on the benchmark results, the following observations can be made:
- The Sonic JSON encoder/decoder consistently outperforms the standard JSON encoder/decoder in terms of execution time, bytes allocated, and allocations per operation.
- The custom XML encoder/decoder performs slightly slower than the default XML encoder/decoder in most cases, with similar memory usage.
- The JSON benchmarks generally have better performance compared to the XML benchmarks, with lower execution time, bytes allocated, and allocations per operation.
![]()
Overall, the benchmarks using the Sonic JSON encoder/decoder demonstrate the best performance among the tested scenarios.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.