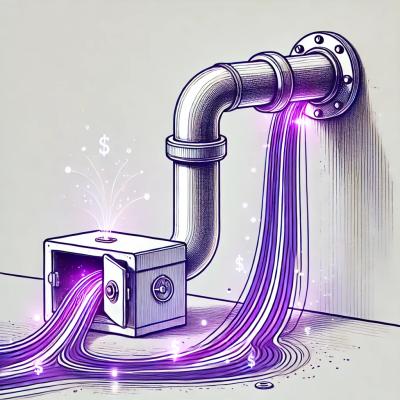
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
github.com/HuKeping/rbtree
This is an implementation of Red-Black tree written by Golang which does not support duplicate keys
.
With a healthy Go language installed, simply run go get github.com/HuKeping/rbtree
All you have to do is to implement a comparison function Less() bool
for your Item
which will be store in the Red-Black tree, here are some examples.
int
items.package main
import (
"fmt"
"github.com/HuKeping/rbtree"
)
func main() {
rbt := rbtree.New()
m := 0
n := 10
for m < n {
rbt.Insert(rbtree.Int(m))
m++
}
m = 0
for m < n {
if m%2 == 0 {
rbt.Delete(rbtree.Int(m))
}
m++
}
// 1, 3, 5, 7, 9 were expected.
rbt.Ascend(rbt.Min(), Print)
}
func Print(item rbtree.Item) bool {
i, ok := item.(rbtree.Int)
if !ok {
return false
}
fmt.Println(i)
return true
}
string
items.package main
import (
"fmt"
"github.com/HuKeping/rbtree"
)
func main() {
rbt := rbtree.New()
rbt.Insert(rbtree.String("Hello"))
rbt.Insert(rbtree.String("World"))
rbt.Ascend(rbt.Min(), Print)
}
func Print(item rbtree.Item) bool {
i, ok := item.(rbtree.String)
if !ok {
return false
}
fmt.Println(i)
return true
}
struct
items.package main
import (
"fmt"
"github.com/HuKeping/rbtree"
"time"
)
type Var struct {
Expiry time.Time `json:"expiry,omitempty"`
ID string `json:"id",omitempty`
}
// We will order the node by `Time`
func (x Var) Less(than rbtree.Item) bool {
return x.Expiry.Before(than.(Var).Expiry)
}
func main() {
rbt := rbtree.New()
var1 := Var{
Expiry: time.Now().Add(time.Second * 10),
ID: "var1",
}
var2 := Var{
Expiry: time.Now().Add(time.Second * 20),
ID: "var2",
}
var3 := Var{
Expiry: var2.Expiry,
ID: "var2-dup",
}
var4 := Var{
Expiry: time.Now().Add(time.Second * 40),
ID: "var4",
}
var5 := Var{
Expiry: time.Now().Add(time.Second * 50),
ID: "var5",
}
rbt.Insert(var1)
rbt.Insert(var2)
rbt.Insert(var3)
rbt.Insert(var4)
rbt.Insert(var5)
tmp := Var{
Expiry: var4.Expiry,
ID: "This field is not the key factor",
}
// var4 and var5 were expected
rbt.Ascend(rbt.Get(tmp), Print)
}
func Print(item rbtree.Item) bool {
i, ok := item.(Var)
if !ok {
return false
}
fmt.Printf("%+v\n", i)
return true
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.