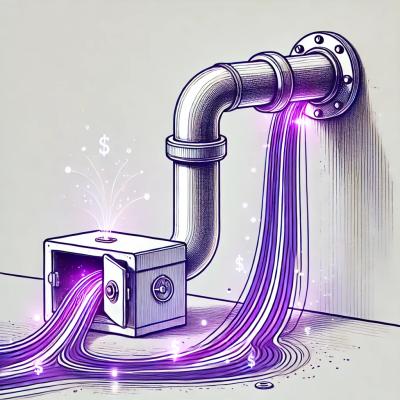
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
github.com/abhiaiyer91/apollo-storybook-decorator
Wrap your React Storybook stories with Apollo Client.
graphql-tools
http://dev.apollodata.com/tools/graphql-tools/mocking.htmlyarn add apollo-storybook-decorator -D
npm install apollo-storybook-decorator --save-dev
The Decorator:
type DecoratorType = {
//string representing your graphql schema, if you use tools like `babel-plugin-inline-import` you can import this from a .graphql file
typeDefs: string | Array<string>,
// object that resolves the keys of your graphql schema
mocks: Object,
// optional reducers to add other redux libraries or your own reducers
reducers?: Object,
// optional redux middlewares to be applied during creation of the redux store
reduxMiddlewares?: Array<Function> | ({ apolloClient }: MiddlewaresType) => Array<Function>,
// optional apollo client constructor options
apolloClientOptions?: Object,
// optional typeResolvers for complex mocking
typeResolvers?: Object,
// optional context
context?: Object,
// optional root value
rootValue?: Object,
}
You can add the decorator at a per story basis:
storiesOf('Apollo Client', module)
.addDecorator(
apolloStorybookDecorator({
typeDefs,
mocks,
}),
)
or you can add it to all stories, head to your storybook config.js
import { configure, addDecorator } from '@storybook/react';
import apolloStorybookDecorator from 'apollo-storybook-decorator';
import typeDefs from '../wherever/your/typeDefs/live';
import mocks from '../wherever/your/mocks/live';
addDecorator(
apolloStorybookDecorator({
typeDefs,
mocks
})
);
function loadStories() {
// stories...
}
configure(loadStories, module);
import React from 'react';
import { graphql } from 'react-apollo';
import gql from 'graphql-tag';
import { storiesOf } from '@storybook/react';
import apolloStorybookDecorator from 'apollo-storybook-decorator';
const typeDefs = `
type Query {
helloWorld: String
}
schema {
query: Query
}
`;
const mocks = {
Query: () => {
return {
helloWorld: () => {
return 'Hello from Apollo!!';
},
};
},
};
let HelloWorld = function HelloWorld({ data }) {
const hello = data && data.helloWorld;
if (data && data.loading) {
return <h1>Loading one second please!</h1>
}
return <h1>{hello}</h1>
};
HelloWorld = graphql(
gql`
query hello {
helloWorld
}
`,
)(HelloWorld);
storiesOf('Apollo Client', module)
.addDecorator(
apolloStorybookDecorator({
typeDefs,
mocks,
}),
)
.add('Hello World Test', () => {
return <HelloWorld />;
});
To get started, clone this repo and run the following command:
$ yarn # install node deps
To run the project's examples, run:
$ yarn storybook # for storybook testing
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.